Question
I need help with the get quote sql that is all Build a rate quote GUI. The system will first allow us to select a
I need help with the get quote sql that is all
Build a rate quote GUI. The system will first allow us to select a customer (assume they are already in the DB), an employee giving the quote, and then select part(s) and/or motorcycles. You system should also include the shipping information in the quote. After all of the items have been selected for the rate quote, we should give the user a bottom line total that includes shipping (we wont worry about sales tax in this system). We d like a hard copy of this quote for future reference.
Therefore, you should format the output neatly in System.out.println area for ease of reference. The output should include the current date, customer, employee and product information as well as the computed total for this quote.
Here what I have so far and comments i got if you're just getting the quote for this scenario, you are going to simply code independent SELECT statements for each table. One for Customer, one for Employee, one for Parts, etc.
import java.awt.*; import java.awt.event.*; import javax.swing.*;
public class ProjectPhase2 extends JFrame { JPanel jp = new JPanel(); JLabel customerLabel = new JLabel("Customer"); JTextField cusField = new JTextField(30); JLabel employeeLabel = new JLabel("Employee"); JTextField empField = new JTextField(30); JLabel partLabel = new JLabel("Part"); JTextField partField = new JTextField(30); JLabel motorLabel = new JLabel("Motor"); JTextField motorField = new JTextField(30); JButton jb = new JButton("Get Quote"); JTextArea textArea = new JTextArea( "This is an editable JTextArea. " );
public ProjectPhase2() { setTitle("Phase2 Get Quote"); setVisible(true); setSize(600, 600); setDefaultCloseOperation(EXIT_ON_CLOSE); GridLayout experimentLayout = new GridLayout(5,2); jp.setLayout(experimentLayout); jp.add(cusField); jp.add(customerLabel); jp.add(empField); jp.add(employeeLabel); jp.add(partField); jp.add(partLabel); jp.add(motorField); jp.add(motorLabel);
jp.add(jb); jb.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { // String input = cusField.getText(); // System.out.println(input); /* Statement stmt = connection.createStatement(); ResultSet cusSet = stmt.executeQuery ("select name, address, city from student where advisor " + " = 'Trono'"); ResultSet empSet = stmt.executeQuery ("select name, address, city from student where advisor " + " = 'Trono'"); // Iterate through the result and print the student names while (rset.next()) System.out.println(rset.getString(1) + " " + rset.getString(2) + ". " + rset.getString(3)); */ String inputPart = partField.getText(); String inputMotor = motorField.getText(); if (inputPart.trim().equals("")){ System.out.println("parts was blank");} else{ System.out.println(inputPart); } if (inputMotor.trim().equals("")) System.out.println("motor was blank"); else System.out.println(inputMotor); } }); textArea.setLineWrap(true); // jp.add(textArea); add(jp);
}
public static void main(String[] args) { // Class.forName("com.mysql.jdbc.Driver"); // System.out.println("Driver loaded"); // Connection connection = DriverManager.getConnection //("jdbc:mysql://localhost/sampdb", "root","cs304"); // System.out.println("Database connected"); ProjectPhase2 t = new ProjectPhase2(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
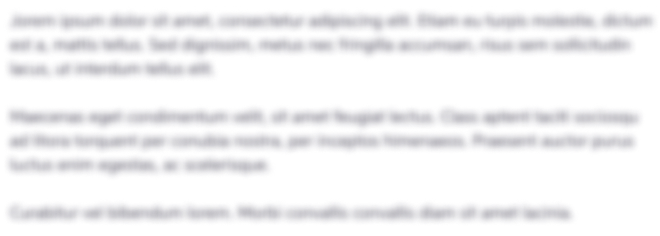
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started