Question
I need help with the C code that would do the following: if command == SPELL_CMD_CHAR This one spell checks a file numbered fileNum by:
I need help with the C code that would do the following:
if command == SPELL_CMD_CHAR
This one spell checks a file numbered fileNum by:
1. Opening it in read-write mode. If opening the file fails then it sends STD_ERROR_MSG back to the client.
2. Putting ENDING_TEXT (a purposeful misspelling) at the end of the file. We do this so we can look for it in the output of the spell-checker: when we see it we know we have seen all the output.
If fileFd is the file-descriptor of your input-output file you can do this with:
long length = lseek(fileFd,0,SEEK_END);
int status = write(fileFd,ENDING_TEXT,sizeof(ENDING_TEXT)-1);
3. Opens a pipe for the spell-checking child process to communicate back to the parent. If opening the pipe fails then it sends STD_ERROR_MSG back to the client.
4. fork()s a child process. The child process resets its position in the input-output file back to the beginning of the file. It then has its input re-directed from the file descriptor of the file, and its output re-directed to the output side of the pipe.
If fileFd is the file-descriptor of your input-output file, and fromSpeller[] is your pipe, you may do this with:
lseek(fileFd,0,SEEK_SET);
close(0);
dup(fileFd);
close(1);
dup(fromSpeller[1]);
Then, the child process executes program SPELLER_PROGNAME with command line argument SPELLER_PROG_OPTION. If the child process cannot execute the program, then it sends STD_ERROR_MSG and ENDING_TEXT back to the parent process using the pipe and does exit(EXIT_FAILURE).
5.Meanwhile, in a loop the parent process continually read()s from the input end of the pipe. Whatever it read()s it should put into a buffer which it will send back to the client. When it finds ENDING_TEXT it knows the input has ended, and it can quit the loop. Look for one string inside of another with strstr().
6. After the read()ing loop the parent process should: wait() for the child process to end remove ENDING_TEXT from the input-output file
ftruncate(fileFd,length);
close() that file and the pipe
Step by Step Solution
There are 3 Steps involved in it
Step: 1
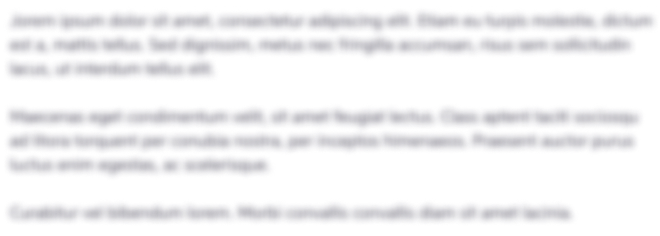
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started