Question
I need max points please C++ The equation of a line in standard form is ax + by = c , wherein both aand b
I need max points please C++
The equation of a line in standard form is ax + by = c , wherein both aand b cannot be zero, and a, b, and c are real numbers. If b0, then a/b is the slope of the line. If a = 0, then it is a horizontal line, and if b = 0, then it is a vertical line. The slope of a vertical line is undefined. Two lines are parallel if they have the same slope or both are vertical lines. Two lines are perpendicular if either one of the lines is horizontal and the other is vertical or the product of their slopes is 1.
Design the class lineType to store a line. To store a line, you need to store the values of a (coefficient of x), b (coefficient of y), and c. Your class must contain the following operations:
If a line is nonvertical, then determine its slope.
Determine if two lines are equal. (Two lines ax + by = c and ax + by = c are equal if either a = a, b = b, and c = c, or a = ka, b = kb and c = kc, and for some real number k.)
PLEASE MAKE SURE TO ANSWER ALL PARTS! thank you
Determine if two lines are parallel.
Determine if two lines are perpendicular.
If two lines are not parallel, then print the point of intersection. The intersection method should indicate one of three options: The intersection point in the format: (x, y) . A message indicating Both lines are equal. A message indicating the Lines do not intersect.
Add appropriate constructors to initialize variables of lineType. Also, write a program to test your class.
I have included the given file names and a code.
/ lineType.h /
#ifndef LINETYPE_LINETYPE_H
#define LINETYPE_LINETYPE_H
#include
using namespace std;
class lineType
{
//Overload the stream insertion and extraction operators
friend ostream& operator<<(ostream&, const lineType&);
friend istream& operator>>(istream&, lineType&);
public:
//overload the assignment operator
const lineType& operator=(const lineType&);
//Function to set the line.
void setLine(double a = 0, double b = 0, double c = 0);
double getXCoefficient() const;
double getYCoefficient() const;
double getCOnstantTerm() const;
void setXCoefficient(double coeff);
void setYCoefficient(double coeff);
void setConstantTerm(double c);
double slope() const;
bool operator+() const;
bool operator-() const;
bool operator==(const lineType& otherLine) const;
bool operator||(const lineType& otherLine) const;
bool operator&&(const lineType& otherLine) const;
void pointOfIntersection(lineType otherLine);
lineType(double a = 0, double b = 0, double c = 0);
private:
double xCoefficient;
double yCoefficient;
double constTerm;
};
#endif
/ lineTypeImp.cpp /
#include
#include
#include "lineType.h"
using namespace std;
//object stream of line type
ostream& operator<<(ostream& lineType, const class lineType& lineOut)
{
if (lineOut.xCoefficient == 0)
{
if(lineOut.yCoefficient < 0)
{
lineType << '-' << setw(2) << -lineOut.yCoefficient << 'y';
lineType << setw(2) << '=' << setw(2) << lineOut.constTerm;
}
else if(lineOut.yCoefficient > 0)
{
lineType << setw(2) << '+' << setw(2) << lineOut.yCoefficient << 'y';
lineType << setw(2) << '=' << setw(2) << lineOut.constTerm;
}
else
cout << "There is no line " << endl;
}
else if(lineOut.yCoefficient == 0)
{
if(lineOut.xCoefficient < 0)
{
lineType << '-' << setw(2) << -lineOut.xCoefficient << 'x';
lineType << setw(2) << '=' << setw(2) << lineOut.constTerm;
}
else if(lineOut.xCoefficient > 0)
{
lineType << '+' << setw(2) << lineOut.xCoefficient << 'x';
lineType << setw(2) << '=' << setw(2) << lineOut.constTerm;
}
else
cout << "There is no line " << endl;
}
else
{
if(lineOut.xCoefficient < 0)
{
lineType << '-' << -lineOut.xCoefficient << 'x';
if(lineOut.yCoefficient < 0)
{
lineType << '-' << -lineOut.yCoefficient << 'y';
lineType << '=' << lineOut.constTerm;
}
else
{
lineType << '+' << lineOut.yCoefficient << 'y';
lineType << '=' << lineOut.constTerm;
}
}
if(lineOut.xCoefficient > 0)
{
lineType << lineOut.xCoefficient << 'x' << setw(2);
if(lineOut.yCoefficient < 0)
{
lineType << '-' << setw(2) << -lineOut.yCoefficient << 'y';
lineType << setw(2) << '=' << setw(2) << lineOut.constTerm;
}
else
{
lineType << '+' << setw(2) << lineOut.yCoefficient << 'y';
lineType << setw(2) << '=' << setw(3) << lineOut.constTerm;
}
}
}
return lineType;
}
//line operators
istream& operator>>(istream& stream, lineType& lineIn)
{
char character;
stream >> lineIn.xCoefficient;
stream.get(character);
stream >> lineIn.yCoefficient;
stream.get(character);
stream >> lineIn.constTerm;
return stream;
}
//line x and y coefficient
const lineType& lineType::operator=(const lineType& line)
{
this->xCoefficient = line.xCoefficient;
this->yCoefficient = line.yCoefficient;
this->constTerm = line.constTerm;
return *this;
}
//set line type
void lineType::setLine(double a, double b, double c)
{
xCoefficient = a;
yCoefficient = b;
constTerm = c;
}
double lineType::getXCoefficient() const
{
return xCoefficient;
}
double lineType::getYCoefficient() const
{
return yCoefficient;
}
double lineType::getCOnstantTerm() const
{
return constTerm;
}
void lineType::setXCoefficient(double coeff)
{
xCoefficient = coeff;
}
void lineType::setYCoefficient(double coeff)
{
yCoefficient = coeff;
}
void lineType::setConstantTerm(double c)
{
constTerm = c;
}
bool lineType::operator+() const
{
return (this->yCoefficient == 0);
}
bool lineType::operator-() const
{
return (this->xCoefficient == 0);
}
bool lineType::operator==(const lineType& otherLine) const
{
return (((this->constTerm / this->xCoefficient) == (otherLine.constTerm / otherLine.xCoefficient)) && ((this->constTerm / this->yCoefficient) == (otherLine.constTerm / otherLine.yCoefficient)) );
}
bool lineType::operator||(const lineType& otherLine) const
{
if (yCoefficient == 0 && otherLine.yCoefficient == 0)
return true;
else if ((yCoefficient != 0 && otherLine.yCoefficient == 0)
|| (yCoefficient == 0 && otherLine.yCoefficient != 0))
return false;
else if ((xCoefficient / yCoefficient) == (otherLine.xCoefficient / otherLine.yCoefficient))
return true;
else
return false;
}
bool lineType::operator&&(const lineType& otherLine) const
{
return ( (this->slope() * otherLine.slope() == -1) );
}
double lineType::slope() const
{
double slope;
slope = - xCoefficient / yCoefficient;
return slope;
}
//check line point of intersection
void lineType::pointOfIntersection(lineType otherLine)
{
if(*this == otherLine)
{
cout << "Both lines are equal. They have infinite points of intersections." << endl;
}
else if(*this || otherLine)
{
cout << "There is no point of intersection" << endl;
}
else
{
float a;
float b;
a = (this->constTerm * otherLine.yCoefficient - this->yCoefficient * otherLine.constTerm) / (this->xCoefficient * otherLine.yCoefficient - this->yCoefficient * otherLine.xCoefficient);
b = (this->constTerm * otherLine.xCoefficient - this->xCoefficient * otherLine.constTerm) / (this->yCoefficient * otherLine.xCoefficient - this->xCoefficient * otherLine.yCoefficient);
cout << "Point of intersection is: (" << a << "," << b << ")" << endl;
}
}
//check line type
lineType::lineType(double a,double b,double c)
{
lineType::setLine(a,b,c);
}
/ main.cpp /
#include
#include
#include "lineType.h"
#include "lineType.cpp"
using namespace std;
int main()
{
//declare line type objects
lineType line1(2,3,4),
line2(3,5,7),
line3(2,3,-2),
line4(3,-2,1),
line5(2,0,3),
line6(0,-1,2),
line7(4,6,8),
line8;
cout << "Line1: " << line1 << endl;
//slope of line
cout << "The slope of line1: " << line1.slope() << endl;
//line2
cout << "line2: " << line2 << endl;
//line3
cout << "line3: " << line3 << endl;
//line4
cout << "line4: " << line4 << endl;
//line5
cout << "line5: " << line5 << endl;
//line6
cout << "line6: " << line6 << endl;
//line7
cout << "line7: " << line7 << endl;
//check if lines are parallel
if(line1 || line2)
cout << "line1 and line2 are parallel" << endl;
else
cout << "line1 and line2 are not parallel" << endl;
line1.pointOfIntersection(line2);
if(line1 || line3)
cout << "line1 and line3 are parallel" << endl;
else
cout <<"line 1 and line3 are not parallel" << endl;
//check if lines are perpendicular
if(line1 && line4)
cout << "line1 and line4 are perpendicular" << endl;
else
cout << "line1 and line4 are not perpendicular" << endl;
//check if lines are vertical
if(+(line5))
cout << "line5 is a vertical line" << endl;
if(-(line6))
cout << "line6 is a horizontal line" << endl;
//check point of intersection of lines
line5.pointOfIntersection(line6);
if(line1 == line7)
cout << "line1 and line7 are equal" << endl;
line1.pointOfIntersection(line7);
//prompt user to input the data
cout << "Input line8 in the form(a,b,c: (";
cin >> line8;
cout << line8 << endl;
//check and print if lines are parallel or not
if(line1 || line8)
cout << "line1 and line8 are parallel" << endl;
else
cout << "line1 and line8 are not parallel" << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
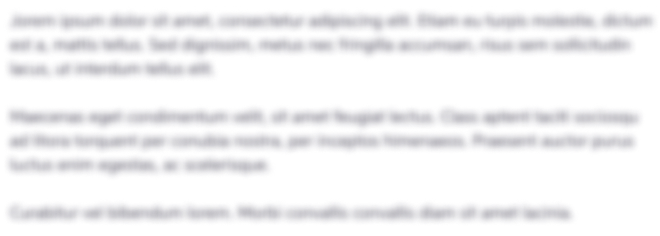
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started