Question
I need help with this C program. 1. I need to create a phonebook.c program that should read the addresses.csv CSV
I need help with this C program.
1. I need to create a "phonebook.c" program that should read the "addresses.csv" CSV file when the prgogram is ran the first time (the file contains people's first and last names, phone number and address)
a. also, any other time the program starts, it should not read from this file, rather from a binary file.
b. the program should store each person into a linked list (linkedList.c) of structures that hold the information given in the file.
2. Once it is done then, the program should sort the list by last name. If there are multiple last names then the program should then sort by first names (example, "Smith, Amy -then- "Smith, Sam")
3. Then the program should print a menu to the user with the following options:
a. Print the Phone List
b. Add a Person
c. Delete a Person
d. Find a Person
e. Save and Quit
the program should loop to take the user input until the person types e- Save and Quit
4. The options
a. Print the Phone List
prints the entire list to standard output
b. Add a Person
Prompts the user for the: last name, first name, phone number, and address
and Adds the person to the linked list in the correct location
c. Delete a Person
Asks the user for the last and first name.
If they exist: deletes the entry and prints a message stating the person was removed
If they dont exist: prints an error message
d. Find a Person
Asks the user for the last name only
Prints the matching people to the screen if they are found in the book
Prints a message saying could not find that person if no person in the book exists
e. Save And Quit
Saves the entire linked list to a binary file called phonedatabase.bin
5. Subsequent Program Starts Any other time you start the program, you should read the binary file phonedatabase.bin that was last saved to restore your phonebook.
6. Your program should then continue from step 4 displaying a menu of options to the user.
This is the addresses.csv (CVS file)
This is what I have so far for the phonebook.c (still needs some adjustments but file function code (from last assignment) seems to be similar to what I need in order to accomplish getting into file, reading and closing and I hope it helps).
#include
#include
#include
#define MAX_FIRST_PERSON_NAME 30
#define MAX_LAST_PERSON_NAME 30
#define MAX_ADDRESS 50
#define MAX_PHONE_NUMBER 20
char readAddresses(char * filename, Person names[], Person lastnames[], Person address[], Person phone[])
{
FILE * rfp; // this is our object to read data
char line[MAX_PERSON_NAME]; // Just make a buffer big enough to hold most data
char *token; // this is to help us 'parse' the data in the file
int i; // this is our loop variable
rfp = fopen(filename, "r"); //TODO: set your mode !!!
//TODO: You should tell the user that if n > 100 (the size of our hero array) then we will
// have a problem
fgets(line, MAX_LINE_SIZE, rfp); // skip the column names
i=0;
while (feof(rfp) == 0) //TODO: loop for n - times - create this - MAKE SURE YOU PAY ATTENTION TO MAX HEROS
{
fgets(line, MAX_LINE_SIZE, rfp); // read the line from the file
// so we can 'parse' the data out into
// our structure super hero
token = strtok(line, ","); // start by using strtok to break up the line
strcpy(heroes[i].name, token); // copy the name first.
//TODO: Fix the properties
token = strtok(NULL, ","); // health
heroes[i].health = atof(token); // this converts the string into a number for us.
// atof - ASCII to Float (double)
token = strtok(NULL, ","); // strength?
heroes[i].strength = atof(token);
token = strtok(NULL, ","); // charisma?
heroes[i].charisma = atof(token);
token = strtok(NULL, ","); // intelligence?
heroes[i].intelligence = atof(token);
i++;
}
fclose(rfp); // we are done reading, so close the file now so we don't hold it open
// so that other people/programs cannot use it...
// return the total number of heros we read -
// notice we use a tertiary logical operator that is
// condition ? VALUE IF TRUE : VALUE IF FALSE
// this could be re-written as:
// if (n > maxHeroes) return maxHeroes;
// else return n;
return i - 1;
}
void printphoneBook(file addresses);
void addPerson(str name, str lastName, int phoneNumber, str address);
int main()
{
char userInput;
printf("What choice do you want? ");
while(userInput != 'e')
{
printf("a. Print the Phone List ");
printf("b. Add a Person ");
printf("c. Delete a Person ");
printf("d. Find Person ");
printf("e. Save and Quit ");
scanf("%c", &userInput);
if (userInput == 'a')
if (userInput == 'b')
printf(" Add a Person ");
if (userInput == 'c')
printf(" Delete a Person ");
if (userInput == 'd')
printf(" Find a Person ");
if (userInput == 'e')
printf(" Save and Quit ");
else
printf(" Sorry invalid input, try again ");
}
type def struct phoneBook
{
str lastName;
str firstName;
int phoneNumber;
str address;
}
void printphoneBook(" Print the Phone List ");
return 0;
}
This code (might be completely wrong) but it is a start to the linkedList.c C file
#include
#include
#include
struct person //declaring
{
char firstName [30];
char lastName [30];
char address [50]; //make a string of characters
char phoneNumber [20];
struct Person *next;
struct personLocation;
};
char search(personList *currentPerson, char name) //in order to search for a person in addr. book
{
while(currentPerson != NULL && currentPerson->person_name != name)
{
currentPerson = currentPerson->next;
}
if(currentPerson == NULL)
return 0;
return 1;
}
void printList(personList *currentPerson) //print the list of the person in addr. book
{
double sum = 0;
printf("The list is: ");
while(currentPerson != NULL)
{
printf("%i ->", currentPerson->person_name);
sum += currentPerson->lastName;
currentPerson = currentPerson->next;
}
printf("List of People in Address Book: %.1lf ", sum);
}
void insertPerson(personList **sPtr, int name, int lastName)
{
if(name
printf("Person must be positive! ");
else if (search(*sPtr, name))
printf("Person %i already exists! ", name);
else
{
personList *temp;
personList *newNode = (personList *)malloc(sizeof(personList));
newNode-> person_name = name;
newNode-> lastName = lastName; //check
if(*sPtr == NULL) //if the spot is NULL (empty)
*sPtr = newNode;
else
{
temp = *sPtr;
while(temp->next != NULL)
temp = temp->next;
temp->next = newNode;
}
}
printList(*sPtr);
}
void deletePerson(personList **sPtr, int name, int lastName) //insert lastName statement
{
personList *temp;
if(!search(*sPtr, name))
printf("Person %i is not found. ", name);
else
{
if(*sPtr != NULL)
{
temp = *sPtr;
if(temp->person_name == name)
*sPtr = temp->next;
else
{
while(temp->next != NULL && temp->next->person_name != name)
temp = temp->next;
if(temp->next->person_name == name)
temp->next = temp->next->next;
}
}
}
}
int main()
{
struct person
personList *list = NULL;
int name, choice;
double lastName;
printf("Enter your choice: a. Print the Phone List b. Add a Person c. Delete a Person d. Find a Person e. Save and Quit ");
while(1)
{
printf()
scanf("%i,& choice");
switch(choice)
{
case 'b':
printf("Enter person name and lastName to insert: ");
scanf("%i%lf", &name, &lastName);
insertPerson(&list, name, lastName);
break;
case 'c':
printList(list);
printf("Enter PErson name to delete:");
scanf("%i%lf", &name, &lastName);
deletePerson(&list, name, lastName);
break;
case 'e': return 0;
}
} //free right before it returns 0
}
, wrap Text AutoSum Calibri General Copy Format Painter | Fill- Paste B 1 u A -E] Merge & Center. $. % , S8' Conditional Format as Cell Insert Delete Format Formatting Table" Styles Clear Clipboard Font Alignment Number Styles Cells POSSIBLE DATA LOSS Some features might be lost if you save this workbook in the comma-delimited (.csv) format. To preserve these features, save it in an Excel file format. Don't show again Save A A1 last 1 last 2 Kringleris800-555-5410 Candycane Lane 3 Kent 4 Wayne Bruce 509-555-11412 Batcave Lane 5 Smith Sally 6 Smith Cameron 555-100-2:600 1st Ave 7 Anderson Cooper 506-555-16743rd St. 8 Obama Barack 555-555-51600 Pennslyvania Ave 9 McCain John 10 Dali 11 Gandhi Mahatma 671-555-3.1 Peace Lane 12 Church Winston 122-555-19 West Clearwater Apt A202 13 Romney Mitt irst phoneaddress Clark 509-555-11411 State St. 555-100-150001 68th St. 450-555-2:56 90th Ave NE Salvador509-555-96th Painting St. 405-555-9:5th Avenue 15 16 17 18 19 20 addresses ReadyStep by Step Solution
There are 3 Steps involved in it
Step: 1
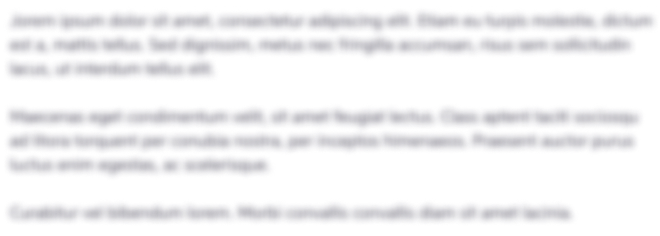
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started