Question
I need help with the TO-DO sections(C++): mediaList.hpp #pragma once // include guard #include #include // size_t #include #include #include #include // domain_error, length_error #include
I need help with the "TO-DO" sections(C++):
mediaList.hpp
#pragma once // include guard
#include
#include // size_t
#include
#include
#include
#include // domain_error, length_error
#include
#include
#include
#include "MediaItem.hpp"
class MediaList
{
// Insertion and Extraction Operators
friend std::ostream & operator
friend std::istream & operator>>( std::istream & stream, MediaList & mediaList );
// Relational Operators
friend bool operator==( const MediaList & lhs, const MediaList & rhs );
friend bool operator
public:
// Types and Exceptions
enum class Position {TOP, BOTTOM};
struct InvalidInternalState_Ex : std::domain_error { using domain_error::domain_error; }; // Thrown if internal data structures become inconsistent with each other
struct CapacityExceeded_Ex : std::length_error { using length_error::length_error; }; // Thrown if more items are inserted than will fit
struct InvalidOffset_Ex : std::logic_error { using logic_error ::logic_error; }; // Thrown of inserting beyond current size
// Constructors, destructor, and assignment operators
MediaList(); // construct an empty media list
MediaList( const MediaList & other ); // construct a media list as a copy of another media list
MediaList( MediaList && other ); // construct a media list by taking the contents of another media list
MediaList & operator=( MediaList rhs ); // intentionally passed by value and not const ref
MediaList & operator=( MediaList && rhs );
MediaList ( const std::initializer_list & initList ); // constructs a media list from a braced list of media items
MediaList & operator+=( const std::initializer_list & rhs ); // concatenates a braced list of media items to this list
MediaList & operator+=( const MediaList & rhs ); // concatenates the rhs list to the end of this list
~MediaList();
// Queries
std::size_t size() const;
std::size_t find( const MediaItem & item ) const; // returns the (zero-based) offset from top of list
// returns the (zero-based) position of the item, size() if item not found
// Mutators
void insert( const MediaItem & item, Position position = Position::TOP ); // add the media item to the top (beginning) of the media list
void insert( const MediaItem & item, std::size_t offsetFromTop ); // inserts before the existing item currently at that offset
void remove( const MediaItem & item ); // no change occurs if item not found
void remove( std::size_t offsetFromTop ); // no change occurs if (zero-based) offsetFromTop >= size()
void moveToTop( const MediaItem & item );
void swap( MediaList & rhs ) noexcept; // exchange one media list with another
private:
// Helper functions
bool containersAreConsistant() const;
std::size_t media_sl_list_size() const; // std::forward_list doesn't maintain size, so calculate it on demand
// Instance Attributes
std::size_t _media_array_size = 0; // std::array's size is constant so manage that attributes ourself
std::array _media_array;
std::vector _media_vector;
std::list _media_dl_list;
std::forward_list _media_sl_list;
};
// Relational Operators
bool operator==( const MediaList & lhs, const MediaList & rhs );
bool operator!=( const MediaList & lhs, const MediaList & rhs );
bool operator
bool operator
bool operator> ( const MediaList & lhs, const MediaList & rhs );
bool operator>=( const MediaList & lhs, const MediaList & rhs );
MediaItem.hpp
#pragma once // include guard
#include
#include
class MediaItem
{
// Insertion and Extraction Operators
friend std::ostream & operator
friend std::istream & operator>>( std::istream & stream, MediaItem & mediaItem );
// Relational Operators
friend bool operator==( const MediaItem & lhs, const MediaItem & rhs );
friend bool operator
public:
// Constructors
MediaItem() = default;
MediaItem( const std::string & movieTitle,
const std::string & director = {},
const std::string & studioName = {},
int runTime = 0 );
// Queries
std::string movieTitle () const;
std::string director () const;
std::string studioName () const;
int runTime () const;
// Mutators
void movieTitle ( const std::string & movieTitle );
void director ( const std::string & director );
void studioName ( const std::string & studioName );
void runTime ( int runTime );
private:
std::string _movieTitle;
std::string _director;
std::string _studioName;
int _runTime = 0;
};
// Relational Operators
bool operator==( const MediaItem & lhs, const MediaItem & rhs );
bool operator!=( const MediaItem & lhs, const MediaItem & rhs );
bool operator
bool operator
bool operator> ( const MediaItem & lhs, const MediaItem & rhs );
bool operator>=( const MediaItem & lhs, const MediaItem & rhs );
> to-da Aa Ab! .* 3 of 4 /************************************************************************ ** Mutators *********************************************************** *****/ void MediaList::insert( const MediaItem & item, Position position ) { // Convert the TOP and BOTTOM enumerations to an offset and delegate the work if (position =- Position:: TOP ) insert( item, else if( position == Position:: BOTTOM) insert( item, size() ); else throw std:: Logic_error( "Unexpected insertion position" exception_location ); // Programmer error. // Programmer error. Should never hit this! } void MediaList::insert( const MediaItem & item, std::size_t offsetFromTop ) // insert new item at offsetFromTop, which places it before the current item at offsetFromTop // validate offset parameter before attempting the insertion. std::size_t is an unsigned type, so no need to check for negative // offsets, and an offset equal to the size of the list says to insert at the end (bottom) of the list. Anything greater than the // current size is an error. if( offsetFromTop > size() } throw Invalidoffset Ex{ "Insertion position beyond end of current list size" exception_location); /********** Prevent duplicate entries ***********************/ //////// TO-DO ///////// 1 silently discard duplicate items from getting added to the media list. If the to-be-inserted item is already in the list, /// simply return. // Inserting into the media list means you insert the media item into each of the containers (array, vector, list, and // forward_list). Because the data structure concept is different for each container, the way an item gets inserted is a little // different for each. You are to insert the media item into each container such that the ordering of all the containers is the // same. A check is made at the end of this function to verify the contents of all four containers are indeed the same. Insert into array ***** *******/ /*********** { //////// TO-DO ///////// ///// /// Unlike the other containers, std::array has no insert() function, so you have to write it yourself. Insert into the array /// by shifting all the items at and after the insertion point (offestFrom Top) to the right opening a gap in the array that /// can be populated with the given media item. Remember that arrays have fixed capacity and cannot grow, so make sure /// there is room in the array for another item before you start by verifying _media_array_size is less than /// _media_array.size(). If not, throw CapacityExceeded_ex. Also remember that you must keep track of the number of /// valid media items in your array, so don't forget to adjust _media_array_size. } > to-da Aa Ab! .* 3 of 4 /************************************************************************ ** Mutators *********************************************************** *****/ void MediaList::insert( const MediaItem & item, Position position ) { // Convert the TOP and BOTTOM enumerations to an offset and delegate the work if (position =- Position:: TOP ) insert( item, else if( position == Position:: BOTTOM) insert( item, size() ); else throw std:: Logic_error( "Unexpected insertion position" exception_location ); // Programmer error. // Programmer error. Should never hit this! } void MediaList::insert( const MediaItem & item, std::size_t offsetFromTop ) // insert new item at offsetFromTop, which places it before the current item at offsetFromTop // validate offset parameter before attempting the insertion. std::size_t is an unsigned type, so no need to check for negative // offsets, and an offset equal to the size of the list says to insert at the end (bottom) of the list. Anything greater than the // current size is an error. if( offsetFromTop > size() } throw Invalidoffset Ex{ "Insertion position beyond end of current list size" exception_location); /********** Prevent duplicate entries ***********************/ //////// TO-DO ///////// 1 silently discard duplicate items from getting added to the media list. If the to-be-inserted item is already in the list, /// simply return. // Inserting into the media list means you insert the media item into each of the containers (array, vector, list, and // forward_list). Because the data structure concept is different for each container, the way an item gets inserted is a little // different for each. You are to insert the media item into each container such that the ordering of all the containers is the // same. A check is made at the end of this function to verify the contents of all four containers are indeed the same. Insert into array ***** *******/ /*********** { //////// TO-DO ///////// ///// /// Unlike the other containers, std::array has no insert() function, so you have to write it yourself. Insert into the array /// by shifting all the items at and after the insertion point (offestFrom Top) to the right opening a gap in the array that /// can be populated with the given media item. Remember that arrays have fixed capacity and cannot grow, so make sure /// there is room in the array for another item before you start by verifying _media_array_size is less than /// _media_array.size(). If not, throw CapacityExceeded_ex. Also remember that you must keep track of the number of /// valid media items in your array, so don't forget to adjust _media_array_size. }Step by Step Solution
There are 3 Steps involved in it
Step: 1
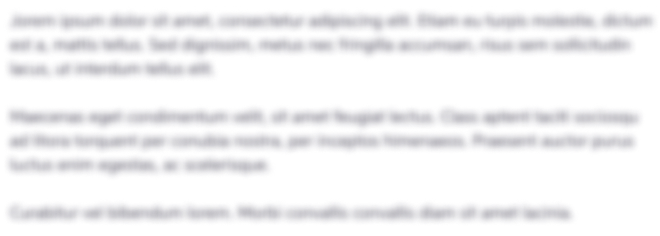
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started