Question
I need helping creating a Java program. Please read instructions. A basic and simple code would be appreciated. In a triangle, the sum of any
I need helping creating a Java program. Please read instructions. A basic and simple code would be appreciated.
In a triangle, the sum of any two sides is greater than the other side. The Triangle class must adhere to this rule. Create the IllegalTriangleException class, and modify the constructor of the Triangle class to throw an IllegalTriangleException object if a triangle is created with sides that violate the rule, as follows:
/** Construct a triangle with the specified sides */
public Triangle2 (double side1, double side2, double side3) throws IllegalTriangleException
{
// Implement it
}
You will make a custom exception class. See Listing 12.10, pg. 470, for an example. TestTriangle.java has been provided that has a main that you will need to add exception handling code and also in the class Triangle2. Triangle2 is declared in TestTriangle.java The class is named Triangle2 to distinguish from the Triangle class from an earlier assignment.
TestTriangle.java
import java.util.Scanner;
public class TestTriangle { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter three sides: "); double side1 = input.nextDouble(); double side2 = input.nextDouble(); double side3 = input.nextDouble();
Triangle2 triangle = new Triangle2(side1, side2, side3);
System.out.println("The area is " + triangle.getArea()); System.out.println("The perimeter is " + triangle.getPerimeter()); System.out.println(triangle);
} }
class Triangle2 { private double side1 = 1.0, side2 = 1.0, side3 = 1.0;
/** Constructor */ public Triangle2() { }
/** Constructor */ public Triangle2(double side1, double side2, double side3) { this.side1 = side1; this.side2 = side2; this.side3 = side3; }
public double getSide1() { return side1; }
public double getSide2() { return side2; }
public double getSide3() { return side3; }
public double getArea() { double s = (side1 + side2 + side3) / 2; return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3)); }
public double getPerimeter() { return side1 + side2 + side3; }
public String toString() { // Implement it to return the three sides return "Triangle: side1 = " + side1 + " side2 = " + side2 + " side3 = " + side3; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
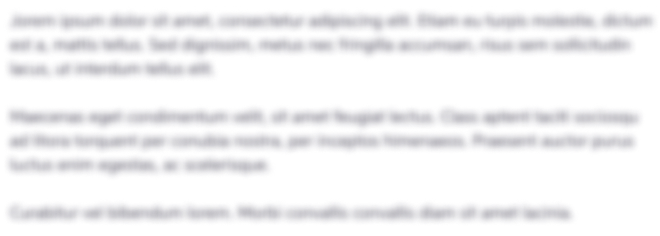
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started