Question
I need help to complete this code: #include pqueue.h // constructor pqueue::pqueue() { count = 0; // no jobs yet } // destructor does nothing
I need help to complete this code:
#include "pqueue.h"
// constructor
pqueue::pqueue()
{ count = 0; // no jobs yet
}
// destructor does nothing
pqueue::~pqueue() {}
// Purpose: to add a job to a right place in the Pqueue
// Argument: j is the job priority number
void pqueue::insertjob(int j)
{
// ** add the job j at the rear
trickleup(); // moves the job to the right place
}
// Purpose: to print a job and reheapify the Pqueue
void pqueue::printjob()
{
cout
reheapify();
}
// Purpose: to display all jobs
void pqueue::displayAll()
{ cout
// ** loop to display jobs from slot 0 to slot count-1 horizontally
}
// Utility functions follow: ------------------
// Purpose: to make the very last job trickle up to the right location
void pqueue::trickleup()
{
int x = count-1; // the very last job location
// ** while x is > 0
// compare Q[x] with the parent and if the parent is bigger swap & update x. Otherwise stop.
// You can call getParent to get the location of the parent
}
// Purpose: find the location of the parent
// The child location is given. Need to call even.
int getParent(int child)
{ // ** return the parent location
}
// Purpose: is location i even? Important in finding its parent location
bool pqueue::even(int i)
{
if ((i % 2) == 0) return true; else return false; }
// Purpose: to reheapify the Pqueue after printing
void pqueue::reheapify()
{
Q[0] = Q[count-1]; // move the last job to the front
count--;
// ** start at the root and repeat the following:
// find the location of the smaller child if you have not fallen off the tree yet
// if the smaller child is smaller than the parent, swap
// else stop
}
// Purpose: to find the location of the smaller child
// where children at locations 2*i+1 and 2*i+2
int pqueue::getSmallerchild(int i)
{
// ** return the location of the smaller child
}
#includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
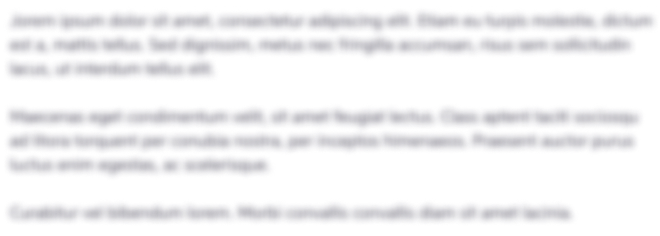
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started