Question
I need help with this ASAP!!!!!!!! For this assignment you will add a copy constructor and the following overloaded operators to your MyVector class:
I need help with this ASAP!!!!!!!!
For this assignment you will add a copy constructor and the following overloaded operators to your MyVector class: <, ==, =, +, <<, [], and ++.
Here's a description of how each operator should behave:
operator< : returns true if the length of the left-hand side MyVector is less than the length of the right-hand side MyVector, false otherwise.
operator== : returns true if both MyVectors contain the exact same values, false otherwise.
operator= : overwrites the contents of the left-hand side MyVector with the contents of the right-hand side MyVector.
operator+ : returns a MyVector object that contains an element-by-element sum of the two operators. Consider the following snippet:
MyVector m, n;
cout << m + n;
If m contains the values 1, 2, 3 and n contains the values 4, 5, then the output would be:
[5, 7, 3].
operator<< : sends a string representation of the MyVector object to the output stream. For example, if the MyVector contains 8 6 7, then operator<< would return [8, 6, 7] so that if you had a statement like
MyVector m; ... cout << m;
You would see
[8, 6, 7]
on the screen.
operator++: post-fix version. increments each element by 1. Returns a MyVector object.
operator[]: allows for retrieving and changing elements of the MyVector.
class MyVector {
private:
int* list;
int max_size;
int list_size;
public:
// Constructor
MyVector() {
max_size = 10;
list_size = 0;
list = new int[max_size];
}
// Destructor
~MyVector() {
delete[]list;
}
void push_back(int i);
void pop_back();
int& at(int i);
void clear();
int size();
};
--------------------------------------------------
Test your class using the attached test driver.
#include "MyVector.h" #include using namespace std; #include int main() { MyVector m; for(int i = 0; i < 5; i++) m.push_back(1 + rand() % 100); cout << m.size() << endl; cout << m << endl; MyVector n(m); cout << n << endl; n++; cout << n << endl; MyVector o; o = m + n; cout << o << endl; cout << (o < m) << endl; cout << (n == m) << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
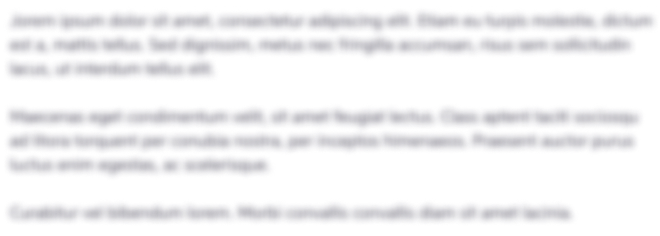
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started