Question
I need JAVA CODE TO BE MODIFIED TO ACCOMPLISH AS PER THE INSTRUCTION BELOW: Employee Class: The Employee information you must track is as follows:
I need JAVA CODE TO BE MODIFIED TO ACCOMPLISH AS PER THE INSTRUCTION BELOW:
Employee Class:
The Employee information you must track is as follows: • ID (This is generated randomly) • Name • Gender • Job Title • Organization they work for • Birthday As for the Organization that the Employee works for, you must also track this information: • Organization Name • Number of Employees of each organization Note: If an employee is added to a specific company, only the number of employees in that company is incremented by one, not the other.
Caller class:
You should be displaying a menu where the user can choose an action of the following: • Add Employee. • Remove Employee. • Update Employees Information: o Update the name. o Update the job title. o Update the workplace of an employee. • Print the number of employees: o In the whole system. o In Google only. o In Microsoft only. • Print employees' information: o Print the information of a single employee. o Print the information of all employees in the system. o Print the information of Google employees. o Print the information of Microsoft employees. Note: As a requirement for all inputs in your system, you must do error checking. For example, if the user to enter a whole number, nothing accepted but integers (NO characters, floating point numbers, or text of any kind), etc
//THE CODE BELOW WORKS FINE BUT PLEASE MODIFY IT FOR THE EMPLOYEE ID TO BE //GENERATED RANDOMLY
Employee.java (Employee class);
public class Employee {
private int empid;
private String empname;
private char gender;
private String jobdtitle;
private String orgname;
private String dob;
public static int googleempcount = 0;
public static int microsoftempcount = 0;
public static int empCount = 0;
public int getEmpid() {
return empid;
}
public void setEmpid(int empid) {
this.empid = empid;
}
public String getEmpname() {
return empname;
}
public void setEmpname(String empname) {
this.empname = empname;
}
public char getGender() {
return gender;
}
public void setGender(char gender) {
this.gender = gender;
}
public String getJobdtitle() {
return jobdtitle;
}
public void setJobdtitle(String jobdtitle) {
this.jobdtitle = jobdtitle;
}
public String getOrgname() {
return orgname;
}
public void setOrgname(String orgname) {
this.orgname = orgname;
}
public String getDob() {
return dob;
}
public void setDob(String dob) {
this.dob = dob;
}
/**
*
*/
public Employee() {
super();
}
/**
* @param empname
* @param gender
* @param jobdtitle
* @param orgname
* @param dob
*/
public Employee(String empname, char gender, String jobdtitle, String orgname, String dob) {
super();
this.empid = ++empCount;
this.empname = empname;
this.gender = gender;
this.jobdtitle = jobdtitle;
this.orgname = orgname;
this.dob = dob;
if (orgname.toLowerCase().equals("google")) {
googleempcount++;
} else if (orgname.toLowerCase().equals("microsoft")) {
microsoftempcount++;
}
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (!(obj instanceof Employee))
return false;
Employee other = (Employee) obj;
if (empid != other.empid)
return false;
return true;
}
@Override
public String toString() {
return "Employee [empid=" + empid + ", empname=" + empname + ", gender=" + gender + ", jobdtitle=" + jobdtitle
+ ", orgname=" + orgname + ", dob=" + dob + "]";
}
}
EmployeeSystem.java (EmployeeSystem class):
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class EmployeeSystem {
static List employees = new ArrayList<>();
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int userChoice = 0;
while (userChoice != 6) {
System.out.println("Please choose one option.");
System.out.println("Enter 1 for add employee.");
System.out.println("Enter 2 for update employee.");
System.out.println("Enter 3 for remove employee.");
System.out.println("Enter 4 for printing number of employee's.");
System.out.println("Enter 5 for printing employee's. info");
System.out.println("Enter 6 for quit");
userChoice = sc.nextInt();
if (userChoice == 1) {
addEmployee();
} else if (userChoice == 2) {
System.out.println("Please enter empId.");
int empId = sc.nextInt();
System.out.println("Please choose one option.");
System.out.println("Enter 1 for update name.");
System.out.println("Enter 2 for update job Title.");
System.out.println("Enter 3 for updating workplace.");
userChoice = sc.nextInt();
if (userChoice == 1) {
System.out.println("Please enter name");
String name = sc.next();
for (Employee emp : employees) {
if (emp.getEmpid() == empId) {
emp.setEmpname(name);
System.out.println("Updated");
}
}
} else if (userChoice == 2) {
System.out.println("Please enter job title");
String jobTitle = sc.next();
for (Employee emp : employees) {
if (emp.getEmpid() == empId) {
emp.setJobdtitle(jobTitle);
System.out.println("Updated");
}
}
} else if (userChoice == 3) {
for (Employee emp : employees) {
if (emp.getEmpid() == empId) {
if (emp.getOrgname().equals("google")) {
emp.setOrgname("microsoft");
Employee.googleempcount--;
Employee.microsoftempcount++;
} else {
emp.setOrgname("google");
Employee.googleempcount++;
Employee.microsoftempcount--;
}
System.out.println("Updated");
}
}
} else {
System.out.println("Invalid choice.");
}
} else if (userChoice == 3) {
System.out.println("Please enter empId.");
int empId = sc.nextInt();
for (Employee emp : employees) {
if (emp.getEmpid() == empId) {
Employee.empCount--;
if (emp.getOrgname().equals("google")) {
Employee.googleempcount--;
} else {
Employee.microsoftempcount--;
}
break;
}
}
Employee emp = new Employee();
emp.setEmpid(empId);
if (!employees.remove(emp)) {
System.out.println("Invalid empid");
} else {
System.out.println("Removed");
}
} else if (userChoice == 4) {
System.out.println("Please choose one option.");
System.out.println("Enter 1 for Whole system.");
System.out.println("Enter 2 for google employee.");
System.out.println("Enter 3 for microsoft employee.");
userChoice = sc.nextInt();
if (userChoice == 1) {
System.out.println("Total number of employees are : " + Employee.empCount);
} else if (userChoice == 2) {
System.out.println("Total number of employees in Google are: " + Employee.googleempcount);
} else if (userChoice == 3) {
System.out.println("Total number of employees in Microsoft are: " + Employee.microsoftempcount);
} else {
System.out.println("Invalid choice.");
}
} else if (userChoice == 5) {
System.out.println("Please choose one option.");
System.out.println("Enter 1 for single employee.");
System.out.println("Enter 2 for all employees.");
System.out.println("Enter 3 for google employees.");
System.out.println("Enter 4 for microsoft employees.");
userChoice = sc.nextInt();
if (userChoice == 1) {
System.out.println("Please enter empId:");
int empId = sc.nextInt();
for (Employee emp : employees) {
if (emp.getEmpid() == empId) {
System.out.println(emp.toString());
break;
}
}
} else if (userChoice == 2) {
for (Employee emp : employees) {
System.out.println(emp.toString());
}
} else if (userChoice == 3) {
for (Employee emp : employees) {
if (emp.getOrgname().equals("google")) {
System.out.println(emp.toString());
}
}
} else if (userChoice == 4) {
for (Employee emp : employees) {
if (emp.getOrgname().equals("microsoft")) {
System.out.println(emp.toString());
}
}
} else {
System.out.println("Invalid choice.");
}
} else if (userChoice == 6) {
break;
} else {
System.out.println("Please enter valid choice.");
}
}
sc.close();
}
private static void addEmployee() {
System.out.println("Enter name: ");
Scanner sc1 = new Scanner(System.in);
String name = sc1.next();
System.out.println("Enter gender (m/f): ");
String gender = sc1.next();
while (!(gender.equals("m") || gender.equals("f"))) {
System.out.println("Please enter valid gender only m or f");
gender = sc1.next();
}
System.out.println("Enter Job title: ");
String jobTitle = sc1.next();
System.out.println("Enter Organisation name: ");
String orgName = sc1.next();
System.out.println("Enter DOB: ");
String dob = sc1.next();
Employee emp = new Employee(name, gender.toCharArray()[0], jobTitle, orgName, dob);
employees.add(emp);
System.out.println("Employee added with empId : " + emp.getEmpid());
}
}
Step by Step Solution
3.49 Rating (159 Votes )
There are 3 Steps involved in it
Step: 1
To modify the program for generating employee IDs randomly you can use javautilUUID to generate unique identifiers Heres the modified Employee class a...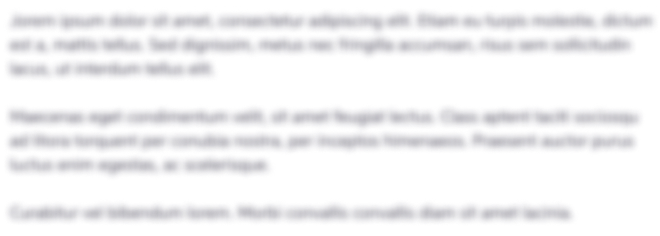
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started