Question
I need main help with toString in User class. Need to produce the following image. I mainly need the trophies part of the toString. Since
I need main help with toString in User class. Need to produce the following image. I mainly need the trophies part of the toString. Since it is in an Array, i don't know how to make the array release into the string line by line as per the image. right now it outputs in Array format [a, b, c, d] style. I need this to work for variable array sizes not just one size.
String string = username + all the trophy stuff + all the game stuff + date. I can get the username and birthdate stuff, not the trophy and game stuff for variable lengths.
The User class requires the following instance variables:
private String username; private int level; private ArrayList trophies; private GameList games; private Calendar dob; private User left; private User right;
An ArrayList type was chosen for variable trophies as you figured it would be easier to add a new trophy to a list than a standard array, and you probably would mostly just traverse the list in order.
The toString method should output per the image
Code Skeleton below
package Database;
import java.util.ArrayList; import java.util.Calendar;
public class User {
private String username; private int level; private Calendar dob; private ArrayList trophies; private GameList games; private User left; private User right;
public User(String username, Calendar dob, int level) {
}
public String getUsername() { return null; }
public int getLevel() { return 0; }
public double getKey() { return 0; }
public Calendar getDob() { return null; }
public ArrayList getTrophies() { return null; }
public GameList getGames() { return null; }
public User getLeft() { return null; }
public User getRight() { return null; }
public void setTrophies(ArrayList trophies) {
}
public void setGames(GameList games) {
}
public void setLeft(User left) {
}
public void setRight(User right) {
}
@Override public String toString() { return null; } }
------> Trophy Class
package Database;
import java.util.Calendar;
public class Trophy {
public enum Rank { BRONZE, SILVER, GOLD, PLATINUM }
public enum Rarity { COMMON, UNCOMMON, RARE, VERY_RARE, ULTRA_RARE }
private String name; private Rank rank; private Rarity rarity; private Calendar obtained; private Game game;
public Trophy() {}
public Trophy(String name, Rank rank, Rarity rarity, Calendar obtained, Game game) {
}
public String getName() { return null; }
public Rank getRank() { return null; }
public Rarity getRarity() { return null; }
public Calendar getObtained() { return null; }
public Game getGame() { return null; }
@Override public String toString() { return null; } } ------> GameList Class
package Database;
public class GameList {
public Game head;
public GameList(Game head) {
}
public Game getGame(String game) { return null; }
public void addGame(Game game) {
}
public void removeGame(String game) {
}
public void removeGame(Game game) {
}
@Override public String toString() { return null; }
}
User: lewdster Trophies: "Whole game, no kills", rank: GOLD, rarity: ULTRA_RARE, obtained on: Jan 25, 2016 "Big dragon, you killed it", rank: SILVER, rarity: UNCOMMON, obtained on: Jun 15, 2014 "How did you manage to get this on PS4?!", rank: PLATINUM, rarity: ULTRA_RARE, obtained on: Feb 21, 2016 "Watched a cutscene, here's a trophy", rank: BRONZE, rarity: COMMON, obtained on: Jan 15, 2016 Games: "Assassin's Creed IV: Black Flag", released on: Nov 29, 2013 "Child of Light", released on: May 01, 2014 "Dragon Age: Inquisition", released on: Dec 18, 2014 Birth Date: Jun 16, 1980 User: lewdster Trophies: "Whole game, no kills", rank: GOLD, rarity: ULTRA_RARE, obtained on: Jan 25, 2016 "Big dragon, you killed it", rank: SILVER, rarity: UNCOMMON, obtained on: Jun 15, 2014 "How did you manage to get this on PS4?!", rank: PLATINUM, rarity: ULTRA_RARE, obtained on: Feb 21, 2016 "Watched a cutscene, here's a trophy", rank: BRONZE, rarity: COMMON, obtained on: Jan 15, 2016 Games: "Assassin's Creed IV: Black Flag", released on: Nov 29, 2013 "Child of Light", released on: May 01, 2014 "Dragon Age: Inquisition", released on: Dec 18, 2014 Birth Date: Jun 16, 1980Step by Step Solution
There are 3 Steps involved in it
Step: 1
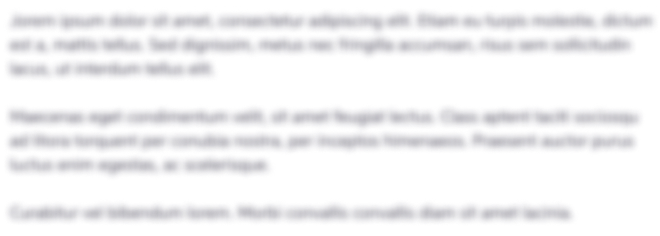
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started