Question
I need help with a code I have. It currently works however the program crashes whenever is pressed. I need the program to catch the
I need help with a code I have. It currently works however the program crashes whenever
- if it's Hours Worked: "that is not an integer > 0, try again"
- if it's Pay Rate: "pay rate must be greater than $5 but less than or equal to $15
then allow the user to enter another number in a line below.
Here's the code:
#include
#include
#include
using namespace std;
// Constant for the array size.
const int EMPLOYEE_SIZE = 7;
// Function Prototypes
void getEmployeeInfo(int empId[], int hours[], double payRate[], double wages[], int EMPLOYEE_SIZE);
void displayWages(int empId[], double wages[], int EMPLOYEE_SIZE);
void validateHoursWorked(string& str1, int& userNumber);
void validatePayRate(string& str1, double& userNumber);
int main()
{
int empID[] = { 1, 2, 3, 4, 5, 6, 7 }; // declare Array of employee ID numbers
int hours[EMPLOYEE_SIZE]; // declare Array to hold the hours worked for each employee
double payRate[EMPLOYEE_SIZE]; // declare Array to hold the hourly pay rate for each employee
double wages[EMPLOYEE_SIZE]; // declare Array to hold the gross wages for each employee
cout << "Enter the requested information for each employee. ";
// Get the employee payroll information and store it in the arrays.
// call the function getEmployeeInfo
getEmployeeInfo(empID, hours, payRate, wages, EMPLOYEE_SIZE);
// Display the payroll information.
// call the function displayWages.
displayWages(empID, wages, EMPLOYEE_SIZE);
system("pause");
return 0;
}
//function defitions
// ********************************************************
// The getEmployeeInfo function receives four parallel *
// arrays as arguments. The 1st array contains employee *
// IDs to be displayed in prompts. It asks for input and *
// stores hours worked and pay rate information in the *
// 2nd and 3rd arrays. This information is used to *
// calculate gross pay, which it stores in the 4th array. *
// ********************************************************
void getEmployeeInfo(int empId[], int hours[], double payRate[], double wages[], int EMPLOYEE_SIZE)
{
for (int i = 0; i < EMPLOYEE_SIZE; i++)
{
string str1;
int userNumberHours = 0;
double userNumberPay = 0;
double userNumberWages = 0;
cout << " Employee # " << empId[i] << endl;
cout << " Hours worked: ";
getline(cin, str1);
//call function validateHoursWorked
validateHoursWorked(str1, userNumberHours);
hours[i] = userNumberHours;
cout << " Pay rate: $";
getline(cin, str1);
//call function validatePayRate
validatePayRate(str1, userNumberPay);
payRate[i] = userNumberPay;
userNumberWages = userNumberHours * userNumberPay;
wages[i] = userNumberWages;
}
}
// ********************************************************
// The displayWages function receives 2 parallel arrays. *
// The first holds employee IDs and the second holds *
// employee gross pay. The function displays this *
// information for each employee. *
// ********************************************************
void displayWages(int empId[], double wages[], int EMPLOYEE_SIZE)
{
cout << "----------------------- " << "Employee Wages" << endl << "----------------------- ";
for (int i = 0; i < EMPLOYEE_SIZE; i++)
{
cout << "Employee #" << empId[i] << " $ "
<< fixed << setprecision(2) << wages[i] << " " << endl;
}
}
// ********************************************************
//The validateHoursWorked fuction receives a string and *
//returns an integer. The hours worked must be greater *
//than 0 but less than or equal to 40 *
// ********************************************************
//=================================================
void validateHoursWorked(string& str1, int& userNumber)
{
bool numberNotValid = true;
while (numberNotValid == true)
{
for (int i = 0; i < str1.length();) // ALLOW
{
if ((str1[i] != '0' && str1[i] != '1' && str1[i] != '2' && str1[i] != '3' && str1[i] != '4' && str1[i] != '5' && str1[i] != '6' && str1[i] != '7' && str1[i] != '8' && str1[i] != '9')) // FIX: EMPTY ENTER LETS IT THROUGH
{
i = 0;
cout << " Hours worked must be greater than 0 and less than or equal to 40 ";
cin.clear();
getline(cin, str1);
continue;
}
i++;
}
// Should be a working number for stoi, may not be valid number though for validation
userNumber = stoi(str1);
if (userNumber > 40 || userNumber <= 0)
{
str1 = "Invalid.";
numberNotValid = true;
}
else
numberNotValid = false;
}
}
// ********************************************************
//The validatePayRate fuction receives a string and *
//returns a double. The pay rate must be greater *
//than or equal to 5 but less than or equal to 15 *
// ********************************************************
void validatePayRate(string& str1, double& userNumber)
{
//hint: just as there is an stoi, theres is an stof (string to float) and an stod (string to double)
bool numberNotValid = true;
int periodCounter = 0;
int decimalCounter = 0;
while (numberNotValid == true)
{
for (int i = 0; i < str1.length();) // ALLOW
{
if (str1[i] == '.')
{
periodCounter++;
decimalCounter = str1.length() - 1 - i;
}
if ((str1[i] < '0' && str1[i] != '.') || (str1[i] > '9' && str1[i] != '.') || periodCounter > 1 || decimalCounter > 2) // FIX: EMPTY ENTER LETS IT THROUGH
{
periodCounter = 0;
decimalCounter = 0;
i = 0;
cout << " Pay rate must be greater than $5 but less than or equal to $15: ";
cin.clear();
getline(cin, str1);
continue;
}
i++;
}
userNumber = stod(str1);
if (userNumber > 15 || userNumber < 5)
{
str1 = "Invalid.";
numberNotValid = true;
}
else
numberNotValid = false;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
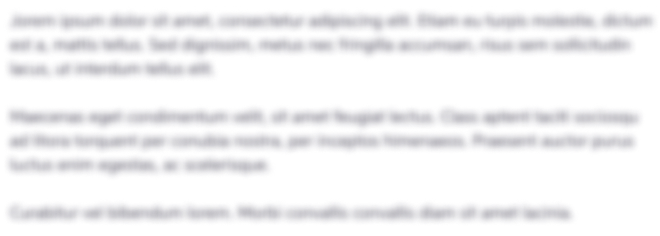
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started