Question
I NEED HELP WITH THIS CODE, I NEED TO BE ABLE TO STORE THE DATA ... AND PRINT IT OUT THE STORE DATA . I
I NEED HELP WITH THIS CODE, I NEED TO BE ABLE TO STORE THE DATA ...
AND PRINT IT OUT THE STORE DATA . I BELIEVE I WAS ABLE TO DO PART 1 BUT I NEED HELP IN....
PAR 2 OF THE PROGRAM.
PLEASE HELP.
THE PROGRAM DESCRIPTION:
You are writing a simple application to help Ernies Car Consignment, to keep track of his inventory. Ernie sells cars and truck for their owner
Part I (20 points)
You will first need to code Person, Vehicle, Car and Truck class. As you can see, Vehicle is the base class from which the Truck and Auto classes inherit. The two derived classes describe two types of Vehicle. Note that the owner field is of class Person described by the diagram:
The Vehicle class provides a constructor which initializes its instance data variables, in addition to accessor methods for each instance variable. Each of the subclasses provide additional data members specific to the type of Vehicle, plus additional methods needed to maintain the object. The desiredPrice is the price that the owner wants for the Vehicle.
Each of these classes provides a specific regCost method to compute the price the vehicle. Ernies pricing works as follows: each vehicle cost computation begins with the amount the owner wants for the vehicle. Ernie then adds 33% if it is a Car and 25% if it is a Truck. The totalCost for any vehicle is its regCost plus tax, which is 8.25%.
PART 2:
You are to write an application that will allow the user to store vehicle information. Your program should allow the user to do any of the following, until the user decides to exit.
enter a new Car
enter a new Truck
print out all Cars currently stored
print out all Trucks currently stored
accept a price as input, and output all Cars which are available under that price (before tax)
accept a price as input, and output all Trucks which are available under that price (before tax)
accept a price as input, and output all vehicles which are available for that price (before tax)
accept a car manufacturer, and output all Vehicles which are made by that manufacturer
Each object created must be stored for the remainder of the program, so you must have ONE list to store all vehicles.
CODE:
class Person: def __init__(self, name = "No Name"): self.name = name def __str__(self): return self.name
def setName(self, name): self.name = name
def getName(self): return self.name
# add the Vehicle class definition here class Vehicle: def __init__(self,manu,esize,name,price): self.manufacturer = manu self.engineSize = esize self.owner = name self.desiredPrice = price
def __str__(self): return self.name
def setManu(self, manu): self.manufacturer = manu
def setEng(self,esize): self.engineSize = esize
def setOwner(self,name): self.owner = name
def setPrice(self,price): self.price = price
def getManu(self): return self.manufacturer
def getSize(self): return self.engineSize
def getOwner(self): return self.owner
def regCost(self): return self.desiredPrice
def totalCost(self): return self.regCost() * 1.0825
# add the Truck class definition here.
class Truck(Vehicle): def __init__(self,manu,esize,name,load,tow,price): self.LoadCapacity = load self.TowCapacity = tow super().__init__(manu,esize,name,price)
def __str__(self): return self.owner
def setLoad(self,Load): self.LoadCapacity = Load
def setTow(self,tow): self.TowCapacity = tow
def getLoad(self): return self.LoadCapacity
def getTow(self): return self.TowCapacity
def regCost(self): return self.desiredPrice*(1.33)
# add the Car class definition here. class Car(Vehicle): def __init__(self,manu,esize,name,doors,price): self.Door = doors super().__init__(manu,esize,name,price)
def setDoor(self,door): self.Door = door
def getDoor(self): return self.Door
def regCost(self): return self.desiredPrice*(1.25)
def __str__(self): return self.owner def addCar(): print("Please enter car info:") owner = input("Owner: ") manu = input("Manufacturer: ") doors = input("Number of Doors: ") engineSize = input("Engine Size: ") desiredPrice = input("Price: ") human = Person(owner) newCar = Car(manu,int(engineSize),human,int(doors),float(desiredPrice)) return newCar def addTruck(): print("Please enter truck info:") owner = input("Owner: ") manu = input("Manufacturer: ") Load = input("Load Capacity: ") Tow = input("Tow Capacity: ") engineSize = input("Engine Size: ") desiredPrice = input("Price: ") human = Person(owner) newTruck = Truck(manu,int(engineSize),human,float(Load),float(Tow),float(desiredPrice)) return newTruck
def printCars(carList): # remove pass statement and add code here for car in carList: print ("owner: ",car.getOwner().getName()) print ("Manufacturer: ",car.getManu()) print ("Price: ",car.regCost()) print ("Engine Size: ",car.getSize()) print ("Doors: ",car.getDoor())
def printTrucks(TruckList): # remove pass statement and add code here for truck in TruckList: print ("owner: ",truck.getOwner().getName()) print ("Manufacturer: ",truck.getManu()) print ("Price: ",truck.regCost()) print ("Engine Size: ",truck.getSize()) print ("Load Capacity: ",truck.getLoad()) print ("Tow Capacity: ",truck.getTow())
def printCarPrice(CarList,price): # remove pass statement and add code here for car in CarList: if car.regCost() <= price: print ("owner: ",car.getOwner().getName()) print ("Manufacturer: ",car.getManu()) print ("Price: ",car.regCost()) print ("Engine Size: ",car.getSize()) print ("Doors: ",car.getDoor()) print ("") print ("******************************************************")
def printTruckPrice(TruckList,price): # remove pass statement and add code here for truck in TruckList: if truck.regCost() <= price: print ("owner: ",truck.getOwner().getName()) print ("Manufacturer: ",truck.getManu()) print ("Price: ",truck.regCost()) print ("Engine Size: ",truck.getSize()) print ("Load Capacity: ",truck.getLoad()) print ("Tow Capacity: ",truck.getTow()) print ("") print ("******************************************************") def printAllPrice(VehicleList,price): # remove pass statement and add code here for Vehicle in VehicleList: if Vehicle.regCost() <= price: print ("owner: ",Vehicle.getOwner().getName()) print ("Manufacturer: ",Vehicle.getManu()) print ("Price: ",Vehicle.regCost()) print ("Engine Size: ",Vehicle.getSize()) print ("") print ("******************************************************") def printVehicleManu(VehicleList,manufacturer): # remove pass statement and add code here for Vehicle in VehicleList: if Vehicle.getManu() == manufacturer: print ("owner: ",Vehicle.getOwner().getName()) print ("Manufacturer: ",Vehicle.getManu()) print ("Price: ",Vehicle.regCost()) print ("Engine Size: ",Vehicle.getSize()) print ("") print ("******************************************************") def main(): CarList = [] TruckList = [] VehicleList= [] more = True
while more: print("Welcome to Ernie's Car Consignment Program.") print("(1) Enter a new car") print("(2) Enter a new truck") print("(3) Print out all cars currently stored") print("(4) Print out all trucks currently stored") print("(5) Print out all cars avalaible under the entered price") print("(6) Print out all trucks avalaible under the entered price") print("(7) Print out all vehicles avalaible under the entered price") print("(8) Print out all vehicles made by the entered manufacturer") print("") choice = input("Please enter your choice (1-8): ") if choice == "1": newCar = addCar() CarList.append(newCar) VehicleList.append(newCar) elif choice == "2": newTruck = addTruck() TruckList.append(newTruck) VehicleList.append(newTruck) elif choice == "3": printCars(CarList) elif choice == "4": printTrucks(TruckList) elif choice == "5": price = input("Enter the desired price: ") printCarPrice(CarList,float(price)) elif choice == "6": price = input("Enter the desired price: ") printTruckPrice(TruckList,float(price)) elif choice == "7": price = input("Enter the desired price: ") printAllPrice(VehicleList,float(price)) elif choice == "8": Manufacturer = input("Enter the Manufacturer Name: ") printVehicleManu(VehicleList,Manufacturer) else: more = False
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
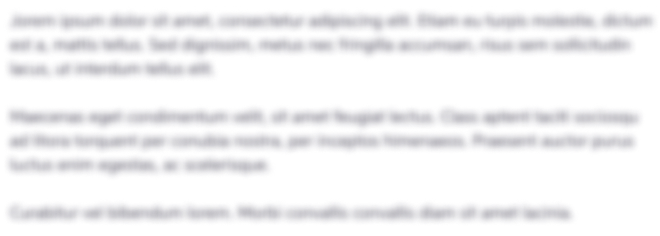
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started