Question
I need help passing a string that the user entered in Main in to a constructor from the class Message. In main (), you will
I need help passing a string that the user entered in Main in to a constructor from the class Message.
In main(), you will need to create object of the Message class. You will use this object to call the functions in the Message class that will encrypt, decrypt, save, and print the words retrieved from the document.
Before you create this Message object, you should prompt the user to enter an encryption/decryption key. The same key must be used for decryption as was used for encryption. Otherwise, the algorithms will not produce the correct results. Once the user enters the key, pass this string in to the constructor of the Message class when creating your Message object. This key is ultimately converted to upper case and stored in the "key" data member in the Vigenere class.
//Main:
/*
//Include statements #include
//Global Variables
//Function prototypes void displayMenu(); string strtok_s(); void wait();
//Display Menu Funciton void displayMenu() { //Variable declaration int choice{ 0 }; //Output the menu cout << "Vinegere Cypher" << endl; cout << "Main Menu" << endl; cout << "1 - Encrypt File" << endl; cout << "2 - Decrypt File" << endl; cout << endl; cout << "Selection" << endl; cin >> choice; }
//Strtok_s Function to tokenize the entire message into separate words. string strtok_s() { //Message class object declaration Message m; //Function call encryptWord //m.encryptWord(); }
//Function Main int main() { //Variable declaration char message[1001]; //This code reads the data from the file into the array bool running = true; int selection{ 0 }; string edKey; //File object declaration ifstream inFile; //Program Logic //Vinegere class object declaration Vinegere v;
//Promp the user to enter an encryption/decryption key. cout << "Enter an encryption/decryption key: " << endl; cin >> edKey;
//Encryption key v.encrypt(edKey);
//Decryption key v.decrypt(edKey);
//Message class object declaration Message m;
//Display Menu displayMenu();
//Switch Statement switch (selection) { case '1': break; case '2': cout << "Enter the name of the file to decrypt: "; break; case '3': wait(); return 0; break; default: cout << "The selection is invalid. Please enter a number" << endl << endl; }
//Close file inFile.close();
//End of the program wait(); return 0;
}
//Wait function from Professor Sipantzi's template void wait() { //The following if-statement checks to see how many characters are in cin's buffer //If the buffer has characters in it, the clear and ignore methods get rid of them. if (cin.rdbuf()->in_avail() > 0) //If the buffer is empty skip clear and ignore { cin.clear(); cin.ignore(numeric_limits
//Message.cpp
//Message Implementation file
//Include Statements #include
//Constructors Message::Message() //default (zero argument) constructor { } //Second Constructor: k is the key passed in to the constructor, which is then passed in to the Vinegere class's setKey function. Message::Message(string k) { v.setKey(k); } //Void Encrypt Word Function void Message::encryptWord(char* token) { words.push_back(v.encrypt(token)); //string encryptKey; ////Prompt te user to enter the name of the file to encrypt //cout << "Enter the name of the file to encrypt: "; //cin >> encryptKey; } //Void DecryptWord Function void Message::decryptWord(char* token) { words.push_back(v.decrypt(token)); } //Void Make File Function void Message::makeFile(string fileName) { //File object declaration ifstream inFile;
cout << "Enter the name of the file: " << endl; getline(cin, fileName);
//Open file inFile.open(fileName); //Try to open the file
//An error check should go here to ensure that the file opened successfully. while (inFile.fail()) { cout << "The file you entered was not found. Please enter a valid file." << endl; //inFile.getline(message(), 1000); inFile.open(fileName); }
//Read in the file //inFile.getline(message(), 1000); } //Show Words Function void Message::showWords() { //for loop to show words for (string s : words) cout << s << " " << endl; }
Vinegere.cpp
//Vinegere Implementation file
//Include Statements #include
using namespace std;
//Constructors Vinegere::Vinegere() //default (zero argument) constructor { key = ""; } //Second Constructor Vinegere::Vinegere(string k) { key = k; } //Set Key Function void Vinegere::setKey(string k) { key = k; } //Get Key Function string Vinegere::getKey() { return key; } //To Upper Case Function string Vinegere::toUpperCase(string k) { toupper(k); } //Encrypt Function string Vinegere::encrypt(string word) { //Variable declaration string output; //For loop to encrypt the message for (int i = 0, j = 0; i < word.length(); ++i) { char c = word[i]; if (c >= 'a' && c <= 'z') c += 'A' - 'a'; else if (c < 'A' || c > 'Z') continue; output += (c + key[j] - 2 * 'A') % 26 + 'A'; //added 'A' to bring it in range of ASCII alphabet [ 65-90 | A-Z ] j = (j + 1) % key.length(); } return output; } //Decrypt Function string Vinegere::decrypt(string word) { //Variable declaration string output; //For loop to decrypt the message for (int i = 0, j = 0; i < word.length(); ++i) { char c = word[i]; if (c >= 'a' && c <= 'z') c += 'A' - 'a'; else if (c < 'A' || c > 'Z') continue; output += (c - key[j] + 26) % 26 + 'A';//added 'A' to bring it in range of ASCII alphabet [ 65-90 | A-Z ] j = (j + 1) % key.length(); } return output; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
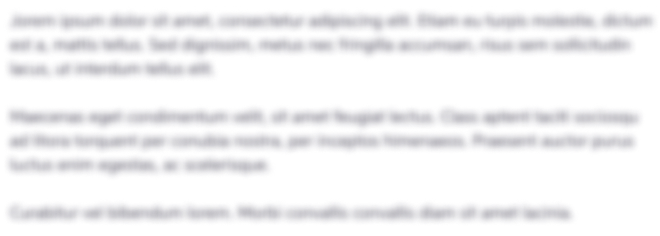
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started