Question
I need help with getting started for this in Java. Codes listed below. Complete the CircularArrayQueue.java class implementation of the Queue.java interface so that it
I need help with getting started for this in Java. Codes listed below.
Complete the CircularArrayQueue.java class implementation of the Queue.java interface so that it does not use a count field. This implementation must have only the following fields:
DEFAULT_CAPACITY
front
rear
queue
Implement the methods
enter
leave
front
isEmpty
isFull
expandCapacity
Write a test class to test each of the methods you implement.
Queue.java
public interface Queue
/** * The front element leaves the queue and is returned. * @throws java.util.NoSuchElementException if queue is empty. */ public E leave();
/** * Returns True if the queue is empty. */ public boolean isEmpty();
/** * Returns the front element without removing it. * @throws java.util.NoSuchElementException if queue is empty. */ public E front(); }
CircularArrayQueue.java
import java.util.NoSuchElementException; import java.util.StringJoiner;
/** * A queue implemented using a circular buffer. * * @author (your name) * @version (a version number or a date) */ public class CircularArrayQueue
public CircularArrayQueue(int initialCapacity) { queue = (E[]) new Object[initialCapacity]; front = 0; rear = 0; }
public CircularArrayQueue() { this(DEFAULT_CAPACITY); } @Override public void enter(E element) { // Implement as part of assignment }
@Override public E leave() throws NoSuchElementException { // Implement as part of assignment }
@Override public E front() throws NoSuchElementException { // Implement as part of assignment } @Override public boolean isEmpty() { // Implement as part of assignment } private boolean isFull() { // Implement as part of assignment }
private int incrementIndex(int index) { return (index + 1) % queue.length; } private void expandCapacity() { // Implement as part of assignment } @Override public String toString() { StringJoiner result = new StringJoiner("[", ", ", "]"); for (int i = front; i != rear; i = incrementIndex(i)) { result.add(queue[i].toString()); } return result.toString(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
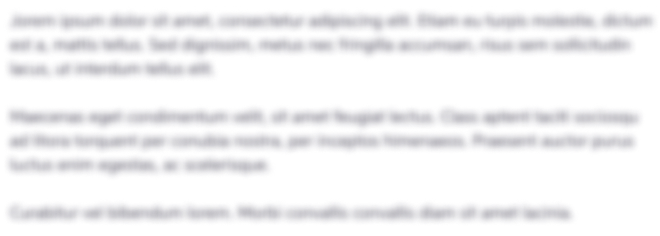
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started