Question
I need help with this project: (Please provide a detailed explanation) This is a Data Structure Project and use javadoc comments Requirements: Implement a HashTable
I need help with this project: (Please provide a detailed explanation)
This is a Data Structure Project and use javadoc comments
Requirements:
Implement a HashTable class. The hash table will be created from States5.csv.
Use the following class definition for a node in the table (put this inside your class
and do not change it, except to add comments):
private class Node
{
String stateName;
int statePopulation;
Node nextNode;
public Node(String state, int population)
{
stateName = state;
statePopulation = population;
}
public void printNode()
{
System.out.printf("%-25s%,10d ", stateName,
statePopulation);
}
}
The HashTable class must use Separate Chaining to deal with Collisions. For the
array of linked lists in the HashTable class, you must use singly linked list, NOT
doubly linked list! Moreover, insertions will happen at the beginning of the
linked list, and searching and deletions will need to go through the list. You will
need to implement this linked list class, so you must NOT use the LinkedList class
provided by Java. The class must use the following hash algorithm: add the Unicode values of all of
the characters in the state name (including spaces) and modulus the result with
101 (this means that you need a hash array of 101 elements.) Unicode value of a
character can be retrieved simply by using a type conversion from char to int. You
must NOT use any hash function provided by Java libraries to compute hash
values of state names.
(1). Create the HashTable class that implements the following public methods:
1. A no-arg constructor that creates an empty hash table.
2. The method: public void insert(String state, int population) that will insert a node into the
proper position in the hash table based on state name.
3. The method: public int find(String state) that will search the table for the state of the given
name and if found will return the population or -1 if not found.
4. The method: public void delete(String state) that fill find and delete the given state from the
table.
5. The method: public void display() that will traverse the table and will print the hash table as
follows:
1. Empty
2. Statename populationvalue
Statename populationvalue
Statename populationvalue
3. Empty
4. Statename populationvalue
5. Empty
6. Empty
. . .
101. Statename populationvalue
6. The method: public void printFreeAndCollisions() that will print the number of empty
spaces and the number of collisions in the table.
There are X spaces available and Y collisions in the hash table
(2). Create a class called Project5 that will
1. Read the States5.csv file of states and create a hash table by calling the insert method,
and display the hash table by calling the display method.
2. Delete states Vermont, California and South Carolina from the hash table by calling
the delete method.
3. Search for states Florida, Rhode Island and California by calling the find method. For
the found state, print out its population.
4. Delete states Kentucky, Minnesota, West Virginia and Ohio from the hash table, and
display the hash table by calling the display method.
5. Print the number of empty cells and the number of cells with collisions in the resulting
hash table by calling the printFreeAndCollisions function.
Provide comments in this form for the HashTable classes:
Comments for the class:
/**
* Detailed description of the class.
*
* @author <your name>
* @version
*/
Public method comments:
/**
* Description of the purpose of the method, the meaning of the
* input parameters (if any) and the meaning of the return values * (if any).
*
* @param parameter description of the parameter (one for each)
* @return description of the return value
*/
Provide comments in this form for the Project5 class.
/**
* COP 3538: Project 5 Hash Tables
*
* Description of the class using as many lines as needed
* with
between paragraphs. Including descriptions of the
* input required and output generated.
*
* @author <your name>
* @version <the date you last modified the program>
*/
public class Project5
{
Example Output:
COP3538 Project 5 Xudong Liu
Hash Tables
Enter the file name: States5.csv
There were 50 state records read into the hash table.
Hash table content:
0. Empty
1. Empty
2. Empty
3. Kansas 2,893,957
4. Oklahoma 3,850,568
5. Empty
6. California 38,332,521
40. Vermont 626,630
North Dakota 723,393
Connecticut 3,596,080
100. Nebraska 1,868,516
Vermont has been deleted from hash table
California has been deleted from hash table
South Carolina has been deleted from hash table
Florida is found with a population of 19,552,860
Rhode Island is found with a population of 1,051,511
California is not found
Kentucky has been deleted from hash table
Minnesota has been deleted from hash table
West Virginia has been deleted from hash table
Ohio has been deleted from hash table
Hash table content:
0. Empty
1. Empty
2. Empty
3. Kansas 2,893,957
4. Oklahoma 3,850,568
5. Empty
6. Empty
40. North Dakota 723,393
Connecticut 3,596,080
100. Nebraska 1,868,516
Hash table has X empty spaces and Y collisions.
This is the .csv file to read from:
State,Capital,Abbreviation,Population,Region,US House Seats
Alabama,Montgomery,AL,4833722,South,7
Alaska,Juno,AK,735132,West,1
Arizona,Phoenix,AZ,6626624,Southwest,9
Arkansas,Little Rock,AR,2959373,South,4
California,Sacramento,CA,38332521,West,53
Colorado,Denver,CO,5268367,West,7
Connecticut,Hartford,CT,3596080,New England,5
Delaware,Dover,DE,925749,Middle Atlantic,1
Florida,Tallahassee,FL,19552860,South,27
Georgia,Atlanta,GA,9992167,South,14
Hawaii,Honolulu,HI,1404054,West,2
Idaho,Boise,ID,1612136,West,2
Illinois,Springfield,IL,12882135,Midwest,18
Indiana,Indianapolis,IN,6570902,Midwest,9
Iowa,Des Moines,IA,3090416,Midwest,4
Kansas,Topeka,KS,2893957,Midwest,4
Kentucky,Frankfort,KY,4395295,South,6
Louisiana,Baton Rouge,LA,4625470,South,6
Maine,Augusta,ME,1328302,New England,2
Maryland,Annapolis,MD,5928814,Middle Atlantic,8
Massachusetts,Boston,MA,6692824,New England,9
Michigan,Lansing,MI,9895622,Midwest,14
Minnesota,St Paul,MN,5420380,Midwest,8
Mississippi,Jackson,MS,2991207,South,4
Missouri,Jefferson City,MO,6044171,Midwest,8
Montana,Helena,MT,1015165,West,1
Nebraska,Lincoln,NE,1868516,Midwest,3
Nevada,Carson City,NV,2790136,West,4
New Hampshire,Concord,NH,1323459,New England,2
New Jersey,Trenton,NJ,8899339,Middle Atlantic,12
New Mexico,Santa Fe,NM,2085287,Southwest,3
New York,Albany,NY,19651127,Middle Atlantic,27
North Carolina,Raleigh,NC,9848060,South,13
North Dakota,Bismarck,ND,723393,Midwest,1
Ohio,Columbus,OH,11570808,Midwest,16
Oklahoma,Oklahoma City,OK,3850568,Southwest,5
Oregon,Salem,OR,3930065,West,5
Pennsylvania,Harrisburg,PA,12773801,Middle Atlantic,18
Rhode Island,Providence,RI,1051511,New England,2
South Carolina,Columbia,SC,4774839,South,7
South Dakota,Pierre,SD,844877,Midwest,1
Tennessee,Nashville,TN,6495978,South,9
Texas,Austin,TX,26448193,Southwest,36
Utah,Salt Lake City,UT,2900872,West,4
Vermont,Montpelier,VT,626630,New England,1
Virginia,Richmond,VA,8260405,Middle Atlantic,11
Washington,Olympia,WA,6971406,West,10
West Virginia,Charleston,WV,1854304,Middle Atlantic,3
Wisconsin,Madison,WI,5742713,Midwest,8
Wyoming,Cheyenne,WY,582658,West,1
Thanks.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
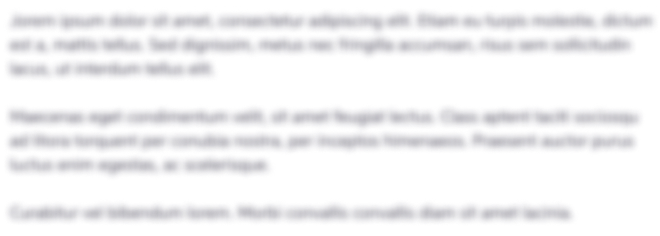
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started