Question
I need help with my homework assignment, please if anyone can help me, thanks! C++ : Write the remaining methods in the List class. Since
I need help with my homework assignment, please if anyone can help me, thanks!
C++ : Write the remaining methods in the List class. Since the List class is templated, implement its methods DIRECTLY in the "hpp" file. I have added "TODO" comments where it may not be clear you have to write additional code.
Start by implementing the ListNode constructors, the no-argument List constructor, size, and empty. If any incomplete methods result in compiler errors, write stubs that do nothing or return a default value. Test the five methods above before continuing. Then implement the size constructor and iterator methods. Test some more. Continue iterating in this fashion. Do NOT attempt to write all of the methods before testing.
// Description: List class, our implementation of a list ADT using a circular, doubly-linked list. #ifndef LIST_HPP #define LIST_HPP /************************************************************/ // System includes #include#include #include #include #include /************************************************************/ // Local includes /************************************************************/ // Using declarations using std::ostream; using std::ostream_iterator; using std::initializer_list; /************************************************************/ template struct ListNode { ListNode () : data () { // Make a circular node, with next and prev // pointing to this node // TODO } // TODO ListNode (const T& d, ListNode* n = nullptr, ListNode* p = nullptr) ... // Initialize the three data members appropriately { } T data; ListNode* next; ListNode* prev; }; /************************************************************/ template struct ListIterator { typedef ListIterator Self; typedef ListNode Node; typedef ptrdiff_t difference_type; typedef std::bidirectional_iterator_tag iterator_category; typedef T value_type; typedef T* pointer; typedef T& reference; ListIterator () : m_nodePtr () { } explicit ListIterator (Node* n) : m_nodePtr (n) { } reference operator* () const { return m_nodePtr->data; } // Return address of node's data member pointer operator-> () const { return &m_nodePtr->data; } // Pre-increment: TODO Self& operator++ () { // Make the iterator point to the next node // and return the updated iterator ... } // Post-increment Self operator++ (int) { Self temp (*this); m_nodePtr = m_nodePtr->next; return temp; } // Pre-decrement: TODO Self& operator-- () { // Make the iterator point to the prev node // and return the updated iterator ... } // Post-decrement Self operator-- (int) { Self temp (*this); m_nodePtr = m_nodePtr->prev; return temp; } bool operator== (const Self& i) const { return m_nodePtr == i.m_nodePtr; } bool operator!= (const Self& i) const { return m_nodePtr != i.m_nodePtr; } /************************************************************/ Node* m_nodePtr; }; /************************************************************/ template struct ListConstIterator { typedef ListConstIterator Self; typedef const ListNode Node; typedef ListIterator iterator; typedef ptrdiff_t difference_type; typedef std::bidirectional_iterator_tag iterator_category; typedef T value_type; typedef const T* pointer; typedef const T& reference; ListConstIterator () : m_nodePtr () { } explicit ListConstIterator (Node* n) : m_nodePtr (n) { } ListConstIterator (const iterator& i) : m_nodePtr (i.m_nodePtr) { } reference operator* () const { return m_nodePtr->data; } pointer operator-> () const { return &m_nodePtr->data; } Self& operator++ () { m_nodePtr = m_nodePtr->next; return *this; } // Post-increment: TODO Self operator++ (int) { } Self& operator-- () { m_nodePtr = m_nodePtr->prev; return *this; } // Post-decrement: TODO Self operator-- (int) { } bool operator== (const Self& i) const { return m_nodePtr == i.m_nodePtr; } bool operator!= (const Self& i) const { return m_nodePtr != i.m_nodePtr; } /************************************************************/ Node* m_nodePtr; }; template inline bool operator== (const ListIterator & i1, const ListConstIterator & i2) { // Return whether the two iterators refer to the same node // TODO } template inline bool operator!= (const ListIterator & i1, const ListConstIterator & i2) { // Return whether the two iterators refer to different nodes // TODO } /************************************************************/ template class List { typedef ListNode Node; public: typedef ListIterator iterator; typedef ListConstIterator const_iterator; typedef T value_type; typedef T* pointer; typedef T& reference; typedef const T& const_reference; // Initialize an empty list List () // Initialize data members { } // Initialize a list with the elements in "values", // in the same order List (initializer_list values) : m_header (), m_size (0) { // Add all values to the list } // Initialize a list of size "n", with each value set to "defValue" explicit List (size_t n, const T& defValue = T ()); // Copy constructor // Initialize this list to be a copy of "otherList" List (const List& otherList); // Destructor ~List () { // Deallocate all nodes // TODO } // Assign "rhs" to this object // Watch for self-assignment List& operator= (const List& rhs) { // TODO return *this; } // Return whether the list is empty bool empty () const { } // Return the size size_t size () const { } // Return the first element reference front () { } const_reference front () const { } // Return the back element reference back () { } const_reference back () const { } // Add "item" to the front of the list void push_front (const_reference item) { } // Remove the first element void pop_front () { } // Add "item" to the back of the list void push_back (const_reference item) { } // Remove the last element void pop_back () { } // Return an iterator referencing the first element iterator begin () { } const_iterator begin () const { } // Return an iterator referencing one past the last element iterator end () { } const_iterator end () const { } // Insert "item" at position "i" // Return an iterator referencing the inserted element iterator insert (iterator i, const T& item) { } // Erase element at position "i" // Return an iterator referencing the element beyond the one erased iterator erase (iterator i) { } // Erase elements in the range [i1, i2) // Return iterator "i2" iterator erase (iterator i1, iterator i2) { } private: // Dummy header Node m_header; size_t m_size; }; /************************************************************/ // Output operator // Output "list" in the format [ e1 e2 e3 ... en ] template ostream& operator<< (ostream& out, const List & list) { out << "[ "; // Print the elements ... // TODO out << ']'; return out; } /************************************************************/ #endif /************************************************************/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
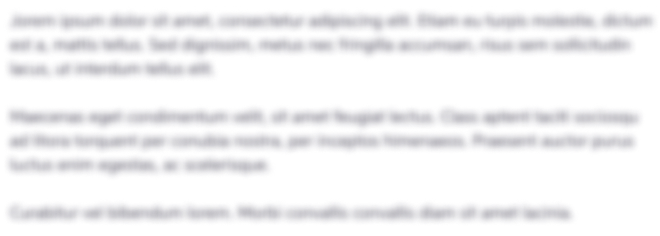
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started