Question
I need help with C++, Random File IO Write a program to keep track of a hardware store inventory. The store sells various items. For
I need help with C++, Random File IO
Write a program to keep track of a hardware store inventory. The store sells various items. For each item in the store, the following information is kept: item ID, item name, number of pieces ordered, number of pieces currently in the store, number of pieces sold, manufacturers price for the item, and the stores selling price. At the end of each week, the store manager would like to see a report in the following format:
Friendly Hardware Store
itemID itemName pOrdered pInStore pSold menufPrice sellingPrice 3 Circular Saw 150 110 40 45.00 125.00 17 Cooking Range 50 30 20 450.00 850.00 . . .
Total Inventory: $########.## Total number of items in the store: ______________
The total inventory is the total selling value of all the items currently in the store. The total number of items is the sum of the number of pieces of all the items in the store.
Your program must be menu driven, giving the user various choices, such as checking whether an item is in the store, selling an item, listing all items, and printing the report. After an item is sold, update the appropriate counts. Also include a menu option of new shipment that updates inventory based on new shipment received (based on a file). New shipment may contain items that were not originally carried.
Initially, the number of pieces (of an item) in the store is the same as the number of pieces ordered, and the number of pieces of an item sold is zero. The file for shipment consists data in the following format. This applies to initial input and also input for each new shipment received.
itemID pOrdered manufPrice sellingPrice itemName
You are asked to code the program using binary file IO. A binary file stores record of each item in fixed number of bytes and allows the program to jump into any particular record to retrieve or modify. To retrieve a record from a binary file, you use the read function to read certain number of bytes from that record position into a destination variable. To modify a record, you use the write function to write certain number of bytes to that record position. Binary file IO directly deal with raw bytes and avoid the conversion between raw bytes and information so the program runs efficiently. A binary file generated by a program can be used by other program as long as that program knows the structure of the records and able to interpret these raw bytes.
For simplicity, the program will assume the hardware store can only carry up to 200 items, and use the item ID as the record number. So item ID of 3 will be written into the third record position, and item ID of 17 will be written into the 17th record position. If there is no item with item ID of 4, then record position 4 is empty. For any empty record the item ID is set to zero. Valid record numbers are 1 to 200. So a record number of 221 is an invalid number.
The program should first provide a menu asking whether it is creating a new inventory or working with an existing one. If creating a new inventory, it creates file hardware.dat if the file doesnt exist, or wipes out everything on the file and starts over if the file exists. It will then initialize 200 empty records and load the initial data from a file. To load a file means to write each item on the input file to the corresponding record position in the inventory file hardware.dat according to the item ID value. If working with existing inventory, then it will open file hardware.dat, check for successful opening, and then proceed. The records on file hardware.dat should not be altered by the opening process.
Define a class for item to encapsulate the above mentioned seven piece of information, i.e., item ID, item name, number of pieces ordered, number of pieces currently in the store, number of pieces sold, manufacturers price for the item, and the stores selling price. Make item name an array of 30 characters. Define public functions that are necessary for this program. What public functions are needed? You make the decision when you plan for the solution.
For an empty record, the item ID is 0, item name is , and the rest are all zero. To set a char array to , you assign \0 to the first character on the array.
Once the file is opened, the program should proceed to display the store menu. The store menu could look like this:
Store Menu 1 Check availability 2 Sell item 3 List all items 4 New shipment 5 Print inventory report 6 Exit
When check item availability is chosen, it will ask for item ID, read the item from hardware.dat, and display the item information if item ID is not zero. If item ID is zero, it will inform that item does not exist and then display the store menu.
When sell item is chosen, it will ask for item ID. If item ID is not zero, it will display item info, ask how many the customer wants to purchase, display how much customer should pay, update the item piece in store and piece sold, and then write the item back to hardware.dat, and then display the store menu. If item ID is zero, it will inform the user that item is not available and display the store menu.
When list all items is chosen, it will display all the items currently in store (i.e., item ID is not zero) in a tablet form, and then display the store menu.
When print inventory report is chosen, it will display the report formatted as shown above, and then display the store menu.
Some input validation is required. You should use a client function that displays store menu and returns user choice. The return value must be valid value, 1 to 6. It should insist on getting a valid user choice until it gets one and then returns it. Define another client function that prompts and gets record number. It should also be stubborn at returning a valid record number between 1 and 200.
The file opening menu should be treated the same way. If plan well, you may define one menu function that can be used for both file menu and store menu. For file IO, you should always check for successful file opening. There is no point to continue if the file cannot be opened.
How to handle this project:
Please read this guideline a few times to fully understand what needs be done. Ask for clarification if any part is not clear.
Once understood, spend some time to plan for a solution. What to be included in the item class should be only related to the item itself. The rest are client that uses the item class.
The client part has a lot of operations. It is necessary to think through the logic and write a brief version of pseudo code to plan for the client part. The plan phase may need a few iterations, that is plan, walk through, revise, and walk through again.
Once your plan is verified, you can start coding. When code the program, first code the class, and then the client.
text file:
2 10 57.98 110.99 Electric sander 3 150 45.00 125.00 Circular Saw 11 76 11.99 19.99 Hammer 17 50 450.00 850.00 Cooking Range 24 25 11.00 22.00 Jig saw 39 30 79.50 168.99 Lawn mower 56 20 99.99 199.99 Power saw 68 106 6.99 14.49 Screwdriver 77 50 21.50 45.00 Sledge hammer 83 100 7.50 15.99 Wrench 12 20 250.50 550.50 Dish Washer 22 75 150.00 400.00 Micro Wave 55 100 12.00 28.99 Big Black Bucket 66 77 13.99 19.99 Easter Bunny
Step by Step Solution
There are 3 Steps involved in it
Step: 1
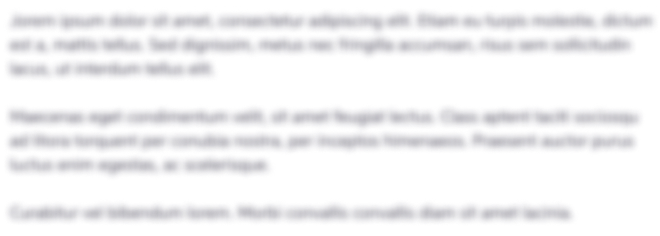
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started