Question
I need help with this programming assignment where we need to write a C++ program with the following functions: I am getting there, but I
I need help with this programming assignment where we need to write a C++ program with the following functions:
I am getting there, but I am extremely slow and I would like to compare my product with a proficient codder's work. Thank you!
intbin_to_dec(string s) |
INPUT(S): An UNsigned binary number as a string (e.g., 10110001) |
RETURN(S): The binary number converted to the equivalent decimal number |
PURPOSE: Converts any UNsigned binary number to decimal |
stringdec_to_bin( int n) |
INPUT(s): A non-negative decimal number (e.g, 15) |
RETURN(S): The decimal number converted to the equivalent binary number (as a string) |
PURPOSE: Converts any non-negative decimal number to binary |
inthex_to_dec(string s) |
INPUT(S): An UNsignedhexadecimal number as a string (e.g., FABC) |
RETURN(S): The hexadecimal number converted to the equivalent decimal number |
PURPOSE: Converts any UNsigned hexadecimal number to decimal |
stringdec_to_hex( int n) |
INPUT(s): A non-negative decimal number (e.g, 15) |
RETURN(S): The decimal number converted to the equivalent hexadecimal number (as a string) |
PURPOSE: Converts any non-negative decimal number to hexadecimal |
NOTE: A major component of this assignment is being able to convert between type char and type int, since binary/hexadecimal numbers are stored as strings, whereas decimal numbers are stored as ints. For example, we know from the ASCII code table that the character 0, represented as 0, has ASCII code 48. The character A has ASCII code 65, and so on. You have to handle converting 0 9 differently from converting A F. Here are some examples to show the point:
Example 1: convert any character 0 9 to the corresponding number 0 -9
char char1 = 0; // the ASCII code for 0 is 48 int num1 = char1 48; // the character 0 is converted to the number 0
Example 2: convert any number 0 9 to the corresponding character 0 9
int num1 = 0; char char1 = num1 + 48; // the number 0 is converted to the character 0
Example 3: convert any character A F to the corresponding number 10 -15
char char1 = A; // the ASCII code for A is 65 int num1 = char1 55; // the character A is converted to the number 10
Example 4: convert any number 10 15 to the corresponding character A F int num1 = 10; char char1 = num1 + 55;
// the number 10 is converted to the character A
**********************************************The starter code:**********************************************
// NOTE: The ONLY files that should be #included for this assignment are iostream, // cmath (for the pow function), // and string // No other files should be #included
#include
// NOTE: The ONLY files that should be #included for this assignment are iostream, // cmath (for the pow function), // and string // No other files should be #included
using namespace std;
int bin_to_dec(string); string dec_to_bin(int); int hex_to_dec(string); string dec_to_hex(int);
int main() { cout<<"10000011 binary = "< } // Converts any UNsigned binary number to decimal int bin_to_dec(string s) { // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION } //Converts any non-negative decimal number to binary string dec_to_bin(int n) { // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION } //Converts any UNsigned hexadecimal number to decimal int hex_to_dec(string s) { // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION } //Converts any non-negative decimal number to hexadecimal Converts any non-negative decimal number to hexadecimal string dec_to_hex(int n) { // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION // IMPLEMENT THIS FUNCTION }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
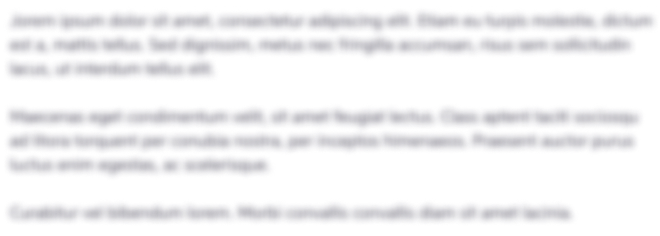
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started