I need the pseudo code this source code in Java import java.util.Scanner; public class Client { public static void displayChange(Change customer[], int coinAmount) { Scanner
I need the pseudo code this source code in Java
import java.util.Scanner;
public class Client { public static void displayChange(Change customer[], int coinAmount) {
Scanner keyboard = new Scanner(System.in);
int counter; // store the denomination type in array int denom[] = new int[4]; // set boolean boolean not_found = true; // prompt user to enter the name System.out.print(" Please enter the customer's name that you would like to display: "); String name = keyboard.next();
// loop to check for the breakdown of the denomination for (counter = 0; counter < coinAmount; counter++) { if (customer[counter].getName().equalsIgnoreCase(name)) { denom = customer[counter].coinchangecalculate();
// display the count for denomination type System.out.println(" Customer: " + customer[counter].getName() + " " + customer[counter].getCoinChangeAmount() + " cent "); System.out.println("Change:"); if (denom[0] != 0) System.out.println("50 cent: " + denom[0]); if (denom[1] != 0) System.out.println("20 cent: " + denom[1]); if (denom[2] != 0) System.out.println("10 cent: " + denom[2]); if (denom[3] != 0) System.out.println("5 cent: " + denom[3]);
not_found = false; break; } }
// error message when the customer's name is not found if (not_found) System.out.println("Name: " + name + " Not found"); }
public static void displaySmallest(Change customer[], int coinAmount) { // store the denomination type in array int denom[] = new int[4]; int counter; // smallest coin amount int min = customer[0].getCoinChangeAmount(); // index of array int index = 0; // loop to check for the smallest coin amount for (counter = 1; counter < coinAmount; counter++) { if (customer[counter].getCoinChangeAmount() < min) { min = customer[counter].getCoinChangeAmount(); index = counter; } }
denom = customer[index].coinchangecalculate(); // display the count for denomination type System.out.println(" Customer: " + customer[index].getName() + " " + customer[index].getCoinChangeAmount() + " cent "); System.out.println("Change:"); if (denom[0] != 0) System.out.println("50 cent: " + denom[0]); if (denom[1] != 0) System.out.println("20 cent: " + denom[1]); if (denom[2] != 0) System.out.println("10 cent: " + denom[2]); if (denom[3] != 0) System.out.println("5 cent: " + denom[3]); }
public static void displayLargest(Change customer[], int coinAmount) { // store the denomination type in array int denom[] = new int[4];
int counter;
// largest coin amount int max = customer[0].getCoinChangeAmount();
// index of array int index = 0;
for (counter = 1; counter < coinAmount; counter++) { if (customer[counter].getCoinChangeAmount() > max) { max = customer[counter].getCoinChangeAmount(); index = counter; } }
denom = customer[index].coinchangecalculate(); // display the count for denomination type System.out.println(" Customer: " + customer[index].getName() + " " + customer[index].getCoinChangeAmount() + " cent "); System.out.println("Change:"); if (denom[0] != 0) System.out.println("50 cent: " + denom[0]); if (denom[1] != 0) System.out.println("20 cent: " + denom[1]); if (denom[2] != 0) System.out.println("10 cent: " + denom[2]); if (denom[3] != 0) System.out.println("5 cent: " + denom[3]); }
public static void displayTotal(Change customer[], int coinAmount) { // store the denomination type in array int denom[] = new int[4]; // store the total denomination type in array int tot_denom[] = new int[4]; int totalLargest = 0; int largest = 0; int sumLargest = 0;
int counter, counter2; // loop to find largest value for the denomination type for (counter = 0; counter < coinAmount; counter++) { denom = customer[counter].coinchangecalculate();
for (counter2 = 0; counter2 < 4; counter2++) tot_denom[counter2] = tot_denom[counter2] + denom[counter2]; }
for (counter = 0; counter < 4; counter++) totalLargest = totalLargest + tot_denom[counter];
for (counter = 0; counter < 4; counter++) { if (largest < tot_denom[counter]) { largest = tot_denom[counter]; } }
System.out.println(" The largest number of coin denomination is:"); // find which index is the largest, sum and display if (tot_denom[0] >= largest) { sumLargest += tot_denom[0]; System.out.println("50 cent: " + tot_denom[0]); }
if (tot_denom[1] >= largest) { sumLargest += tot_denom[1]; System.out.println("20 cent: " + tot_denom[1]); }
if (tot_denom[2] >= largest) { sumLargest += tot_denom[2]; System.out.println("10 cent: " + tot_denom[2]); }
if (tot_denom[3] >= largest) { sumLargest += tot_denom[3]; System.out.println("5 cent: " + tot_denom[3]); }
System.out.println(" Total number of the coin: " + sumLargest); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
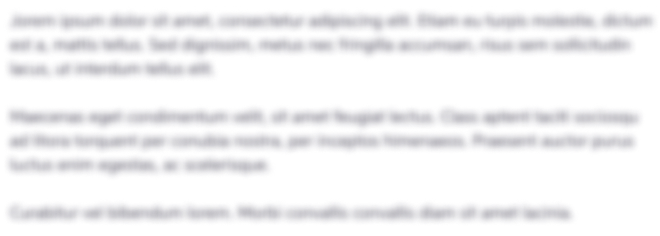
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started