Question
I need part 4 of this assignment done. I have already done parts 1-3 and the code for that is given below. Feel free to
I need part 4 of this assignment done. I have already done parts 1-3 and the code for that is given below. Feel free to change anything. Thank you, will upvote.
Code that I have completed for parts 1-3:
////////////////////////////////////////////Bucket.java/////////////////////////////////////////////////////////////////////////////////////
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.Scanner;
public class Bucket {
// start char of the bucket
private char minInitial;
// end char of the bucket
private char maxInitial;
// list holds all the words in one particular bucket
private LinkedList
// outputfile to store the results
private static File outfile = null;
// Bucket constructor.
public Bucket(char min, char max) {
this.maxInitial = max;
this.minInitial = min;
// initializes a new LinkedList to hold words for that bucket
list = new LinkedList
}
public static void main(String args[]) {
// test sorting of Integer array
sortIntegerList();
Scanner sc = new Scanner(System.in);
System.out.println("Enter file name to read data from");
String inputFileName = sc.nextLine();
System.out.println("Enter file name to write data to");
String outputFileName = sc.nextLine();
createOutputFile(outputFileName);
String[] wordsArray = null;
ArrayList
buckets.add(new Bucket('a', 'b'));
buckets.add(new Bucket('c', 'c'));
buckets.add(new Bucket('d', 'f'));
buckets.add(new Bucket('g', 'k'));
buckets.add(new Bucket('l', 'o'));
buckets.add(new Bucket('p', 'r'));
buckets.add(new Bucket('s', 's'));
buckets.add(new Bucket('t', 'z'));
try {
BufferedReader br = new BufferedReader(new FileReader(inputFileName));
String line = "";
String demlimiter = ",";
while ((line = br.readLine()) != null) {
wordsArray = line.trim().replaceAll("\\s", "").toLowerCase().split(demlimiter);
}
br.close();
} catch (FileNotFoundException e) {
System.out.println("Input file not found" + e.getMessage());
} catch (IOException e) {
System.out.println(e.getMessage());
}
// add words to the correct bucket for sorting
addToBucket(wordsArray, buckets);
// sort the strings in the bucket
sortStringBuckets(outputFileName, buckets);
sc.close();
}
// Creates an integer array with some values and sorts them with Arrays.sort
// Prints the value of array before and after the sorting
private static void sortIntegerList() {
int[] intList = { 23, 455, 66, 2, 56, 1 };
System.out.println("Integer list before sorting");
for (int i : intList) {
System.out.println(i);
}
Arrays.sort(intList);
System.out.println("Integer list after sorting");
for (int i : intList) {
System.out.println(i);
}
}
// Creates an output file for storing the result. FOr each inputfile a new file
// is created.Before starting it checks if the file already exists it delets the
// old one
// and creates a new file (for clean start)
private static void createOutputFile(String outputFileName) {
outfile = new File(outputFileName);
// If already exists delete and create a new
if (outfile.exists()) {
outfile.delete();
try {
outfile.createNewFile();
} catch (IOException e) {
System.out.println("Output file not found" + e.getMessage());
}
}
}
// For every bucket it takes the linkedList
// list individually
private static void sortStringBuckets(String outputFileName, ArrayList
for (int i = 0; i
// get list for this bucket
LinkedList
// create array from linkedlist
String[] array = list.toArray(new String[list.size()]);
// sort the array
Arrays.sort(array);
// write to the output file
writeToFile(outputFileName, buckets, i, array);
}
System.out.println("Writting to the file is done!!");
}
// Write sorted List to the output file .
private static void writeToFile(String outputFileName, ArrayList
BufferedWriter out = null;
try {
out = new BufferedWriter(new FileWriter(outfile, true));
for (int j = 0; j
// if this is the last element of all the buckets then no need to add comma in
// the end
if (i == buckets.size() - 1 && j == array.length - 1) {
out.write(array[j]);
} else {
out.write(array[j] + ",");
}
}
} catch (IOException e1) {
System.out.println("Writting to file failed." + e1.getMessage());
} finally {
try {
// close outputBuffer
out.close();
} catch (IOException e) {
System.out.println("Output buffer can't close." + e.getMessage());
}
}
}
// Get all the Strings obtained from the input file and put them in the correct
// bucket
private static void addToBucket(String[] wordsArray, ArrayList
for (int i = 0; i
// take one word at a time and find the correct bucket for it
String str = wordsArray[i];
char letter = str.toLowerCase().charAt(0);
if (letter == 'a' || letter == 'b') {
buckets.get(0).list.add(str);
} else if (letter == 'b') {
buckets.get(1).list.add(str);
} else if (letter >= 'd' && letter
buckets.get(2).list.add(str);
} else if (letter >= 'g' && letter
buckets.get(3).list.add(str);
} else if (letter >= 'l' && letter
buckets.get(4).list.add(str);
} else if (letter >= 'p' && letter
buckets.get(5).list.add(str);
} else if (letter == 's') {
buckets.get(6).list.add(str);
} else if (letter >= 't' && letter
buckets.get(7).list.add(str);
}
}
System.out.println("Adding to the bucket is done!!");
}
// return the linkedList
public LinkedList
return list;
}
}
//////////////////////////////////////////////////End Bucket.java//////////////////////////////////////////////////////////////////////////////
/////////////////////////////////////input.txt/////////////////////////////////////////////////////////////////////////////////////////////
In, Java ,we ,can, append, a ,string, in, an ,existing ,file, using ,FileWriter, which ,has, an, option ,to ,open, file, in, append, mode
////////////////////////////////end input.txt/////////////////////////////////////////////////////////////////////////////
In this assignment, you are asked to write a program that reads a series of strings sepa- ratod with comma from a given file. Your program needs to alphabetically sort the strings using bucket sort and write the result in the output file. The strings in the output must be separted with comma. The names and addresses of input and output files are given by the user through console at the beginning of the program. 1 Bucket Sort Bucket sort, or bin sort, is a sorting algorithm that works by distributing the elements into a number of buckets. Each bucket is then sorted individually. To sort each bucket in this assignment, you need to use the sort() method in jana util Arrags class. 0-9 10-19 20-29 30-39 40-4 29 25 3 49 9 37 21 43 37 43 49 29 25 3749 21 10-1, 20-29 x -19 404,-> 3 9 21 25 29 37 43 49 Figure I: Exumple of bucket sort for sorting mumbers 29, 25, 3, 19, 9, 37, 21, 13. Numbers distributod aumong bins (left image). Then, elements are sorted within each bin (right are image)Step by Step Solution
There are 3 Steps involved in it
Step: 1
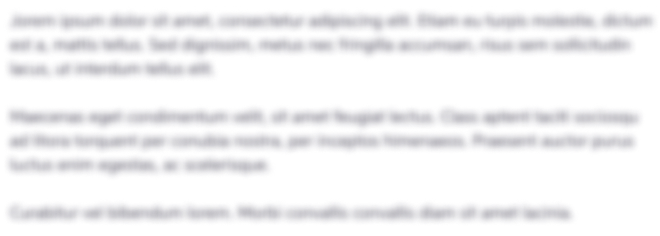
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started