Question
I need the C++ code for this programming project. Part 1: [60 points total] Create a program to (1) shuffle, deal, and display a deck
I need the C++ code for this programming project.
Part 1: [60 points total]
Create a program to (1) shuffle, deal, and display a deck of cards; and (2) shuffle, deal and display a five-card poker hand. When the program begins, the user should be prompted as follows:
Enter: D or d to shuffle, deal, and display the deck P or p to shuffle, deal, and display a five-card poker hand Q or q to quit
The program should loop, prompting the user, until the user enters a Q or q [5 points].
Create a class Card, class DeckOfCards, and a driver program.
Class Card should provide the following [20 points]:
Data members face and suit (use enumerations to represent the faces and suits).
A constructor that receives two enumeration constants representing the face and suit and uses them to initialize the data members.
Two static arrays of strings representing the faces and suits.
A toString function that returns the Card as a string in the form face of suit. You can use the + operator to concatenate strings.
Class DeckOfCards should contain [20 points]:
An array of Cards named deck to store the Cards.
An integer currentCard representing the next card to deal.
A default constructor that initializes the Cards in the deck.
A shuffle function that shuffles the cards in the deck. The shuffle algorithm should iterate through the array of Cards. For each card, randomly select another Card in the deck and swap the two Cards.
A dealCard function that returns the next card object from the deck.
A moreCards function that returns a bool value indicating whether there are more Cards to deal.
The driver program should create a DeckOfCards object, shuffle the cards, then deal the 52 cards
and display them; the output should be similar to the following, i.e., four columns with thirteen cards in each column [15 points] (this code executes when the user enters a D or d):
King of Clubs
Jack of Hearts
Six of Spades
Three of Diamonds
Four of Spades
Three of Clubs
Queen of Clubs
Three of Spades
Ace of Spades
Deuce of Spades
Ace of Diamonds
Queen of Hearts
Five of Diamonds
Ten of Clubs
Queen of Diamonds
Seven of Spades
Eight of Hearts
Four of Diamonds
King of Diamonds
Eight of Diamonds
Ten of Hearts
Deuce of Hearts
Ace of Clubs
Deuce of Clubs
Seven of Clubs
Eight of Spades
Nine of Hearts
King of Hearts
Ten of Spades
Four of Hearts
Jack of Diamonds
Six of Diamonds
Ten of Diamonds
Six of Hearts
Eight of Clubs
Queen of Spades
Nine of Diamonds
King of Spades
Jack of Clubs
Deuce of Diamonds
Nine of Clubs
Seven of Hearts
Five of Hearts
Four of Clubs
Five of Clubs
Jack of Spades
Six of Clubs
Nine of Spades
Ace of Hearts
Seven of Diamonds
Five of Spades
Three of Hearts
Part 2: [40 points total]
The program should also deal a five-card poker hand (this code executes when the user enters a P or p).
Create a class Hand, which should provide the following [30 points]:
Data members hand and faceCount to contain the poker hand and the count of each face in the hand.
A constructor that receives references to the deck and faceCount, deals the hand, and counts each face.
A function that displays the contents of the hand in a format similar to:
Hand is:
Nine of Hearts King of Spades King of Hearts Eight of Hearts Deuce of Hearts
Separate functions to determine whether the hand contains the following:
A pair
Two pairs
Three of a kind (e.g., three Jacks)
Four of a kind (e.g., four Aces)
A flush (i.e., all five card of the same suit)
A straight (i.e., five cards of consecutive face values; Ace may be low or high)
The driver program should create a DeckOfCards object, shuffle the cards, invoke functions in b, c, and d above, and display the highest-ranking combination in the hand (i.e., one of the following) or nothing. [10 points]
Hand contains four of a kind
Hand contains a straight flush (i.e., a straight and also a flush)
Hand contains a full house (i.e., three of a kind and also a pair)
Hand contains a flush
Hand contains a straight
Hand contains three of a kind
Hand contains two pairs
Hand contains a pair
Your total output for a five-card poker hand should look similar to the following:
Hand is:
Nine of Hearts King of Spades King of Hearts Eight of Hearts Deuce of Hearts
Hand contains a pair
NOTE: the remaining 9 points will be allocated for documenting the code, i.e., adding comments that make the code easy to read/understand.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
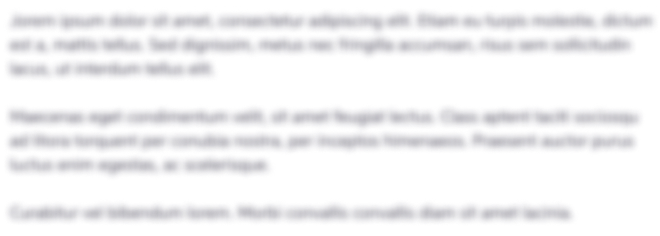
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started