Question
I need help with the following Java code. Implement all missing methods of ArrayDictionary.java. You are allowed to throw UnsupportedOperationException from remove methods of both
I need help with the following Java code.
Implement all missing methods of ArrayDictionary.java. You are allowed to throw UnsupportedOperationException from remove methods of both iterators.
/**
*An interface for a dictionary with distinct search keys.
*/
import java.util.Iterator;
public interface DictionaryInterface
{
/**
* Adds a new entry to this dictionary. If the given search key already exists
* in the dictionary, replaces the corresponding value.
*
* @param key
* An object search key of the new entry.
* @param value
* An object associated with the search key.
* @return Either null if the new entry was added to the dictionary or the
* value that was associated with key if that value was replaced.
*/
public V add(K key, V value);
/**
* Removes a specific entry from this dictionary.
*
* @param key
* An object search key of the entry to be removed.
* @return Either the value that was associated with the search key or null if
* no such object exists.
*/
public V remove(K key);
/**
* Retrieves from this dictionary the value associated with a given search key.
*
* @param key
* An object search key of the entry to be retrieved.
* @return Either the value that is associated with the search key or null if
* no such object exists.
*/
public V getValue(K key);
/**
* Sees whether a specific entry is in this dictionary.
*
* @param key
* An object search key of the desired entry.
* @return True if key is associated with an entry in the dictionary.
*/
public boolean contains(K key);
/**
* Creates an iterator that traverses all search keys in this dictionary.
*
* @return An iterator that provides sequential access to the search keys in
* the dictionary.
*/
public Iterator getKeyIterator();
/**
* Creates an iterator that traverses all values in this dictionary.
*
* @return An iterator that provides sequential access to the values in this
* dictionary.
*/
public Iterator getValueIterator();
/**
* Sees whether this dictionary is empty.
*
* @return True if the dictionary is empty.
*/
public boolean isEmpty();
/**
* Gets the size of this dictionary.
*
* @return The number of entries (key-value pairs) currently in the dictionary.
*/
public int getSize();
/** Removes all entries from this dictionary. */
public void clear();
} // end DictionaryInterface
/**
A class that implements the ADT dictionary by using a resizable array.
The dictionary is unsorted and has distinct search keys.
@author Frank M. Carrano
@author Timothy M. Henry
@version 4.0
*/
import java.util.Arrays;
import java.util.Iterator;
import java.util.NoSuchElementException;
public class ArrayDictionary implements DictionaryInterface
{
private Entry[] dictionary; // Array of unsorted entries
private int numberOfEntries;
private boolean initialized = false;
private final static int DEFAULT_CAPACITY = 25;
private static final int MAX_CAPACITY = 10000;
public ArrayDictionary()
{
this(DEFAULT_CAPACITY); // Call next constructor
} // end default constructor
public ArrayDictionary(int initialCapacity)
{
checkCapacity(initialCapacity);
// The cast is safe because the new array contains null entries
@SuppressWarnings("unchecked")
Entry[] tempDictionary = (Entry[]) new Entry[initialCapacity];
dictionary = tempDictionary;
numberOfEntries = 0;
initialized = true;
} // end constructor
public V add(K key, V value)
{
checkInitialization();
if ((key == null) || (value == null))
throw new IllegalArgumentException("Cannot add null to a dictionary.");
else
{
V result = null;
int keyIndex = locateIndex(key);
if (keyIndex < numberOfEntries)
{
// Key found, return and replace entry's value
result = dictionary[keyIndex].getValue(); // Get old value
dictionary[keyIndex].setValue(value); // Replace value
} else // Key not found; add new entry to dictionary
{
// Add at end of array
dictionary[numberOfEntries] = new Entry<>(key, value);
numberOfEntries++;
ensureCapacity(); // Ensure enough room for next add
} // end if
return result;
} // end if
} // end add
// Returns the array index of the entry that contains key, or
// returns numberOfEntries if no such entry exists.
private int locateIndex(K key)
{
// Sequential search
int index = 0;
while ((index < numberOfEntries) && !key.equals(dictionary[index].getKey()))
index++;
return index;
} // end locateIndex
public V remove(K key)
{
checkInitialization();
V result = null;
int keyIndex = locateIndex(key);
if (keyIndex < numberOfEntries)
{
// Key found; remove entry and return its value
result = dictionary[keyIndex].getValue();
dictionary[keyIndex] = dictionary[numberOfEntries - 1];
dictionary[numberOfEntries - 1] = null;
numberOfEntries--;
} // end if
// Else result is null
return result;
} // end remove
private class Entry
{
private S key;
private T value;
private Entry(S searchKey, T dataValue)
{
key = searchKey;
value = dataValue;
} // end constructor
private S getKey()
{
return key;
} // end getKey
private T getValue()
{
return value;
} // end getValue
private void setValue(T dataValue)
{
value = dataValue;
} // end setValue
} // end Entry
} // end ArrayDictionary
Step by Step Solution
There are 3 Steps involved in it
Step: 1
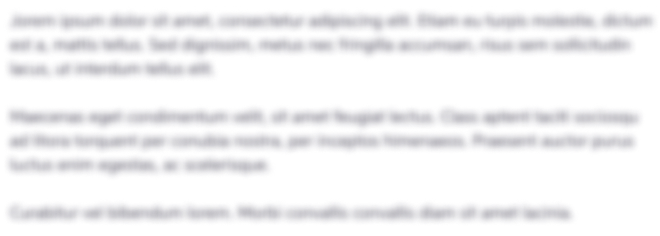
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started