Question
I need help with this assignment. It's urgent. methods to be completed are 1) Update Open Spaces 2) Add Random Tile 3) SwipeLeft 4) MergeLeft
I need help with this assignment. It's urgent. methods to be completed are 1) Update Open Spaces 2) Add Random Tile
3) SwipeLeft
4) MergeLeft
5) Transpose
6) Flip Rows
7) Make Move.
The code for Board.java is given below
package game;
import java.util.ArrayList;
/** * 2048 Board * Methods to complete: * updateOpenSpaces(), addRandomTile(), swipeLeft(), mergeLeft(), * transpose(), flipRows(), makeMove(char letter) * **/ public class Board { private int[][] gameBoard; // the game board array private ArrayList
/** * Zero-argument Constructor: initializes a 4x4 game board. **/ public Board() { gameBoard = new int[4][4]; openSpaces = new ArrayList(); }
/** * One-argument Constructor: initializes a game board based on a given array. * * @param board the board array with values to be passed through **/ public Board ( int[][] board ) { gameBoard = new int[board.length][board[0].length]; for ( int r = 0; r (); }
/** * 1. Initializes the instance variable openSpaces (open board spaces) with an empty array. * 2. Adds open spots to openSpaces (the ArrayList of open BoardSpots). * * Note: A spot (i, j) is open when gameBoard[i][j] = 0. * * Assume that gameBoard has been initialized. **/ public void updateOpenSpaces() { // WRITE YOUR CODE HERE
}
}
/** * Adds a random tile to an open spot with a 90% chance of a 2 value and a 10% chance of a 4 value. * Requires separate uses of StdRandom.uniform() to find a random open space and determine probability of a 4 or 2 tile. * * 1. Select a tile t by picking a random open space from openSpaces * 2. Pick a value v by picking a double from 0 to 1 (not inclusive of 1); public void addRandomTile() { // WRITE YOUR CODE HERE }
/** * Swipes the entire board left, shifting all nonzero tiles as far left as possible. * Maintains the same number and order of tiles. * After swiping left, no zero tiles should be in between nonzero tiles. * (ex: 0 4 0 4 becomes 4 4 0 0). **/ public void swipeLeft() { // WRITE YOUR CODE HERE }
/** * Find and merge all identical left pairs in the board. Ex: "2 2 2 2" will become "2 0 2 0". * The leftmost value takes on double its own value, and the rightmost empties and becomes 0. **/ public void mergeLeft() { // WRITE YOUR CODE HERE }
/** * Rotates 90 degrees clockwise by taking the transpose of the board and then reversing rows. * (complete transpose and flipRows). * Provided method. Do not edit. **/ public void rotateBoard() { transpose(); flipRows(); }
/** * Updates the instance variable gameBoard to be its transpose. * Transposing flips the board along its main diagonal (top left to bottom right). * * To transpose the gameBoard interchange rows and columns. * Col 1 becomes Row 1, Col 2 becomes Row 2, etc. * **/ public void transpose() { // WRITE YOUR CODE HERE }
/** * Updates the instance variable gameBoard to reverse its rows. * * Reverses all rows. Columns 1, 2, 3, and 4 become 4, 3, 2, and 1. * **/ public void flipRows() { // WRITE YOUR CODE HERE }
/** * Calls previous methods to make right, left, up and down moves. * Swipe, merge neighbors, and swipe. Rotate to achieve this goal as needed. * * @param letter the first letter of the action to take, either 'L' for left, 'U' for up, 'R' for right, or 'D' for down * NOTE: if "letter" is not one of the above characters, do nothing. **/ public void makeMove(char letter) { // WRITE YOUR CODE HERE }
/** * Returns true when the game is lost and no empty spaces are available. Ignored * when testing methods in isolation. * * @return the status of the game -- lost or not lost **/ public boolean isGameLost() { return openSpaces.size() == 0; }
/** * Shows a final score when the game is lost. Do not edit. **/ public int showScore() { int score = 0; for ( int r = 0; r
/** * Prints the board as integer values in the text window. Do not edit. **/ public void print() { for ( int r = 0; r
/** * Seed Constructor: Allows students to set seeds to debug random tile cases. * * @param seed the long seed value **/ public Board(long seed) { StdRandom.setSeed(seed); gameBoard = new int[4][4]; }
/** * Gets the open board spaces. * * @return the ArrayList of BoardSpots containing open spaces **/ public ArrayList
/** * Gets the board 2D array values. * * @return the 2D array game board **/ public int[][] getBoard() { return gameBoard; } }
Overview of files provided We provide two drivers (text and graphic) to help you test the individual methods. We suggest that you start with the text driver but once you have a good understanding of the assignment then you may use the graphic driver. - Board: The Board class contains all methods needed to construct a functioning 2048 game. Edit the empty methods with you solution, but DO NOT edit the provided ones or the methods signatures of any method. This is the file you submit. - BoardSpot: An object used to represent a location on the board. It houses a row and a column, along with getters and setters. Don't edit or submit to Autolab. - TextDriver: A tool to test your 2048 board interactively using only text-based boards. This driver is equivalent to AnimatedDriver and functions in the same way - you are free to use this driver to test all of your methods. Feel free to edit this class, as it is provided only to help you test. It is not submitted and/or graded. - To use this driver, pick whether you want to test individual methods or play the full game (this option requires all methods to be completed) by selecting the number that appears for each option. - To test individual methods, first type in the full file name for an input file. Then, select a method to test by typing in the number that appears for each method in the list. Additionally, for makeMove, moves are represented by using the WASD keys like arrow keys (w is up, a is left, s is down, and d is right). Type in the letter corresponding to the move you want to test. - Playing the full game makes use of the WASD keys like arrow keys (W is up, A is left, S is down, and D is right). Press "Q" to quit. - AnimatedDriver: A tool to test your 2048 board interactively through rendered images. This driver is equivalent to TextDriver and functions in the same way - you are free to use this driver to test all of your methods. Feel free to edit this class, as it is provided only to help you test. It is not submitted and/or graded. - To use this driver, pick whether you want to test individual methods or play the full game (this option requires all methods to be completed) by selecting the number that appears for each option. - To test individual methods, first type in the full file name for an input file. Then, select a method to test by typing in the number that appears for each method in the list. You will then see the board stored in the input file. For all methods besides makeMove, press any key to test your method. Press any key again to exit testing that method. - For makeMove, moves are represented by using the WASD keys like arrow keys (w is up, a is left, s is down, and d is right). Type in the letter corresponding to the move you want to test when you see the board stored in the input file. Once you type that letter, the move you want to test will be made. Press any key afterward to exit testing that method. - Playing the full game makes use of the WASD keys like arrow keys ( w is up, a is left, s is down, and d is right). Press " q " to quit. - Stdln and StdOut: libraries to handle input and output. Do not edit these classes. - StdRandom: Provides methods for generating random numbers. Don't edit or submit to Autolab. - Collage, Picture, StdDraw: Helper libraries to support AnimatedDriver. Don't edit or submit to Autolab. - Input Files: Preset boards you're able to use to test your 2048 board in the drivers (intput1.in, input2.in,...). You can use all input files to test all methods. Each input file contains 4 lines, each with 4 space separated numbers. Each number is either 0 (represents an empty space) or a power of 2 Board.java - DO NOT add new import statements. - DO NOT change any of the method's signatures. Methods to be implemented by you: 1. updateOpenSpaces - This method adds a BoardSpot to the openspaces array for every board open spot. A spot (i,j) is open when gameBoard [i][j]=0. - Initialize a new ArrayList of BoardSpot objects in openspaces. - Add to openSpaces all pairs (row, column) where gameBoard [ row] [ column] is 0 (ie. the tile is empty). Use BoardSpot objects to represent these pairs. - Submit Board.java with this method completed under Early Submission to receive extra credit. Note: DO NOT call update0penSpaces in any method. The driver/Autolab will call update0penSpaces and addRandomTile before making a move. Here is an example of testing this method using input1.in in both drivers. In TextDriver, open spaces in the openspaces Arraylist are denoted by two asterisks **. Note: update0penspaces must be completed before starting this method. This method adds a random tile into the board, following these rules: - First, pick a random BoardSpot from the openSpaces ArrayList (test cases are guaranteed to always have an open space). Use StdRandom. uniform(int a, int b) to generate a random integer between a lower bound "a" up to (but not including) an upper bound "b". - To randomly pick a board spot the lower bound is 0 and the upper bound is the number of items in the ArrayList. - Next, assign a value to the tile. There is a 90% chance that a 2 tile will be inserted and a 10% chance that a 4 tile will be inserted. Use StdRandom.uniform (double a, double b) to generate a random double between a lower bound "a" up to (but not including) an upper bound "b." - To randomly pick a number between 0 and 1 the lower bound is 0 and the upper bound is 1 . - If this value is less than, but not equal to, 10 percent (0.1), the tile will have a 4 value. Otherwise, the tile will have a 2 value. - Be sure to update the correct tile in the gameBoard array (as defined by the BoardSpot's row and column values) with the proper value. - Note: On the driver updateOpenSpaces() is called before this method to ensure that openSpaces is up to date. You do not need to remove the board spot from the openSpaces ArrayList! - Note: On the driver, the seed is set so you will get the same outputs every time when testing individual methods. Autolab uses the same seed as the driver to test this method. However, when playing the full game, the driver will not use seed values - it will use random values to generate different boards and tiles. Here is an example of testing this method using input1.in in both drivers. 3. swipeLeft Move all tiles as far left as possible while maintaining the same order and number of tiles. - There should be no empty spaces to the left of any tile. - Remember that 0 (gameBoard [ row [ col ]=0 ) represents an empty space. - Watch this video to learn more about this method. Here is an example of testing this method using input1.in in both drivers. 4. mergeLeft Merge all identical nonzero, horizontal pairs in the board. - The leftmost neighbor (ex. The first 2 in "0 220 ") will double its value, while the rightmost neighbor (ex. The second 2 in "0 22 0") will become 0. The method will turn this row into "0 400 ". - Only pairs will merge. 3-tile neighbors and 4-tile neighbors will not merge to become one tile. For example, "2 22 0" becomes "4 02 0" and " 222 2" becomes "4 040 0". - Watch this video to learn more about this method. Here is an example of testing this method using input1.in in both drivers. The next two methods (transpose and flipRows) can be applied in sequence to rotate the board 90 degrees clockwise. This will allow you to reuse your existing code for swipeLeft and mergeLeft for any of the four directions. For example, we can swipe right by rotating the board twice, calling swipeLeft, then rotating the board twice to return it to its original orientation. The provided - Watch this video to learn more about the intuition behind these methods. 5. transpose Interchange the rows and columns of the board. In other words, row 1 should become column 1 , row 2 becomes column 2 , and so on. More formally, gameBoard [i][j] becomes gameBoard [j][i] for all 0Step by Step Solution
There are 3 Steps involved in it
Step: 1
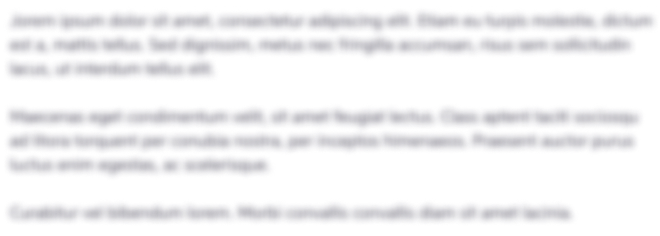
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started