Question
I need help with some errors when trying to build a program. 1>------ Build started: Project: ConsoleApplication1, Configuration: Debug Win32 ------ 1>assignment1searchandsort.cpp 1>c:userszosource eposconsoleapplication1consoleapplication1assignment1searchandsort.cpp(18): error
I need help with some errors when trying to build a program.
1>------ Build started: Project: ConsoleApplication1, Configuration: Debug Win32 ------ 1>assignment1searchandsort.cpp 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(18): error C2065: 'cout': undeclared identifier 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(19): error C2065: 'cout': undeclared identifier 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(19): error C3861: 'setw': identifier not found 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(21): error C2065: 'cout': undeclared identifier 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(21): error C3861: 'setw': identifier not found 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(23): error C2065: 'cout': undeclared identifier 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(23): error C2563: mismatch in formal parameter list 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(23): error C2568: '<<': unable to resolve function overload 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(23): note: could be 'std::basic_ostream<_Elem,_Traits> &std::endl(std::basic_ostream<_Elem,_Traits> &)' 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(89): error C2065: 'cout': undeclared identifier 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(90): error C2065: 'cin': undeclared identifier 1>c:\users\zo\source epos\consoleapplication1\consoleapplication1\assignment1searchandsort.cpp(92): error C2079: 'infile' uses undefined class 'std::basic_ifstream
This is the code:
#include
#include
#include
#include "stdafx.h"
#include
using namespace std;
void display(string message, string words[], int n);
void selectionSort(string words[], int n);
void bubbleSort(string words[], int n);
int binarySearch(string words[], int n, string search);
int linearSearch(string words[], int n, string search);
void display(string message, string words[], int n)
{
cout << message << endl;
cout << left << setw(12) << "Index" << setw(30) << "Word" << endl;
for (int i = 0; i < n; i++)
cout << left << setw(12) << i << setw(30) << words[i] << endl;
cout << endl << endl;
}
void selectionSort(string words[], int n)
{
int minIdx;
string temp;
for (int i = 0; i < n; i++)
{
for (int j = i + 1; j < n; j++)
{
if (words[j] < words[minIdx])
minIdx = j;
}
if (i != minIdx) //swap?
{
temp = words[i];
words[i] = words[minIdx];
words[minIdx] = temp;
}
}
}
void bubbleSort(string words[], int n)
{
for (int i = 0; i < n - 1; i++)
{
for (int j = 0; j < n - i - 1; j++)
{
if (words[j] > words[j + 1]) //compare 2 consecutive locations and swap if needed
{
string temp = words[j];
words[j] = words[j + 1];
words[j + 1] = temp;
}
}
}
}
int binarySearch(string words[], int n, string search)
{
int low = 0, high = n - 1;
int mid;
while (low <= high)
{
mid = (low + high) / 2;
if (words[mid] == search)
return mid;
else if (search < words[mid])
high = mid - 1;
else
low = mid + 1;
}
return -1;//when not found
}
int linearSearch(string words[], int n, string search)
{
for (int i = 0; i < n; i++)
{
if (words[i] == search)
return i;
}
return -1; //when not found
}
int main()
{
char filename[50];
cout << "Enter input filename: ";
cin >> filename;
ifstream infile(filename);
if (!infile.is_open())
{
cout << "Error, could not open: " << filename << endl;
return 1;
}
//put the strings into the array
string words[50];
int n = 0;
while (getline(infile, words[n]))
n++;
infile.close();
display("Words before sorting", words, n);
int choice;
cout << "You may enter 1 to selection sort, or 2 to bubble sort." << endl;
cout << "Enter your choice: ";
cin >> choice;
if (choice == 1)
selectionSort(words, n);
if (choice == 2)
bubbleSort(words, n);
display("Words after sorting", words, n);
string search = "";
int index;
while (search != "stop")
{
cout << "Enter a word to search(type stop to stop): ";
cin >> search;
if (search != "stop")
{
cout << "1. Linear search" << endl;
cout << "2. Binary search" << endl;
cout << "Enter your choice: ";
cin >> choice;
if (choice == 1)
index = linearSearch(words, n, search);
else
index = binarySearch(words, n, search);
if (index == -1)
cout << search << " NOT found" << endl << endl;
else
cout << search << " found at index " << index << endl << endl;
}
}
}
It will compile fine on other compilers like https://www.onlinegdb.com/online_c++_compiler.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
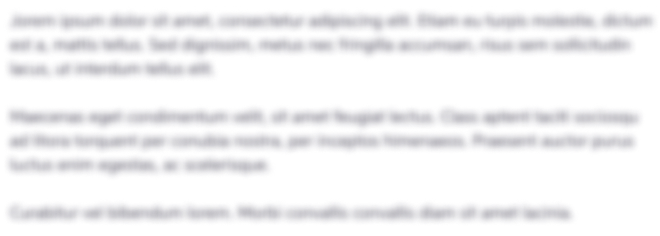
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started