Question
I need some assistance with a program. I can't seem to figure out why the program listed below keeps give me this compile issue. Exception
I need some assistance with a program. I can't seem to figure out why the program listed below keeps give me this compile issue.
Exception in thread "main" java.lang.NullPointerException at java.awt.Container.addImpl(Container.java:1093) at java.awt.Container.add(Container.java:417) at Test_Average.
7 8 import javax.swing.*; //Needed for JFrame and JTextField 9 import java.awt.*; //Needed for the class Container 10 import java.awt.event.*; //Needed for the events 11 import java.util.*; //import all the classes in a package at once 12 import java.math.*; //Contains class methods for performing basic numeric operations 13 14 public class Test_Average extends JFrame 15 { 16 17 private static final int WIDTH = 400; 18 private static final int HEIGHT = 175; 19 20 private JLabel t1L,t2L,t3L,t4L; 21 private JTextField t1TF,t2TF,t3TF,t4TF; 22 23 private JLabel w1L,w2L,w3L,w4L; 24 private JTextField w1TF,w2TF,w3TF,w4TF; 25 26 private JLabel avgL; 27 private JTextField avgTF; 28 29 private JButton calculateB,exitB; 30 31 private CalculateButtonHandler cbHandler; 32 private ExitButtonHandler ebHandler; 33 34 public Test_Average() 35 { 36 37 setTitle("Weighted Average"); 38 39 //test fields 40 // 1. What is a JLabel? Where is it in our textbook? A JLabel are objects of a particular class type. Page 340 chapter6 41 // 2. How do you create a JLabel? Where is it in our textbook? JLabel lengthL; lengthL= new JLabel("Enter the Length")page340 Chap.6 42 // 3. What does SwingConstants.RIGHT mean? Where is it in our textbook? They specify whether to set the string describing the label as left-justified, right-justified, or centered. Pg340 43 44 t1L = new JLabel("Test score 1:",SwingConstants.RIGHT); 45 t2L = new JLabel("Test score 2:",SwingConstants.RIGHT); 46 t3L = new JLabel("Test score 3:",SwingConstants.RIGHT); 47 t4L = new JLabel("Test score 4:",SwingConstants.RIGHT); 48 49 t1TF = new JTextField("0",5); 50 t1TF.setHorizontalAlignment(JTextField.CENTER); 51 t2TF = new JTextField("0",5); 52 t2TF.setHorizontalAlignment(JTextField.CENTER); 53 t3TF = new JTextField("0",5); 54 t3TF.setHorizontalAlignment(JTextField.CENTER); 55 t4TF = new JTextField("0",5); 56 t4TF.setHorizontalAlignment(JTextField.CENTER); 57 58 //avg fields 59 avgL = new JLabel("Average:",SwingConstants.RIGHT); 60 avgTF = new JTextField("0",5); 61 avgTF.setHorizontalAlignment(JTextField.CENTER); 62 63 //calc button 64 // 1. What is a JButton? Where is it in our textbook? a way to create objects belonging to the class JButton. PG.347 65 // 2. How do you create a JButton? Where is it in our textbook? 66 // Ex.JButton calculateB, 67 // exitB;calculateB = new JButton("Calculate"); 68 // exitB = new JButton("exit"); 69 // Page 347 70 // 3. What is a CalculateButtonHandler()? Where is it in our textbook? 71 // CalculateButtonHandler()= a way to provide the necessary code for the method actionPerformed. 72 // Page 349 73 // 4. How do you create a CalculateButtonHandler()? Where is it in our textbook? 74 // private class CalculateButtonHandler implements ActionListener 75 // { 76 // public void actionPerformed(ActionEvent e) 77 // { 78 // double width, length, area, perimeter; 79 // length 80 // = Double.parseDouble(lengthTF.getText()); 81 // width 82 // = Double.parseDouble(widthTF.getText()); 83 // area = length * width; 84 // parimeter = 2 * (length + width); 85 // areaTF.setText("" + area); 86 // perimeterTF.setText("" + perimeter); 87 // } 88 // } 89 // page 349/350 90 // 5. What is an ActionListener? Where is it in our textbook? 91 // ActionListener handles the action event Page 349 92 93 calculateB = new JButton("Calculate"); 94 cbHandler = new CalculateButtonHandler(); 95 calculateB.addActionListener(cbHandler); 96 97 //exit button 98 99 exitB = new JButton("Exit"); 100 ebHandler = new ExitButtonHandler(); 101 exitB.addActionListener(ebHandler); 102 103 Container pane = getContentPane(); 104 105 pane.setLayout(new GridLayout(5,4)); 106 107 pane.add(t1L); 108 pane.add(t1TF); 109 110 pane.add(w1L); 111 pane.add(w1TF); 112 113 pane.add(t2L); 114 pane.add(t2TF); 115 116 pane.add(w2L); 117 pane.add(w2TF); 118 119 pane.add(t3L); 120 pane.add(t3TF); 121 122 pane.add(w3L); 123 pane.add(t3TF); 124 125 pane.add(t4L); 126 pane.add(t4TF); 127 128 pane.add(w4L); 129 pane.add(w4TF); 130 131 //buttons 132 pane.add(calculateB); 133 pane.add(exitB); 134 135 //avg 136 pane.add(avgL); 137 pane.add(avgTF); 138 139 setSize(WIDTH,HEIGHT); 140 setVisible(true); 141 setDefaultCloseOperation(EXIT_ON_CLOSE); 142 } 143 144 private class CalculateButtonHandler implements ActionListener 145 146 { 147 148 public void actionPerformed(ActionEvent e) 149 150 { 151 152 //declare variables 153 154 double test1, test2, test3, test4; 155 156 double weight1, weight2, weight3, weight4; 157 158 double avg1, avg2, avg3, avg4; 159 160 double favg; 161 162 //input 163 164 test1 = Double.parseDouble(t1TF.getText()); 165 166 test2 = Double.parseDouble(t2TF.getText()); 167 168 test3 = Double.parseDouble(t3TF.getText()); 169 170 test4 = Double.parseDouble(t4TF.getText()); 171 172 weight1 = Double.parseDouble(w1TF.getText()); 173 174 weight2 = Double.parseDouble(w2TF.getText()); 175 176 weight3 = Double.parseDouble(w3TF.getText()); 177 178 weight4 = Double.parseDouble(w4TF.getText()); 179 180 //process 181 182 avg1 = test1*weight1; 183 184 avg2 = test2*weight2; 185 186 avg3 = test3*weight3; 187 188 avg4 = test4*weight4; 189 190 favg = avg1+avg2+avg3+avg4; 191 192 //output 193 194 avgTF.setText(""+String.format("%.2f",favg)); 195 } 196 } 197 198 private class ExitButtonHandler implements ActionListener 199 { 200 201 public void actionPerformed(ActionEvent e) 202 { 203 204 System.exit(0); 205 } 206 } 207 208 public static void main(String[]args) 209 { 210 211 Test_Average rectObject = new Test_Average(); 212 } 213 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
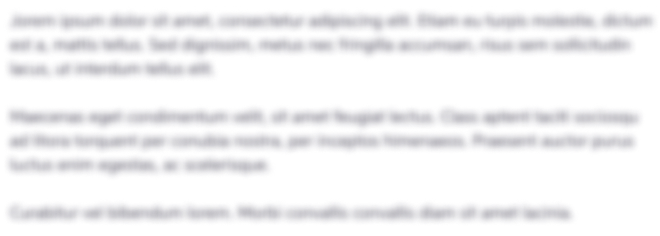
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started