Question
I need help with my code. My code works fine but it can only run twice. Basically when you run the program once and you
I need help with my code. My code works fine but it can only run twice. Basically when you run the program once and you finish the game it askes you if another player wants to play. After I run the program twice it automatically ends without asking me if another player wants to play. If someone can please take a look at my code and help me with that I would greatly appreciate that:
import java.util.Random; import java.util.Scanner; /** * PigGame.java contains main method and other methods to play Pig game * with computer */ public class PigGame { // Total points to be achieved to win the game public static final int POINTS = 30; // points to forfeit public static final int FORFEIT_POINTS = 20; /* * main method to execute the program */ public static void main(String[] args) { // random object to create random values for dice, names, coin flip Random random = new Random(); // scanner object to read the input from the file Scanner scanner = new Scanner(System.in); // call to play method to start the game description(); play(scanner, random); System.out.println("Is there another player?"); // if user wants to play game again while (scanner.next().equalsIgnoreCase("yes")) { description(); String human = scanner.nextLine(); play(scanner, random); } } public static void description() { System.out.println("***********************************************************************************"); System.out.println("* You are about to play the pig game against the computer. *"); System.out.println("* On each turn, the current player will roll a pair of dice *"); System.out.println("* and acumulates points. The goal is to reach to 30 points *"); System.out.println("* before your opponent does. If, on any turn, the player roll *"); System.out.println("* all the points accumulated for that round are forfeited and *"); System.out.println("* the control of the dice moves to the other player. If the player *"); System.out.println("* rolls two 1s in one turn , the player loses all the points *"); System.out.println("* accumulated thus far and forfeit and the control moves to the *"); System.out.println("* other player. The player may voluntarily turn over the dice after *"); System.out.println("* each roll. Therefore player must decide to roll again(be a pig) *"); System.out.println("* and risk losing points , or relinquish control of the dice, possibly *"); System.out.println("* allowing the other player to win. *"); System.out.println("* Computer is going to flip a coin to choose the first player *"); System.out.println("***********************************************************************************"); } // to check if input is yes or no public static boolean isValid(String input, Scanner scanner) { if (input.equalsIgnoreCase("yes") || input.equalsIgnoreCase("no")) { return true; } return false; } /** * @returns the heads or tails based on the random number generated */ public static String flipCoin(Random random) { int output = random.nextInt(2); if (output == 0) { return "Head"; } else { return "Tail"; } } /* * returns the sum of the two dice by creating random number for each die * and adding both, if any of the die value is 1 returns 0, prints the * values of dice1 and dice2 to the console */ public static int rollDice(Random random) { // variable to store value for firstDie int firstDie = random.nextInt(6) + 1; // variable to store value for second die int secondDie = random.nextInt(6) + 1; System.out.println("dice1: " + firstDie); System.out.println("dice2: " + secondDie); if (firstDie == 1 || secondDie == 1) { return 0; } return firstDie + secondDie; } /** * returns the name by randomly selecting one of the name given in * computerNames array */ public static String selectName(Random random) { String[] computerNames = { "Porky", "Ginger", "Tom", "John", "Mary", "Robert", "Josh", "Mike", "Adam", "Gary" }; String randomName = computerNames[random.nextInt(10)]; return randomName; } /** * used to play the game, creates computer and human and assigns names and * scores correspondingly and declares the winner */ public static void play(Scanner scanner, Random random) { // select random name for computer String computer = selectName(random); // variable to store the winner if 1 computer if 2 human playing with // computer int winner = 0; int computerScore = 0; int humanScore = 0; int activePlayer = 0; System.out.println("Lets start the fun"); System.out.println(); System.out.println(); System.out.println("Hi my name is " + computer); System.out.print("What is your name?"); String human = scanner.nextLine(); System.out.println("Hi " + human + ", I am fliping the coin to determine who goes first press any key to start the game."); scanner.nextLine(); String coinResult = flipCoin(random); if (coinResult.equals("Head")) { System.out.println(computer + " is going to start the game."); activePlayer = 1; } else { System.out.println(human + " is going to start the game."); activePlayer = 2; } while (winner == 0) { while (activePlayer == 1) { System.out.println(computer + "'s turn"); System.out.println("Points: " + computerScore); int diceSum = rollDice(random); if (diceSum == 0) { System.out.println("Sorry " + computer + " you lost the points for this turn"); computerScore = 0; activePlayer = 2; } computerScore += diceSum; if (computerScore >= POINTS) { System.out.println("Hurray !!!!"); System.out.println("You reached or passed 30 points"); winner = 1; activePlayer = 0; } else if (computerScore > FORFEIT_POINTS) { System.out.println("I am forfeiting my turn since I have 21 which is more than 20 points."); activePlayer = 2; } System.out.println("Points: " + computerScore); System.out.println(); System.out.println("Press any key to continue"); scanner.nextLine(); } while (activePlayer == 2) { System.out.println(human + "'s turn"); System.out.println("Points: " + humanScore); int diceSum = rollDice(random); if (diceSum == 0) { System.out.println("Sorry " + human + " you lost the points for this turn"); humanScore = 0; activePlayer = 1; } humanScore += diceSum; System.out.println("Points: " + humanScore); if (humanScore >= POINTS) { System.out.println("Hurray !!!!"); System.out.println("You reached or passed 30 points"); winner = 2; activePlayer = 0; } else if (humanScore > 0) { System.out.println("Do you want to forfeit your turn?"); String check = scanner.next(); if (check.equalsIgnoreCase("yes")) { activePlayer = 1; } } System.out.println(); System.out.println("Press any key to continue"); scanner.nextLine(); System.out.println(); } } System.out.println("Hurray!!! We have a winner Somebody got 30 or more"); System.out.println(human + " points " + humanScore); System.out.println(computer + " points " + computerScore); if (winner == 1) { System.out.println(computer + " won the game."); } else { System.out.println(human + " won the game."); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
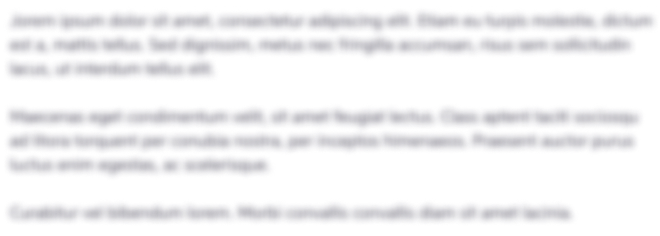
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started