Question
I need some help coding this file. The Student class represents a single student, their maximum course load, and a list of courses the student
I need some help coding this file. The Student class represents a single student, their maximum course load, and a list of courses the student would like (in order of preference, most-preferred-to-least). I have included the tests. Thank you!
package scheduler;
import java.util.List;
/**
* A class representing a student.
*
* @author liberato
*
*/
public class Student {
/**
*
* Instantiates a new Student object. The student's maximum course load must be greater
* than zero, and the preferences list must contain at least one course.
*
* The preference list is copied into this Student object.
*
* @param name the student's name
* @param maxCourses the maximum number of courses that can be on this student's schedule
* @param preferences the student's ordered list of preferred courses
* @throws IllegalArgumentException thrown if the maxCourses or preferences are invalid
*/
public Student(String name, int maxCourses, List
}
/**
*
* @return the student's name
*/
public String getName() {
return null;
}
/**
*
* @return the student's max course load
*/
public int getMaxCourses() {
return -1;
}
/**
* Returns the student's list of course preferences, ordered from most- to least-desired.
*
* This returned object does not share state with the internal state of the Student.
*
* @return the student's preference list
*/
public List
return null;
}
/**
* Returns the student's current schedule.
*
* This returned object does not share state with the internal state of the Student.
*
* @return the student's schedule
*/
public List
return null;
}
}
StudentTest.java
package scheduler;
import org.junit.Test;
import static org.junit.Assert.*;
import java.util.ArrayList; import java.util.List;
import org.junit.Before; import org.junit.Rule; import org.junit.Test; import org.junit.rules.Timeout;
public class StudentTest {
// uncomment the following if you're trying to diagnose an infinite loop in a test // @Rule // public Timeout globalTimeout = Timeout.seconds(10); // 10 seconds
private Student joe; private List
@Test public void testGetMaxCourses() { assertEquals(4, joe.getMaxCourses()); }
@Test public void testGetPreferences() { assertEquals(courseList, joe.getPreferences()); }
@Test public void testGetPreferencesNotShared() { List
@Test public void testGetScheduleEmptyNotShared() { List
@Test(expected = IllegalArgumentException.class) public void testInvalidMaxCourses() { Student s = new Student("NoCourses", 0, courseList); }
@Test(expected = IllegalArgumentException.class) public void testEmptyPreferences() { Student s = new Student("DontCare", 3, new ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
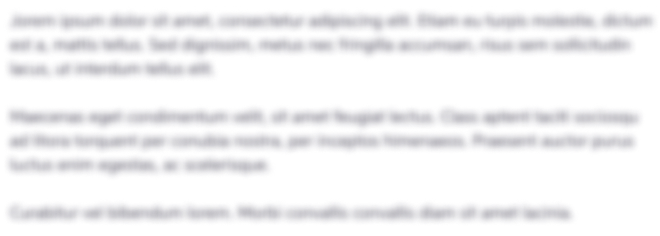
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started