Question
I need help with my Computer Science 2 project. Develop a high-quality, menu-driven object-oriented C++ program that creates a small database, using a binary search
I need help with my Computer Science 2 project.
Develop a high-quality, menu-driven object-oriented C++ program that creates a small database, using a binary search tree structure to store and process the data. The database will contain the top 100 highest grossing films of 2015.
The C++ object-oriented program must use the BinarySearchTree class from the textbook (along with the BinaryNode, BinaryNodeTree, BinaryTreeInterface, NotFoundException,and PrecondViolatedExcep classes it requires) to fulfill the requirements of this project. When designing and implementing the program, apply good software engineering principles. Create a makefile for the program that allows compilation and execution of the program from within jGRASP using the Windows operating system. Start the analysis and design process by drawing a complete UML class diagram for the program that includes all the classes that are contained in the program, including the classes provided by the textbook and the classes that you create. The UML class diagram will assist you in understanding the components of the project.
Your program must include:
a Film class that stores all the data for a Film and provides appropriate methods to support good software engineering principles,
a FilmDatabase class that stores the binary search tree and provides appropriate methods to support good software engineering principles,
a Menu class that contains, as a minimum, a method for each menu used in the program that displays the menu and responds to all menu choices made by the user, calling appropriate methods in the FilmDatabase class as necessary, and
a file named Project1.cpp that contains the main() method.
You may also include other classes, as needed. Note that the binary search tree must store Film objects, and the >, <, and == operators must be defined for that class because the BinarySearchTree class uses those overloaded operators.
The downloaded files include the Student class (Student.h and Student.cpp), the StudentDatabase class (StudentDatabase.h and StudentDatabase.cpp), BSTTest.cpp, and makefile for you to use as an example to help you begin your program. The program compiles, links, and executes if you Make it using jGRASP/Windows.
Data Details:
The database contains data pertaining to the 100 highest grossing films of 2015. A comma delimited file named Films2015.csv contains the initial data. Each record is stored on one line of the file in the following format:
Data Data type
Rank int
Film Title (key) string
Studio string
Total Gross double
Total Theaters int
Opening Gross double
Opening Theaters int
Opening Date string
Each of the data fields is separated in the file using the comma (,) character as a delimiter. There is no comma (,) character after the last field on the line. The data is considered clean; There are no errors in the input file.
When storing the data in the binary search tree, use the data types shown above. The Film Title will serve as the key field (its value is unique).
Menu Details:
Your program must begin by inputting the text data from the Films2015.csv text file and building a binary search tree for the Films, in order by the key (the film title). Then the program must display the following Main Menu:
MAIN MENU
D - Describe the Program
R Reports
S - Search the Database
X - Exit the Program
Enter Selection ->
All menu choices are selected by typing the number of the choice.
D - Describe the Program
If the user chooses Describe the Program, the program provides a detailed description for the user, explaining what the program is doing and how it works. Note that this method does NOT substitute for javadoc-style comments. The audience for this method consists of non-technical users that have no information at all about the assignment. After providing the description, display the MAIN MENU again. Do NOT use recursion to do this; use a loop.
R - Reports
If the user chooses Reports from the MAIN MENU, the program displays the following menu:
REPORTS MENU T - Order by Film Title report R - Order by Rank report X - Return to main menu
Enter Selection ->
If the user chooses Order by Film Title report from the REPORTS MENU, the program should do the following:
Display a report in order by Film Title that contains all the data for each record stored in the binary search tree.
Identify the report and label all the data displayed appropriately.
If the user chooses Order by Rank report from the REPORTS MENU, the program should do the following:
Display a report sorted in increasing order by earnings rank that contains all the data for each record stored in the binary search tree. Note that this does not involve a simple tree traversal or single method call. You will need to write code that specifically performs this function. You may assume that the rank is never less than 1 and never greater than 100. Do NOT copy the data into another binary search tree, into an array, into a linked list, vector or into any other type of data structure. Retrieve the data directly from the binary search tree which is stored in order by the Film Title.
Identify the report and label all the data displayed appropriately.
If the user chooses Return to main menu from the REPORTS MENU, then display the MAIN MENU again. Do NOT use recursion to do this; use a loop.
S - Search the Database
If the user chooses Search the database, the program should display another menu, as follows:
Search Menu
T - Search by Title
K - Search by Keyword(s)
S - Search by Studio
M - Search by month of release
X - Return to main menu
Enter Selection ->
If the user chooses Search by Title, the program should do the following:
Request a film title from the user.
Search the database for the title given. This will be an exact match of the film title and at most, only 1 record will match. A case insensitive search should be performed.
If the film title is found, display the record stored in the binary search tree. Otherwise report that the requested film title was not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Search by Keyword(s), the program should do the following:
Request title keywords from the user.
Search the database for all titles that contain the keyword(s). The keywords given represent all or a portion of the title. When multiple keywords are given, only titles that contain the keywords in the order given will be selected. For example, if the user enters Star Wars, Star Wars: The Force Awakens will be selected as will The Making of Star Wars. However, One Star, Many Wars, Once Upon a Star, Wars and Wars with Stars will not be selected.
Multiple search keywords may be entered by separating the keywords with a comma. All titles containing one or more of the keywords will be selected. For example, if the user enters 'Cinderella,SpongeBob,Dinosaur', "Cinderella", "The Good Dinosaur", "In Search of Dinosaurs", "The SpongeBob Movie: Sponge Out of Water" as well as "Cinderella Loves Dinosaurs and SpongeBob!" will be selected.
Display all records stored in the binary search tree where the title contains the keyword(s) given by the user. If there are no records containing the keyword(s), report that the requested keyword(s) were not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Search by Studio, the program should do the following:
Request the name of a Studio from the user.
Search the database for an exact match of the studio name.
Display all records stored in the binary search tree where the studio name matches the name given by the user. If there are no records with the studio name given, report that the requested studio was not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Search by month of release, the program should do the following:
Request a month value between 1 and 12 from the user.
Search the database for all records where the release date contains the month requested. Note: the date is stored as a string.
Display all records stored in the binary search tree that match the month given by the user. If there are no records with the requested release month, report that the requested month was not found.
Identify the output and label all the data displayed appropriately.
If the user chooses Return to main menu, then display the MAIN MENU again. Do NOT use recursion to do this; use a loop.
X Exit the Program
If the user chooses Exit the Program from the MAIN MENU, the program ends.
Since the program is controlled and manipulated by a human user, be sure to perform adequate error checking on every aspect of the program.
Be sure to follow the Project Guidelines & Evaluation Criteria, since the project will be evaluated using this criteria. In addition, be sure to use javadoc-style comments appropriately. When creating javadoc-style comments, keep in mind that the comment will eventually become part of an html file that will be used by other programmers on your programming team, and by maintenance programmers. Remember also that maintenance programmers have not seen the assignment (the specification), so the information you are providing here must provide all of the detailed information another programmer will need to completely understand the program and to maintain the code.
Here's the student class and the BSTTest program. The programs should look like this.
************************* Student.h *************************
#ifndef STUDENT_H
#include
#include
using namespace std;
// Name of file in which to save all output data
const string OUTPUT_FILENAME = "studentOutFile.txt";
class Student
{
private:
// Student's name, the key
string name;
// Student's id number - automatically generated by the constructor
int idNum;
// Student's telephone number, including area code and dashes
string phoneNumber;
// Static class members
/* A static variable is shared among all instances of a class
and may be accessed without an instance of the class.
Static class members MUST be defined and intialized
in the implementation file.
*/
// Static variable used to initialize the student id number
static int idNumbers;
// Output file to store the data for a student
static ofstream outFile;
public:
/**
* Default constructor
*/
Student();
/**
* Overloaded constructor
* @param name - student's name; value for search key
* @param newPhoneNumber - value for student's phone number
*/
Student(const string& name,
const string& newPhoneNumber);
/**
* Accessor for the name.
* @return name of this person, the key
*/
string getName(void);
/**
* Displays all the data stored for a student.
*/
void printStudent(void);
/**
* Inputs data for a student object, providing specific instructions
* for the user and validating the input data.
*/
void inputStudent(void);
/**
* Outputs the data to the output file.
*/
void saveData();
/**
* Defines the > operator for a Student object.
* @param right - right hand Student object for comparison
* to the this Student object using >
*/
bool operator> (const Student &right);
/**
* Defines the < operator for a Student object.
* @param right - right hand Student object for comparison
* to the this Student object using <
*/
bool operator< (const Student &right);
/**
* Defines the == operator for a Student object.
* @param right - right hand Student object for comparison
* to the this Student object using ==
*/
bool operator== (const Student &right);
/**
* Closes the output file. This method MUST be called before the
* program terminates.
*/
static void closeOutputFile();
}; // end Student
#define STUDENT_H
#endif
************************* Student.cpp *************************
#include "Student.h" #include
using namespace std;
// Define and initialize the static class members shared by all instances int Student::idNumbers = 100; // used to automatically generate idNum ofstream Student::outFile(OUTPUT_FILENAME.c_str()); // open output file // output file Student::Student() { idNum = idNumbers++; // auto initialize the idNum to the next available number }
Student::Student(const string& newName, const string& newPhoneNumber) : name(newName), phoneNumber(newPhoneNumber) { idNum = idNumbers++; // auto initialize the idNum to the next available number }
string Student::getName(void) { return name; }
void Student::printStudent(void) { cout << left << "Name: " << setw(10) << name << " IdNum: " << setw(5) << idNum << " Phone: " << phoneNumber << endl; }
void Student::inputStudent(void) { string inputData; bool validInput = false; while (!validInput) { cout << "Enter last name for new person. Use uppercase for only the" << endl << "first letter:" << endl; getline(cin, inputData); if (isupper(inputData[0])) { name = inputData; validInput = true; } else cout << inputData << " is an invalid name. Please try again." << endl; } validInput = false; while (!validInput) { cout << "Enter phone number for new student in the form ###-###-####:" << endl; getline(cin, inputData); if (inputData.length() == 12 && isdigit(inputData[0]) && isdigit(inputData[1]) && isdigit(inputData[2]) && inputData[3] == '-' && isdigit(inputData[4]) && isdigit(inputData[5]) && isdigit(inputData[6]) && inputData[7] == '-' && isdigit(inputData[8]) && isdigit(inputData[9]) && isdigit(inputData[10]) && isdigit(inputData[11])) { phoneNumber = inputData; validInput = true; } else cout << inputData << " is an invalid phone number. Please try again." << endl; } }
void Student::saveData() { if (outFile) outFile << name << "," << idNum << "," << phoneNumber << endl; else cout << "Error: Output file " << OUTPUT_FILENAME << " is not ready for output." << endl << endl; }
bool Student::operator> (const Student &right) { return (name > right.name); }
bool Student::operator< (const Student &right) { return (name < right.name); }
bool Student::operator== (const Student &right) { return (name == right.name); }
void Student::closeOutputFile() { outFile.close(); }
************************* BSTTest.cpp *************************
#include "StudentDatabase.h" #include
void explanation(void);
int main (void) { explanation(); StudentDatabase studentDB; // database to store Student objects studentDB.createDatabase(); studentDB.displayData(); studentDB.saveDatabase();
return EXIT_SUCCESS; }
/** * Provides an explanation of the program for the user */ void explanation(void) { cout << "This program demonstrates the use of a binary search tree" << endl; cout << "to store items of the Student class type to help CTP 250" << endl; cout << "students understand how to use the binary search tree classes" << endl; cout << "provided by the textbook. The program creates eight arbitrary" << endl; cout << "pre-defined Student objects, which consist of name, id," << endl; cout << "and phone, and adds them to a binary search tree. It then" << endl; cout << "inputs data for a new Student object from the user, giving" << endl; cout << "the user specific instructions about data entry. The program" << endl; cout << "adds the entered Student object to the binary search tree." << endl << endl; cout << "The program searches for a specific student in the tree, and" << endl; cout << "deletes that student, if found. The program then displays the" << endl; cout << "data in the binary search tree using an inorder traversal," << endl; cout << "which results in an alphabetical listing of Student objects" << endl; cout << "in the tree. Next, the program displays the contents of the" << endl; cout << "tree using a preorder and a postorder traversal, so the order" << endl; cout << "of the Student objects appears random. Before exiting, the"<< endl; cout << "program saves the data for each Student object in the tree" << endl; cout << "to a text file using a preorder traversal." << endl << endl; }
************************* Film.h *************************
************************* Film.cpp *************************
************************* FilmDatabase.h *************************
************************* FilmDatabase.cpp *************************
************************* Menu.h *************************
************************* Menu.cpp *************************
************************* Project.cpp *************************
Films2015.csv
1 | Star Wars: The Force Awakens | BV | 9.16E+08 | 4134 | 2.48E+08 | 4134 | ######## |
2 | Jurassic World | Uni. | 6.52E+08 | 4291 | 2.09E+08 | 4274 | ######## |
3 | Avengers: Age of Ultron | BV | 4.59E+08 | 4276 | 1.91E+08 | 4276 | 5/1/2015 |
4 | Inside Out | BV | 3.56E+08 | 4158 | 90440272 | 3946 | ######## |
5 | Furious 7 | Uni. | 3.53E+08 | 4022 | 1.47E+08 | 4004 | 4/3/2015 |
6 | Minions | Uni. | 3.36E+08 | 4311 | 1.16E+08 | 4301 | ######## |
7 | The Hunger Games: Mockingjay - Part 2 | LGF | 2.81E+08 | 4175 | 1.03E+08 | 4175 | ######## |
8 | The Martian | Fox | 2.28E+08 | 3854 | 54308575 | 3831 | ######## |
9 | Cinderella (2015) | BV | 2.01E+08 | 3848 | 67877361 | 3845 | ######## |
10 | Spectre | Sony | 2E+08 | 3929 | 70403148 | 3929 | ######## |
11 | Mission: Impossible - Rogue Nation | Par. | 1.95E+08 | 3988 | 55520089 | 3956 | ######## |
12 | Pitch Perfect 2 | Uni. | 1.84E+08 | 3660 | 69216890 | 3473 | ######## |
13 | Ant-Man | BV | 1.8E+08 | 3868 | 57225526 | 3856 | ######## |
14 | Home (2015) | Fox | 1.77E+08 | 3801 | 52107731 | 3708 | ######## |
15 | Hotel Transylvania 2 | Sony | 1.7E+08 | 3768 | 48464322 | 3754 | ######## |
16 | Fifty Shades of Grey | Uni. | 1.66E+08 | 3655 | 85171450 | 3646 | ######## |
17 | The SpongeBob Movie: Sponge Out of Water | Par. | 1.63E+08 | 3680 | 55365012 | 3641 | 2/6/2015 |
18 | Straight Outta Compton | Uni. | 1.61E+08 | 3142 | 60200180 | 2757 | ######## |
19 | The Revenant | Fox | 1.6E+08 | 3711 | 474560 | 4 | ######## |
20 | San Andreas | WB | 1.55E+08 | 3812 | 54588173 | 3777 | ######## |
21 | Mad Max: Fury Road | WB | 1.54E+08 | 3722 | 45428128 | 3702 | ######## |
22 | Daddy's Home | Par. | 1.47E+08 | 3483 | 38740203 | 3271 | ######## |
23 | The Divergent Series: Insurgent | LG/S | 1.3E+08 | 3875 | 52263680 | 3875 | ######## |
24 | The Peanuts Movie | Fox | 1.3E+08 | 3902 | 44213073 | 3897 | ######## |
25 | Kingsman: The Secret Service | Fox | 1.28E+08 | 3282 | 36206331 | 3204 | ######## |
26 | The Good Dinosaur | BV | 1.21E+08 | 3749 | 39155217 | 3749 | ######## |
27 | Spy | Fox | 1.11E+08 | 3715 | 29085719 | 3711 | 6/5/2015 |
28 | Trainwreck | Uni. | 1.1E+08 | 3171 | 30097040 | 3158 | ######## |
29 | Creed | WB | 1.09E+08 | 3502 | 29632823 | 3404 | ######## |
30 | Tomorrowland | BV | 93436322 | 3972 | 33028165 | 3972 | ######## |
31 | Get Hard | WB | 90411453 | 3212 | 33803253 | 3175 | ######## |
32 | Terminator: Genisys | Par. | 89760956 | 3783 | 27018486 | 3758 | 7/1/2015 |
33 | Taken 3 | Fox | 89256424 | 3594 | 39201657 | 3594 | 1/9/2015 |
34 | Sisters | Uni. | 86623660 | 2978 | 13922855 | 2962 | ######## |
35 | Alvin and the Chipmunks: The Road Chip | Fox | 84170141 | 3705 | 14287159 | 3653 | ######## |
36 | Maze Runner: The Scorch Trials | Fox | 81697192 | 3792 | 30316510 | 3791 | ######## |
37 | Ted 2 | Uni. | 81476385 | 3448 | 33507870 | 3442 | ######## |
38 | Goosebumps | Sony | 80021740 | 3618 | 23618556 | 3501 | ######## |
39 | Pixels | Sony | 78747585 | 3723 | 24011616 | 3723 | ######## |
40 | Paddington | W/Dim. | 76271832 | 3355 | 18966676 | 3303 | ######## |
41 | The Intern | WB | 75764672 | 3320 | 17728313 | 3305 | ######## |
42 | Bridge of Spies | BV | 72086318 | 2873 | 15371203 | 2811 | ######## |
43 | Paul Blart: Mall Cop 2 | Sony | 71038190 | 3633 | 23762435 | 3633 | ######## |
44 | War Room | TriS | 67790117 | 1945 | 11351389 | 1135 | ######## |
45 | Magic Mike XXL | WB | 66013057 | 3376 | 12857184 | 3355 | 7/1/2015 |
46 | The Big Short | Par. | 65881837 | 2529 | 705527 | 8 | ######## |
47 | The Visit | Uni. | 65206105 | 3148 | 25427560 | 3069 | ######## |
48 | The Wedding Ringer | SGem | 64460211 | 3003 | 20649306 | 3003 | ######## |
49 | Black Mass | WB | 62575678 | 3188 | 22635037 | 3188 | ######## |
50 | Vacation | WB (NL) | 58884188 | 3430 | 14681108 | 3411 | ######## |
51 | The Perfect Guy | SGem | 57027435 | 2230 | 25888154 | 2221 | ######## |
52 | Joy | Fox | 56119692 | 2924 | 17015168 | 2896 | ######## |
53 | Fantastic Four | Fox | 56117548 | 4004 | 25685737 | 3995 | 8/7/2015 |
54 | Focus (2015) | WB | 53862963 | 3323 | 18685137 | 3323 | ######## |
55 | The Hateful Eight | Wein. | 53350994 | 2938 | 4610676 | 100 | ######## |
56 | Southpaw | Wein. | 52421953 | 2772 | 16701294 | 2772 | ######## |
57 | Insidious Chapter 3 | Focus | 52218558 | 3014 | 22692741 | 3002 | 6/5/2015 |
58 | Poltergeist (2015) | Fox | 47425125 | 3242 | 22620386 | 3240 | ######## |
59 | Jupiter Ascending | WB | 47387723 | 3181 | 18372372 | 3181 | 2/6/2015 |
60 | Sicario | LGF | 46889293 | 2620 | 401288 | 6 | ######## |
61 | The Man From U.N.C.L.E. | WB | 45445109 | 3673 | 13421036 | 3638 | ######## |
62 | McFarland USA | BV | 44482410 | 2792 | 11020798 | 2755 | ######## |
63 | The Gift (2015) | STX | 43787265 | 2503 | 11854273 | 2503 | 8/7/2015 |
64 | Everest (2015) | Uni. | 43482270 | 3009 | 7222035 | 545 | ######## |
65 | The Night Before | Sony | 43035725 | 2960 | 9880536 | 2960 | ######## |
66 | Krampus | Uni. | 42725475 | 2919 | 16293325 | 2902 | ######## |
67 | Max (2015) | WB | 42656255 | 2870 | 12155254 | 2855 | ######## |
68 | The Age of Adaline | LGF | 42629776 | 3070 | 13203458 | 2991 | ######## |
69 | The Longest Ride | Fox | 37446117 | 3371 | 13019686 | 3366 | ######## |
70 | Spotlight | ORF | 37337021 | 1089 | 295009 | 5 | ######## |
71 | The Boy Next Door | Uni. | 35423380 | 2615 | 14910105 | 2602 | ######## |
72 | Pan | WB | 35088320 | 3515 | 15315435 | 3515 | ######## |
73 | Hot Pursuit | WB | 34580201 | 3037 | 13942258 | 3003 | 5/8/2015 |
74 | Concussion (2015) | Sony | 34255169 | 2841 | 10513749 | 2841 | ######## |
75 | Brooklyn | FoxS | 34221253 | 962 | 187281 | 5 | ######## |
76 | The DUFF | LGF | 34030343 | 2622 | 10809149 | 2575 | ######## |
77 | Woman in Gold | Wein. | 33307793 | 2011 | 2091551 | 258 | 4/1/2015 |
78 | The Second Best Exotic Marigold Hotel | FoxS | 33078266 | 2022 | 8540370 | 1573 | 3/6/2015 |
79 | Unfriended | Uni. | 32482090 | 2775 | 15845115 | 2739 | ######## |
80 | Entourage | WB | 32363404 | 3108 | 10283250 | 3108 | 6/3/2015 |
81 | Paper Towns | Fox | 32000304 | 3031 | 12650140 | 3031 | ######## |
82 | Chappie | Sony | 31569268 | 3201 | 13346782 | 3201 | 3/6/2015 |
83 | Crimson Peak | Uni. | 31090320 | 2991 | 13143310 | 2984 | ######## |
84 | A Walk in the Woods | BG | 29504281 | 2158 | 8246267 | 1960 | 9/2/2015 |
85 | Point Break (2015) | WB | 28661671 | 2910 | 9800252 | 2910 | ######## |
86 | Sinister 2 | Focus | 27740955 | 2799 | 10542116 | 2766 | ######## |
87 | The Last Witch Hunter | LG/S | 27367660 | 3082 | 10812861 | 3082 | ######## |
88 | No Escape | Wein. | 27288872 | 3415 | 8111264 | 3355 | ######## |
89 | Ricki and the Flash | TriS | 26822144 | 2064 | 6610961 | 1603 | 8/7/2015 |
90 | The Woman in Black 2: Angel of Death | Rela. | 26501323 | 2602 | 15027415 | 2602 | 1/2/2015 |
91 | Run All Night | WB | 26461644 | 3171 | 11012305 | 3171 | ######## |
92 | Love the Coopers | LGF | 26302731 | 2603 | 8317545 | 2603 | ######## |
93 | The Lazarus Effect | Rela. | 25801047 | 2666 | 10203437 | 2666 | ######## |
94 | Ex Machina | A24 | 25442958 | 2004 | 237264 | 4 | ######## |
95 | In the Heart of the Sea | WB | 25020758 | 3103 | 11053366 | 3103 | ######## |
96 | The Gallows | WB | 22764410 | 2720 | 9808463 | 2720 | ######## |
97 | Hitman: Agent 47 | Fox | 22467450 | 3273 | 8326530 | 3261 | ######## |
98 | Project Almanac | Par. | 22348241 | 2900 | 8310252 | 2893 | ######## |
99 | Black or White | Rela. | 21571189 | 1823 | 6213362 | 1823 | ######## |
100 | Aloha | Sony | 21067116 | 2815 | 9670235 | 2815 | ######## |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
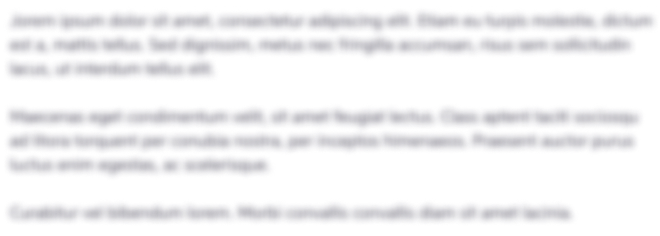
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started