Question
I need help with the following program using Java. The program is supposed to sort a list of science fair judges into a science fair
I need help with the following program using Java.
The program is supposed to sort a list of science fair judges into a science fair catogory they can judge. The list of judges has the judge's name and subject it will look like this.
Ben Miller: Biology, Chemistry
Joe Hansen: Chemistry, Earth Science
Jill Clemson: Behavioral/Social Science, Life Science, Environmental Science
Cathy Lively: Life Science
Francisco Aranda: Chemistry, Mathematics/Physics
Betty Baines: Environmental Science
Jim Green: Life Science
Bill Jones: Chemistry, Behavioral/Social Science, Earth/Space Science, Mathematics//Physics, Environmental Science
Susan Garcia: Life Science
Jamie Aguirre: Mathematics/Physics
Carla Phillips: Earth/Space Science
Tony Brown: Life Science
Joseph Martin: Life Science, Environmental Science
Chris Suarez: Environmental Science, Behavioral/Social Science
Sara Rodriguez: Chemistry
Karen Simi: Mathematics/Physics, Environmental Science
Maria Gonzales: Life Science, Chemistry
Leticia Jones: Chemistry
Jamie Garcia: Earth/Space Science, Mathematics/Physics, Chemistry
The list of projectws will have a project number followed the subject it falls into, that looks like this.
403 Chemistry
412 Chemistry
400 Behavioral/Social Science
407 Chemistry
410 Chemistry
417 Life Science
415 Environmental Science
419 Life Science
411 Chemistry
420 Mathematics/Physics
421 Mathematics/Physics
408 Chemistry
409 Chemistry
406 Chemistry
401 Behavioral/Social Science
416 Environmental Science
413 Earth/Space Science
405 Chemistry
414 Environmental Science
402 Behavioral/Social Science
418 Life Science
404 Chemistry
The progrram should get the two input files, one for the subjects th eother the judges and read them and then produce a group of the judges that will judge each project. The maximum number of judges in a group is 3 and the most projects they can judge is 6 out put can look something like this
Chemistry_1 Bill Jones, Joe Hansen, Sara Rodriguez
Projects: 403, 404, 405, 406, 407, 408
Chemistry_2 Jamie Garcia, Leticia Jones, Maria Gonzales
Projects: 409, 410, 411, 412
Here is the code I have so far.
//the main class
package build_Project;
import java.awt.event.ActionEvent;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.LinkedList;
import javax.swing.JButton;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class Processing_J extends testFrame{
public static LinkedList
public static LinkedList
public static ArrayList
public Processing_J(){
super();
inputStream("project_List.txt", "judge_List.txt");
visualLinkedLists();
printOutGroups();
}
public static void printOutGroups(){
for(int i=0; i alist.get(i).printGroup(); System.out.println(); } } // prints out 'alist' to console public static void visualArrayList(){ OutputGroup hold; String[] str; int[] num; for(int i=0; i hold = alist.get(i); str = hold.names; num = hold.projects; jta3.setText( jta3.getText()+" "+ hold.catagory+"_"+hold.group+" (" ); int k=0; while(k<6){ int n = num[k]; if(k==0){ jta3.setText( jta3.getText()+ n ); }else if(n==0){ // Do nothing }else if(n!=0){ jta3.setText( jta3.getText()+ ", "+n ); } k++; } jta3.setText( jta3.getText()+ ") Judges: "+ str[0]+","+str[1]+","+str[2]+" " ); } } // prints out 'alist' to mock GUI public static void printLinkedLists(){ System.out.println("\tPROJECT LIST"); for(String x: projectList){ System.out.println(x); } System.out.println(" \tJUDGE LIST"); for(String x: judgeList){ System.out.println(x); } } // prints out 'projectList' and 'judgeList' to console public static void visualLinkedLists(){ for(String x:projectList){ jta1.setText(jta1.getText()+x+" "); } for(String x:judgeList){ jta2.setText(jta2.getText()+x+" "); } } // prints out 'projectList' and 'judgeList' to mock GUI public static void processing() throws NullPointerException, Exception{ int projectIndex=0, grouping=1; int filled=0; String subject="", projectLine=""; while(projectIndex!=projectList.size()-1){ projectLine = projectList.get(projectIndex); if(subject.equalsIgnoreCase(projectLine.substring(4))){ //subject is same String[] hold2 = alist.get(alist.size()-1).names; int[] hold1 = alist.get(alist.size()-1).projects; filled=0; for(int n:hold1) if(n>1) filled++; if(filled!=6){ // room for more projects grouping=1; }else { // create new grouping example: "Chemestry_2" grouping++; newAListEntity(subject+"_"+grouping, Integer.parseInt(projectLine.substring(0, 3))); processingLoop(projectIndex, subject); } }else { subject=projectList.get(projectIndex).substring(4); newAListEntity(subject+"_"+grouping, Integer.parseInt(projectLine.substring(0, 3))); processingLoop(projectIndex, subject); } projectIndex++; } } // creating alist public static int processingLoop(int pI, String subject){ int projects=1; String projectLine=""; while(pI if(subject.equalsIgnoreCase(projectList.get(pI).substring(4))){ alist.get(alist.size()-1).projects[2] = Integer.parseInt(projectList.get(pI).substring(0, 3)); }else { pI--; break; } if(projects>=6) break; pI++; } return pI--; } // recursive to either end of projects with 'subject' or till there are 6 projects public static void newAListEntity(String subject, int project1){ int count=0; String judge; alist.add(new OutputGroup()); alist.get(alist.size()-1).setCategory(subject); alist.get(alist.size()-1).projects[0]=project1; for(int i=0; i judge = judgeList.get(i); if(judge.contains(subject.substring(0, subject.length()-2))&&count!=3){ alist.get(alist.size()-1).names[count] = judge.substring(0, judge.indexOf(":")); count++; judgeList.remove(i); } } // add judges here } // creates a new OutputGroup and adds to alist public static void sortProjects(){ String LineI, LineJ; for(int i=0; i for(int j=i+1; j LineI=projectList.get(i).substring(projectList.get(i).indexOf(" ")+1); LineJ=projectList.get(j).substring(projectList.get(j).indexOf(" ")+1); sortProjects2(LineI, i, LineJ, j, 0); } } }// sorts project list by taking project subjects and passes that to sortProjects2 to sort alphabetically public static void sortProjects2(String str1, int index1, String str2, int index2, int indexKey){ int num1, num2; String hold; if((indexKey num1 = convertCharToInt(str1.substring(indexKey, indexKey+1)); num2 = convertCharToInt(str2.substring(indexKey, indexKey+1)); if(num1==num2){ sortProjects2(str1, index1, str2, index2, indexKey+1); }else if(num1>num2){ hold = projectList.get(index1); projectList.set(index1, projectList.get(index2)); projectList.set(index2, hold); } } } // 2 strings are compared to find which one is alphabetically first public static int convertCharToInt(String str){ str = str.toLowerCase(); switch(str){ case "a": return 1; case "b": return 2; case "c": return 3; case "d": return 4; case "e": return 5; case "f": return 6; case "g": return 7; case "h": return 8; case "i": return 9; case "j": return 10; case "k": return 11; case "l": return 12; case "m": return 13; case "n": return 14; case "o": return 15; case "p": return 16; case "q": return 17; case "r": return 18; case "s": return 19; case "t": return 20; case "u": return 21; case "v": return 22; case "w": return 23; case "x": return 24; case "y": return 25; case "z": return 26; default: System.exit(0); } return 0; } // converts a letter to a number ('a'-'z') => (1-26) public static void sortJudge(){ String hold; for(int i=0; i for(int j=i; j if(judgeList.get(i).split(",").length>judgeList.get(j).split(",").length){ hold = judgeList.get(i); judgeList.set(i, judgeList.get(j)); judgeList.set(j, hold); hold = null; } } } } // Swap Sorts judgeList to be in order by which teacher can cover the least areas of science to the most public static void outputStream(){ try { PrintWriter outputStream = new PrintWriter(new FileOutputStream("OutputGroup.txt")); int projects[]; String[] judgeNames; for(OutputGroup grp: alist){ judgeNames = grp.names; projects = grp.projects; outputStream.println( grp.catagory+"_"+grp.group+": ("+ grp.printProjects()+") "+ grp.printJudges()+" " ); } outputStream.close(); } catch(IOException e){ System.out.println("Error opening the file"); System.exit(0); } catch(Exception e){ JOptionPane.showMessageDialog( null, "Error occured "+e.getMessage(), "ERROR!", JOptionPane.ERROR_MESSAGE); } }// Saves current linkList to the file specified in openStream public static void inputStream(String projectFile, String judgeFile){ try { BufferedReader inputStream = new BufferedReader(new FileReader(projectFile)); BufferedReader inputStream2 = new BufferedReader(new FileReader(judgeFile)); String line = inputStream.readLine(); String line2 = inputStream2.readLine(); while(line!=null||line2!=null){ if(line!=null){ projectList.add(line); line=inputStream.readLine(); } if(line2!=null){ judgeList.add(line2); line2=inputStream2.readLine(); } } inputStream.close(); inputStream2.close(); } catch(FileNotFoundException e){ JOptionPane.showMessageDialog( null, "File was not found or could not be opened "+ e.getMessage(), "ERROR!", JOptionPane.ERROR_MESSAGE); } catch(IOException e){ JOptionPane.showMessageDialog( null, "Error occured "+e.getMessage(), "ERROR!", JOptionPane.ERROR_MESSAGE); } sortProjects(); sortJudge(); }// takes in which file should be opened then runs imidiat sorting public static void main(String[] args){ @SuppressWarnings("unused") Processing_J run = new Processing_J(); } public void actionPerformed(ActionEvent e) { Object source = e.getSource(); String name = ((JButton)source).getName(); if(name.equals("Card 1 South Panel Next Button")){ try{ processing(); } catch(NullPointerException Error){ System.out.println("Null Pointer Error"); } catch(Exception Error){ System.out.println("Error: "+Error.getMessage()); } visualArrayList(); printOutGroups(); outputStream(); }else if(name.equals("Card 2 South Panel Next Button")){ visualLinkedLists(); } cl.next( ((JPanel) // "Main Panel Content Pane" ((JPanel) // "Card Panel #" ((JPanel) // "Card # South Panel" ((JButton) // "Card # South Panel Next Button" source).getParent()).getParent()).getParent()) ); } } The following is the output group package build_Project; public class OutputGroup { public int catNum; // unknown public int group; // chemestry_1, chemestry_2, Group = 1, 2 public String catagory; // catagory = {Chemistry, Behavioral/Social Science, Life Science, ...} public String[] names; // judge names public int[] projects; // project numbers public int count; // unknown public OutputGroup(){ names = new String[3]; projects = new int[6]; for(int i=0;i } public void printGroup1(){ System.out.print(catagory+"_"+group+" "); for(String x: names) if(x!=null) System.out.print(x+", "); System.out.println(); System.out.print("Projects: "); for(int x: projects) if(x>1) System.out.print(x+", "); System.out.println(); } public void printGroup(){ int num; String str; System.out.print(catagory+"_"+group+" ("); for(int i=0; i if(i!=0){ if(projects[i]!=0){ System.out.print(", "+projects[i]); } }else{ System.out.print(projects[i]); } } System.out.print(") "); System.out.print("Judges: "); for(int i=0; i if(i!=0){ if(names[i]!=null){ System.out.print(", "+names[i]); } }else{ System.out.print(names[i]); } } System.out.println(); } public void setCategory(String cat){ String[] x = cat.split("_"); catagory=x[0]; group=Integer.parseInt(x[1]); } public String printProjects(){ String str=""; for(int i=0; i<6; i++){ if(projects[i]!=0){ str+=str+projects[i]+", "; }else if(i==projects.length-1){ str+=str+projects[i]; } } return str; } public String printJudges(){ String str=""; for(int i=0; i<3; i++){ if(names[i]!=null){ str+=str+names[i]+", "; }else if(i==names.length-1){ str+=str+names[i]; } } return str; } } The following is the testFrame package build_Project; import java.awt.BorderLayout; import java.awt.CardLayout; import java.awt.Container; import java.awt.FlowLayout; import java.awt.GridBagConstraints; import java.awt.GridBagLayout; import java.awt.Insets; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JTextArea; public class testFrame extends JFrame implements ActionListener{ public static final int WIDTH = 1100; public static final int HEIGHT = 800; public static JTextArea jta1; public static JTextArea jta2; public static JTextArea jta3; public CardLayout cl = new CardLayout(); public testFrame() { setTitle("Testing file processing"); setSize(WIDTH, HEIGHT); setLocation(200, 100); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setName("Main JFrame"); Container C = getContentPane(); makeWindow(C); setVisible(true); } public void makeWindow(Container c){ c.setName("Main Window Content Pane"); c.setLayout(cl); c.add(buildCard1(), "Card 1"); c.add(buildCard2(), "Card 2"); } public JPanel buildCard1(){ JPanel card1 = new JPanel(new BorderLayout()); card1.setName("Card Panel 1"); card1.add(buildCenterBox1(), BorderLayout.CENTER); card1.add(buildSouthBox1(), BorderLayout.SOUTH); return card1; } public JPanel buildCenterBox1(){ JPanel cBox1 = new JPanel(new GridBagLayout()); cBox1.setName("Card 1 Center Panel"); GridBagConstraints gl = new GridBagConstraints(); gl.weightx = 1; gl.weighty = 1; gl.gridheight = 2; gl.insets = new Insets(5, 5, 5, 5); JPanel textPanel1 = new JPanel(new FlowLayout(FlowLayout.CENTER)); textPanel1.setName("Card 1 Center Panel Text Panel 1"); jta1 = new JTextArea(30, 25); jta1.setName("Card 1 Center Text Panel 1 TextArea1"); jta1.setEditable(false); textPanel1.add(jta1); gl.gridx = 0; gl.gridy = 0; cBox1.add(textPanel1, gl); JPanel textPanel2 = new JPanel(new FlowLayout(FlowLayout.CENTER)); textPanel2.setName("Card 1 Center Panel Text Panel 2"); jta2 = new JTextArea(30, 25); jta2.setName("Card 1 Center Text Panel 1 TextArea2"); jta2.setEditable(false); textPanel1.add(jta2); gl.gridx = 1; gl.gridy = 0; cBox1.add(textPanel2, gl); return cBox1; } public JPanel buildSouthBox1(){ JPanel sBox1 = new JPanel(new FlowLayout(FlowLayout.CENTER)); sBox1.setName("Card 1 South Panel"); JButton nextBtn = new JButton("Next"); nextBtn.setName("Card 1 South Panel Next Button"); nextBtn.addActionListener(this); sBox1.add(nextBtn); return sBox1; } public JPanel buildCard2(){ JPanel card2 = new JPanel(new BorderLayout()); card2.setName("Card Panel 2"); card2.add(buildCenterBox2(), BorderLayout.CENTER); card2.add(buildSouthBox2(), BorderLayout.SOUTH); return card2; } public JPanel buildCenterBox2(){ JPanel cBox2 = new JPanel(); cBox2.setName("Card 2 Center Panel"); jta3 = new JTextArea(30, 25); jta3.setName("Card 1 Center Text Panel 1 TextArea1"); jta3.setEditable(false); cBox2.add(jta3); return cBox2; } public JPanel buildSouthBox2(){ JPanel sBox2 = new JPanel(new FlowLayout(FlowLayout.CENTER)); sBox2.setName("Card 2 South Panel"); JButton nextBtn = new JButton("Next"); nextBtn.setName("Card 2 South Panel Next Button"); nextBtn.addActionListener(this); sBox2.add(nextBtn); return sBox2; } public void actionPerformed(ActionEvent e) { } } As it stands the sorting is not working properly. Please help, thanks!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
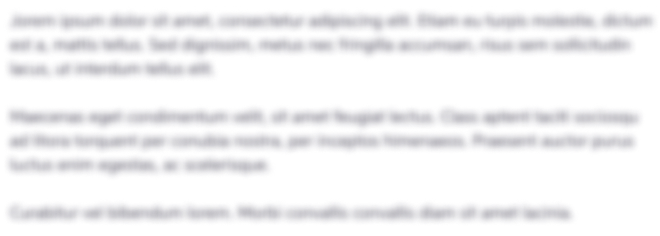
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started