Question
I need my Java program to reset and start a new order when the New Order button is pressed. I know I have to reset
I need my Java program to reset and start a new order when the New Order button is pressed. I know I have to reset the variables but am I missing anything else in my code?
This is my Summation Class
public class Summation {
public static double subTotal(String arrayFromFile, int howMany) {
double subTotal;
double price = Double.parseDouble(arrayFromFile);
subTotal = price * howMany;
//System.out.println(price);
//System.out.println(howMany);
//cost = howMany * arrayFromFile;
return subTotal;
}
}
This is the ReadFile class
import java.io.*;
import java.util.*;
public class ReadFile {
public static int myMethod()
{
int numberOfLines = 0;
try
{
// Open the file that is the first
// command line parameter
FileInputStream fstream = new FileInputStream("inventory.txt");
// Get the object of DataInputStream
DataInputStream in = new DataInputStream(fstream);
BufferedReader br = new BufferedReader(new InputStreamReader(in));
String strLine;
//Read File Line By Line
while ((strLine = br.readLine()) != null)
{
// Print the content on the console
//System.out.println (strLine);
++numberOfLines;
}
//Close the input stream
in.close();
br.close();
}
catch (Exception e)
{
//Catch exception if any
System.err.println("Error: " + e.getMessage());
}
//System.out.println(numberOfLines);
return numberOfLines;
}
public static String[][] convertTo2DArray() {
String [][] array2D = null;
try
{
FileInputStream fstream = new FileInputStream("inventory.txt");
DataInputStream in = new DataInputStream(fstream);
BufferedReader br = new BufferedReader(new InputStreamReader(in));
String thisLine;
List
//Read File Line By Line
while ((thisLine = br.readLine()) != null)
{
lines.add(thisLine.split(","));
}
array2D = new String[lines.size()][0];
lines.toArray(array2D);
//Close the input stream
in.close();
br.close();
}
catch (Exception e)
{
//Catch exception if any
System.err.println("Error: " + e.getMessage());
}
//System.out.println(numberOfLines);
return array2D;
}
}
The following code is the class with most of my code called Project1GUI
/*Name: Kenneth Gonzalez
* Course: CNT 4714 - Spring 2018
* Assignment Title: Program 1 - Event Driven Programming
* Date: Sunday January 28, 2018
*/
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JTextField;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.awt.event.ActionEvent;
import java.awt.Font;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.lang.String;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.awt.Color;
public class Project1GUI {
private JFrame frame;
private JTextField txtFieldNumOfItems;
private JTextField BookID;
private JTextField Quantity;
private JTextField BookInformation;
private JTextField Subtotal;
private JButton btnProcessItem;
private JButton btnConfirmItem;
private JButton btnViewOrder;
private JButton btnFinishOrder;
private int numOfItems;
int quantityOfBook;
private int count = 1;
private int subTotalCount = 0;
private double subTotalforItems = 0;
private static String array2DFromFile[][];
private static String arrayFromFile[];
String [][]array2 = null;
static int numRows;
static int numCols;
int count1 = 0;
int bookIDProc = 0;
double subTotal = 0;
String everything = null;
String ViewOrderOutput="";
String FinishOrderOutput="";
String text;
String numberList;
String s = null;
String result;
String discount = null;
ArrayList
ArrayList
static ArrayList
ArrayList
/**
* Launch the application.
*/
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
try
{
Project1GUI window = new Project1GUI();
window.frame.setVisible(true);
//Calling the start function that begins by reading in the file.
start();
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public static void start()
{
//Parses the file and returns the elements in a 2D array.
array2DFromFile = ReadFile.convertTo2DArray();
numCols = array2DFromFile[0].length;
numRows = array2DFromFile.length;
//Add the elements of the 2D array to a 1D array so we can check if a BOOKID exist or doesn't exist.
for(int x = 0; x < numRows; x++)
{
for(int y = 0; y < numCols; y++)
{
list.add(array2DFromFile[x][y]);
}
}
arrayFromFile = new String[list.size()];
for(int i = 0; i < arrayFromFile.length; i++)
{
arrayFromFile[i] = list.get(i);
}
}
/**
* Create the application.
*/
public Project1GUI() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
@SuppressWarnings("deprecation")
private void initialize() {
frame = new JFrame();
frame.getContentPane().setBackground(Color.GREEN);
frame.setBounds(100, 100, 920, 432);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(null);
txtFieldNumOfItems = new JTextField();
txtFieldNumOfItems.setBounds(370, 50, 376, 22);
frame.getContentPane().add(txtFieldNumOfItems);
txtFieldNumOfItems.setColumns(10);
BookID = new JTextField();
BookID.setBounds(370, 97, 376, 22);
frame.getContentPane().add(BookID);
BookID.setColumns(10);
Quantity = new JTextField();
Quantity.setBounds(370, 147, 376, 22);
frame.getContentPane().add(Quantity);
Quantity.setColumns(10);
BookInformation = new JTextField();
BookInformation.setBounds(370, 197, 376, 22);
frame.getContentPane().add(BookInformation);
BookInformation.setColumns(10);
BookInformation.setEditable(false);
Subtotal = new JTextField();
Subtotal.setBounds(370, 251, 376, 22);
frame.getContentPane().add(Subtotal);
Subtotal.setColumns(10);
Subtotal.setEditable(false);
JLabel lblItemsInOrder = new JLabel("Enter the number of items in this order: ");
lblItemsInOrder.setFont(new Font("Times New Roman", Font.PLAIN, 16));
lblItemsInOrder.setBounds(43, 53, 274, 19);
frame.getContentPane().add(lblItemsInOrder);
JLabel lblBookID = new JLabel("Enter BookID for item #" + count +":");
lblBookID.setFont(new Font("Times New Roman", Font.PLAIN, 16));
lblBookID.setBounds(43, 100, 274, 16);
frame.getContentPane().add(lblBookID);
JLabel lblQuantity = new JLabel("Enter quantity for item #" + count +":");
lblQuantity.setFont(new Font("Times New Roman", Font.PLAIN, 16));
lblQuantity.setBounds(43, 150, 274, 16);
frame.getContentPane().add(lblQuantity);
JLabel lblItemInfo = new JLabel("Item #" + count + " info:");
lblItemInfo.setFont(new Font("Times New Roman", Font.PLAIN, 16));
lblItemInfo.setBounds(43, 200, 274, 16);
frame.getContentPane().add(lblItemInfo);
JLabel lblSubtotal = new JLabel("Order Subtotal for " +subTotalCount+ " item(s):");
lblSubtotal.setFont(new Font("Times New Roman", Font.PLAIN, 16));
lblSubtotal.setBounds(43, 254, 274, 16);
frame.getContentPane().add(lblSubtotal);
btnProcessItem = new JButton("Process Item # " + count);
btnProcessItem.setBounds(43, 337, 131, 25);
frame.getContentPane().add(btnProcessItem);
//If Process Item button is pressed take certain actions
btnProcessItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0)
{
//Disable how many items the customer wants after they press the Process Item button.
numOfItems = Integer.parseInt(txtFieldNumOfItems.getText());
txtFieldNumOfItems.setEditable(false);
//Check to see if the ID entered is 5 characters long
if(count1 < numOfItems)
{
if(BookID.getText().length() == 5)
{
s = BookID.getText();
bookIDProc = Integer.parseInt(BookID.getText());
}
else if(BookID.getText().length() > 5)
{
JOptionPane.showMessageDialog(frame, "Please enter a five digit number!");
System.exit(0);
}
else if(BookID.getText().length() < 5)
{
JOptionPane.showMessageDialog(frame, "Please enter a five digit number!");
System.exit(0);
}
//Converts text user entered to int and assigns to quantityOfBook
quantityOfBook = Integer.parseInt(Quantity.getText());
//Checks if the bookID entered by the user matches the bookID from array that was read from a file
for(int x = 0; x < numRows; x++)
{
for(int y = 0; y < 1; y++)
{
//Checks that the string s entered by the user matches an item in the 2D array
if(array2DFromFile[x][0].equals(s))
{
if(quantityOfBook <= 4)
{
discount = ("0");
BookInformation.setText(array2DFromFile[x][0] + array2DFromFile[x][1] + array2DFromFile[x][2] +" " +quantityOfBook+" " + discount +"% "+ array2DFromFile[x][2]);
}
else if(quantityOfBook >= 5 && quantityOfBook <= 9)
{
discount = ("10");
BookInformation.setText(array2DFromFile[x][0] + array2DFromFile[x][1] + array2DFromFile[x][2] +" " +quantityOfBook+" " +discount +"% " +array2DFromFile[x][2]);
}
else if(quantityOfBook >= 10 && quantityOfBook <= 14)
{
discount = ("15");
BookInformation.setText(array2DFromFile[x][0] + array2DFromFile[x][1] + array2DFromFile[x][2] +" " +quantityOfBook+" " +discount+"% " +array2DFromFile[x][2]);
}
else if(quantityOfBook >= 15)
{
discount = ("20");
BookInformation.setText(array2DFromFile[x][0] + array2DFromFile[x][1] + array2DFromFile[x][2] +" " +quantityOfBook+" "+ discount+"% " +array2DFromFile[x][2]);
}
if(arg0.getSource() == btnProcessItem)
{
btnProcessItem.setEnabled(false);
if(btnProcessItem.isEnabled()==false)
{
btnConfirmItem.setEnabled(true);
count1++;
if(count1 == numOfItems)
{
BookID.setEditable(false);
Quantity.setEditable(false);
lblQuantity.setVisible(false);
lblBookID.setVisible(false);
btnProcessItem.setText("Process Item");
btnProcessItem.setEnabled(false);
}
}
}
}
}
}
if (!list.contains(s))
{
JOptionPane.showMessageDialog(frame, "BookID "+ s + " does not exist.");
BookID.setText("");
Quantity.setText("");
if(arg0.getSource() == btnProcessItem)
{
btnProcessItem.setEnabled(true);
}
}
}
}
});
btnConfirmItem = new JButton("Confirm item #" + count);
btnConfirmItem.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent arg0)
{
btnViewOrder.setEnabled(true);
btnFinishOrder.setEnabled(true);
btnConfirmItem.setEnabled(false);
btnProcessItem.setEnabled(true);
s = BookID.getText();
for(int x = 0; x < numRows; x++)
{
for(int y = 0; y < 1; y++)
{
if(array2DFromFile[x][0].equals(s))
{
JOptionPane.showMessageDialog(frame, "Item # " +count+ " accepted");
BookID.setText("");
Quantity.setText("");
subTotal = Summation.subTotal(array2DFromFile[x][2], quantityOfBook);
subTotalforItems += subTotal;
String number = Double.toString(subTotalforItems);
subTotalCount++;
lblSubtotal.setText("Order Subtotal for " +subTotalCount+ " item(s):");
Subtotal.setText("$" +number);
StringBuilder builder = new StringBuilder();
BookInformation.setText(array2DFromFile[x][0] + array2DFromFile[x][1] + array2DFromFile[x][2] + " " +quantityOfBook + " "+ discount + " "+subTotal);
builder.append(array2DFromFile[x][0]).append(", ").append(array2DFromFile[x][1]).append(", ").append("$").append(array2DFromFile[x][2]).append(", ").append(quantityOfBook).append(", ").append(discount).append(", ").append("$").append(subTotal);
result = builder.toString();
itemsForTransactionFile.add(result);
}
}
}
text = BookInformation.getText();
cart.add(text);
numberList = Integer.toString(count);
numbers.add(numberList);
count = Integer.parseInt(numberList);
count++;
lblBookID.setText("Enter BookID for item #" + count +":");
lblQuantity.setText("Enter quantity for item #" + count + ":");
btnProcessItem.setText("Process Item # " + count);
btnConfirmItem.setText("Confirm item #" + count);
if(count1 == numOfItems)
{
btnProcessItem.setText("Process Item");
btnProcessItem.setEnabled(false);
btnConfirmItem.setText("Confirm Item");
}
}
});
btnConfirmItem.setBounds(186, 337, 131, 25);
frame.getContentPane().add(btnConfirmItem);
btnConfirmItem.setEnabled(false);
btnViewOrder = new JButton("View Order");
btnViewOrder.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent arg0)
{
for(int i =0; i { everything = cart.get(i).toString(); String everything2 = numbers.get(i).toString(); ViewOrderOutput += everything2 +". " + " " +everything+ " "; } JOptionPane.showMessageDialog(frame, ViewOrderOutput); } }); btnViewOrder.setBounds(329, 337, 131, 25); frame.getContentPane().add(btnViewOrder); btnViewOrder.setEnabled(false); btnFinishOrder = new JButton("Finish Order"); btnFinishOrder.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { double TaxAmount = subTotalforItems * .06; double orderTotal = TaxAmount + subTotalforItems; Date today = new Date(); SimpleDateFormat DATE_FORMAT = new SimpleDateFormat("yyMMddHHmmSS"); String date = DATE_FORMAT.format(today); DateTimeFormatter dtf = DateTimeFormatter.ofPattern("MM/dd/yyyy HH:mm:ss"); LocalDateTime now = LocalDateTime.now(); JOptionPane.showMessageDialog(frame, dtf.format(now) + " Number of line items: " + count1 + " Item# / ID / Title / Price / Qty / Disc % / Subtotal: " + " "+ ViewOrderOutput +" Order Subtotal: $" + subTotalforItems + " Tax Rate: 6% "+ "Tax Amount:\t $" + TaxAmount + " Order total:\t $" +orderTotal +" Thanks for shopping at the Ye Olde Book Shoppe!"); ArrayList for(int x = 0; x < itemsForTransactionFile.size(); x++) { StringBuilder builder = new StringBuilder(); String result = null; builder.append(date).append(", ").append(itemsForTransactionFile.get(x)).append(", ").append(now); result = builder.toString(); newlist.add(result); } String []results = newlist.toArray(new String[newlist.size()]); //Writes to transaction file /*BufferedWriter outputWriter = null; try { outputWriter = new BufferedWriter(new FileWriter("transaction.txt")); for(int x = 0; x < results.length; x++) { outputWriter.write(results[x]); outputWriter.newLine(); } outputWriter.flush(); outputWriter.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); }*/ try { File file = new File("transaction.txt"); if(!file.exists()) { file.createNewFile(); } FileWriter fw = new FileWriter(file, true); BufferedWriter bw = new BufferedWriter(fw); for(int x = 0; x < results.length; x++) { bw.write(results[x]); bw.newLine(); } bw.close(); } catch(IOException ioe) { ioe.printStackTrace(); } } }); btnFinishOrder.setBounds(472, 337, 131, 25); frame.getContentPane().add(btnFinishOrder); btnFinishOrder.setEnabled(false); JButton btnNewOrder = new JButton("New Order"); btnNewOrder.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { numOfItems = 0; quantityOfBook = 0; count = 1; subTotalCount = 0; subTotalforItems = 0; array2DFromFile = null; arrayFromFile = null; array2 = null; numRows = 0; numCols = 0; count1 = 0; bookIDProc = 0; subTotal = 0; everything = null; ViewOrderOutput=""; FinishOrderOutput=""; text = null; numberList = null; s = null; result = null; discount = null; } }); btnNewOrder.setBounds(615, 337, 131, 25); frame.getContentPane().add(btnNewOrder); /*This JButton will exit the system when the user clicks it*/ JButton btnExit = new JButton("Exit"); btnExit.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { if(e.getSource() == btnExit) System.exit(0); } }); btnExit.setBounds(758, 337, 131, 25); frame.getContentPane().add(btnExit); } } Here is the inventory.txt file which is read from 11111, "Top Secret Twenty One - Janet Evanovich", 8.99 22222, "W Is For Wasted - Sue Grafton", 9.95 33333, "Gray Mountain - John Grisham", 14.95 39872, "The Whistler - John Grisham", 12.50 44444, "Revival - Stephen King", 12.95 55555, "Everyday Italian - Giada de Laurentiis", 18.99 66666, "Refusal - Felix Francis", 7.99 77777, "Dust - Patricia Cornwell", 12.99 89124, "Into the Water - Paula Hawkins", 14.99 19342, "The Surgeon - Tess Gerritsen", 10.99 69843, "CyberStorm - Matthew Mather", 10.50 69999, "Cross the Line - James Patterson", 9.50 88888, "Terminal City - Linda Fairstein", 10.95 99999, "Rogue Lawyer - John Grisham", 15.95 11112, "The Guilty - David Baldacci", 14.95 11234, "Tricky Twenty-Two - Janet Evanovich", 12.95 23456, "14th Deadly Sin - James Patterson", 14.95 43786, "The Girl on the Train - Paula Hawkins", 9.99 23411, "Chaos - Patricia Cornwell", 10.75 12123, "X - Sue Grafton", 9.99 44556, "The Fix - David Baldacci", 12.99 33214, "Against All Odds - Danielle Steele", 18.99 98342, "The Circle - Dave Eggers", 14.99 67534, "The Black Book - James Patterson", 15.99 89891, "Turbo Twenty-three - Janet Evanovich", 8.99 45376, "The Apprentice - Tess Gerritsen", 9.99 12277, "Y Is For Yesterday - Sue Grafton", 10.99 The following code works. I have the latest java developer packages and such. I am using eclispe to code. All and any help would be appreciated.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
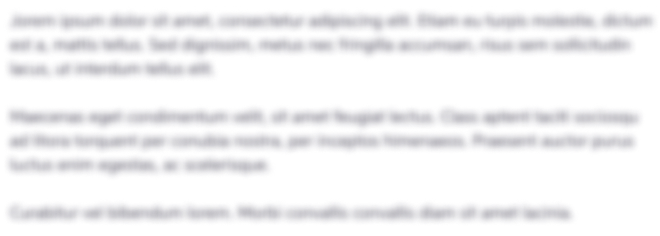
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started