Question
I NEED HELP on this C++ program. With the code that i have so far i am not able to output the same thing as
I NEED HELP on this C++ program. With the code that i have so far i am not able to output the same thing as the SAMPLE RUN provided below. Please tell me how to fix this. I belive I am doing something wrong in the calculations or when creating a random number because I get a negative number when i check the balance and also when i check the interest rates. PLEASE MAKE SURE THAT IT RUNS PROPERLY. thank you!
Grading rubric:
The program must separate Account class implementation from the main function file and, in addition, the Account class
must have its interface (Account.h) in a separate file from its implementation.
The code in the following files:
- AccountMain.cpp
- Account.cpp
- Account.h -- provided below.
Please use these file names exactly.
Summary
The lab requires you to create two files Account.cpp and AccountMain.cpp.
AccountClass Definition:
Class Account | |
Private Data Members: int accountId doublebalance doubleannualInterestRate | Account ID Current Balance of the account Current Annual Interest Rate of the Account (ex. 4.5) |
Public Member Functions: Account() Account(int id, double bal, double interest) | Constructors: Default Constructor(no parameters) Three-Parameter Constructor |
Public Member Functions: void setAccountId (int x) void setBalance(double x) void setInterest(double x) | Setters: Function sets id to x Function sets balance to x Function sets annualInterestRate to x |
Public Member Functions: int getAccountId() double getBalance() double getInterest() | Getters: Function returns accountId Function returns balance Function returns annualInterestRate |
Public Member Functions: | |
double getMonthlyInterestRate() | Function calculates the monthly interest rate and returns the value |
doublegetMonthlyInterest() | Function calculates the amount earned per month from interest and returns the value rounded to 2 decimal places. (Assume interest is not compounding) |
Bool withdraw(double amount) | Function only allows withdraw if the current balance is greater than or equal to the withdraw amount. Return true if withdrawal is successful, else return false. |
void deposit(double amount) | Function adds the amount passed as a parameter to the current balance. |
Write a program that creates an array of 10 Account objects.
When creating each Account object use the following guidelines:
Each objects accountId should be the index of its position within the array.
The balance of each Account object should be created from a random number generator functionreturning values between 10,000.00and 20,000.00. This return value should be rounded to two decimal places.
The interest rate of each Account object should be created from a random number generator function returning values between 1.5 and 5.0. This return value should be rounded to one decimal place.
The program should then ask the user to select an account number from 0 9 or -1 to exit the program.
If an account number is selected, then the user should be presented with some options. The five main bullets should form the menu presented. If a user makes any of the top five selections, then the menu should be represented. The menu should only not be represented with an entry of -1.
Enter 1 to make a deposit
o Ask the user for the amount they wish to deposit
Enter 2 to make a withdraw
o Ask the user for the amount they wish to withdraw
o Return if withdrawal was successful or unsuccessful depending on function return value
Enter 3 to check balance
o Display the accounts current balance
Enter 4 to check interest rate
o Display the accounts monthly and yearly interest rate
Enter 5 to display account summary
o Display account id, balance, monthly interest rate, and monthly interest amount
Enter -1 to return to the main menu
o This will return the user to the main menu prompting to select an account number
SAMPLE RUN HERE:
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Please enter an account number from 0-9: 3
Enter 1 to make a deposit
Enter 2 to make a withdraw
Enter 3 to check balance
Enter 4 to check interest rate
Enter 5 to display account summary
Enter -1 to return to the main menu
3
Current Balance: $10045.
Enter 1 to make a deposit
Enter 2 to make a withdraw
Enter 3 to check balance
Enter 4 to check interest rate
Enter 5 to display account summary
Enter -1 to return to the main menu
4
Monthly interest rate: 0.191667%
Yearly interest rate: 2.3%
Enter 1 to make a deposit
Enter 2 to make a withdraw
Enter 3 to check balance
Enter 4 to check interest rate
Enter 5 to display account summary
Enter -1 to return to the main menu
-1
Please enter an account number from 0-9:
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
MY C++ CODE:
LAB 6
AccountMain.cpp
#include "stdafx.h"
#include "Account.h"
#include
#include
#include
#include
#include
using namespace std;
int main()
{
//Returning Values
double maxBalance = 20000.00;
double minBalance = 10000.00;
double maxInterest = 5.0;
double minInterest = 1.5;
//Declaring arrays
Account accountlist[10];
for (int i = 0; i < 10; i++)
{
float r = (float)rand() / (float)RAND_MAX; //RAND = random value
double balance = minBalance + r*(maxBalance - minBalance);
double annualInterestRate = minInterest + r*(maxInterest - minInterest);
accountlist[i] = Account(i, balance, annualInterestRate);
int accountId;
cout << " Please enter an account number from 0-9: ";
cin >> accountId;
if (accountId < 0 || accountId > 9) {
exit(0);
}
else
{
do
{
int options;
double amount;
cout << " Enter 1 to make a deposit: " << endl;
cout << "Enter 2 to make a withdraw: " << endl;
cout << "Enter 3 to check balance: " << endl;
cout << "Enter 4 to check interest rate: " << endl;
cout << "Enter 5 to display account summary: " << endl;
cout << "Enter -1 to return to the main menu: " << endl;
cout << "Choice: ";
cin >> options;
switch (options)
{
case 1:
cout << " Enter amount to deposit: ";
cin >> amount;
accountlist[accountId].deposit(amount);
break;
case 2:
cout << " Enter amount to withdraw: ";
cin >> amount;
cout << accountlist[accountId].withdraw(amount);
break;
case 3:
cout << " Current Balance: " << "$" << accountlist[accountId].getBalance() << endl;
break;
case 4:
cout << " Monthly Interest Rate: " << accountlist[accountId].getMonthlyInterestRate() << "%" << endl;
cout << "Yearly Interest Rate: " << accountlist[accountId].getInterest() << "%" << endl;
break;
case 5:
cout << " \tAccount Id: " << accountId << endl;
cout << "Balance: " << accountlist[accountId].getBalance() << endl;
cout << "Monthly Interest Rate: " << accountlist[accountId].getMonthlyInterest() << endl;
cout << "Monthly Interest Amount:" << accountlist[accountId].getMonthlyInterestRate() << endl;
break;
default:
cout << " Please enter an account number from 0-9: " << endl;
cin >> accountId;
if (accountId < 0 || accountId > 9)
{
exit(0);
}
}
} while (true);
}
}
return 0;
}
Account.h
#pragma once
#include
using namespace std;
class Account
{
//Private Data Members
private:
int accountId;
double balance;
double annualInterestRate;
//Public Data Members
public:
Account(); //Constructor Function
Account(int id, double bal, double interest);
//Functions that sets to different values
void setAccountId(int x);
void setBalance(double x);
void setInterest(double x);
//Get Functions
int getAccountId();
double getBalance();
double getInterest();
//Functions that calculates
double getMonthlyInterestRate();
double getMonthlyInterest();
bool withdraw(double amount);
void deposit(double amount);
};
Account.cpp
//Libraries needed
#include
#include "Account.h"
#include "stdafx.h"
using namespace std;
//ALL FUNCITIONS GO HERE
//Constructor Function
Account::Account()
{
}
Account::Account(int id, double bal, double interest)
{
accountId = id;
balance = bal;
annualInterestRate = interest;
}
//Functions that sets to different values
void Account::setAccountId(int x)
{
int accountId = x;
}
void Account::setBalance(double x)
{
double balance = x;
}
void Account::setInterest(double x)
{
double annualInterestRate = x;
}
//Get Functions
int Account::getAccountId()
{
return accountId;
}
double Account::getBalance()
{
return balance;
}
double Account::getInterest()
{
return annualInterestRate;
}
//Funcions that calculates
double Account::getMonthlyInterestRate()
{
return annualInterestRate / 12;
}
double Account::getMonthlyInterest()
{
return (balance*(annualInterestRate / 12)) / 100;
}
bool Account::withdraw(double amount)
{
if (balance > amount)
{
balance = balance - amount;
return true;
}
else
{
return false;
}
}
void Account::deposit(double amount)
{
balance = balance + amount;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
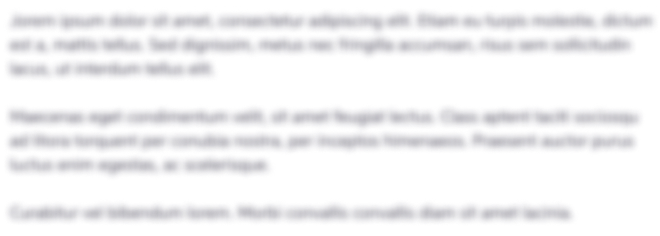
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started