Question
(I need help programming this code. If you can do it in Scala, that would be great. If not, Python works too. Thank you.) Write
(I need help programming this code. If you can do it in Scala, that would be great. If not, Python works too. Thank you.)
Write a tail recursive function `zipLists` that given two lists of integers `List(e1, ..., em)` and `List(f1, ..., fn)`, computes the list of pairs `List( (e1, f1), ..., (ek, fk) )` where =min(,).
The function takes in two arguments of type `List[Int]` and returns `List[(Int, Int)]`. You may have extra arguments but set them to default values or have an explicit helper function.
## Corner Cases - If one of the lists is empty, it must return empty list. ### Restrictions - Must be tail recursive. - Allowed list API functions/operations: `list.length`, `list.head`, `list.tail`, `list.reverse` and `::` (cons or append an element to the front of a list). - All other operations over lists are __not__ allowed. In particular do not use `zip`, `:+` (append to end) or `++` (join/concatenate two lists). - Do not try to convert the list into an array or some other data structure. - Use of vars/loops is not allowed.
### CODE
import scala.annotation.tailrec @tailrec // YOUR CODE HERE ???
//BEGIN TEST
val l1 = zipLists(Nil, List(5, 2, 1, 2, 3)) assert(l1 == Nil, s"Test 1 failed: expected : Nil, obtained: $l1")
val l2 = zipLists( List(5, 2, 1, 2, 3), Nil) assert(l2 == Nil, s"Test 2 failed: expected : Nil, obtained: $l2")
val l3 = zipLists(List(10), List(5, 2, 1, 2, 3)) assert(l3 == List((10,5)) , s"Test 3 failed: expected : List((10,5)), obtained: $l3")
val l4 = zipLists(List(0, 1, 2, 3, 4), List(-5, 2, -1, 2, -3)) assert(l4 == List( (0, -5), (1, 2), (2, -1), (3, 2), (4, -3) ), s"Test 4 failed: expected : List( (0, -5), (1, 2), (2, -1), (3, 2), (4, -3) ) , obtained: $l4")
val l5 = zipLists(List(1, 2, 3), List(5, 2, 1, 2, 3)) assert(l5 == List((1, 5), (2, 2), (3, 1)), s"Test 5 failed: List((1, 5), (2, 2), (3, 1)) expected : , obtained: $l5")
passed(8)
//END TEST
Step by Step Solution
There are 3 Steps involved in it
Step: 1
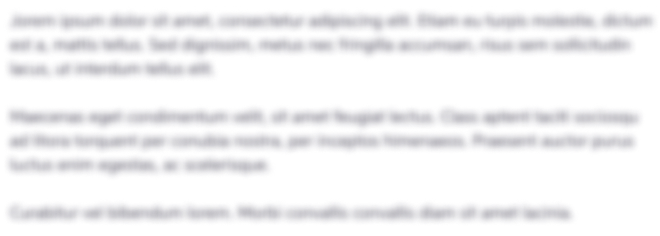
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started