Question
I need to convert this java program to C or C++ program Student class public class student { public String name; public int time; //
I need to convert this java program to C or C++ program
Student class
public class student {
public String name;
public int time;
// constructor
public student(String name, int time) {
this.name = name;
this.time = time;
}
}
Professor java
import java.util.ArrayList;
public class professor {
//creating Queue to store students
ArrayList
// constructor
public professor(){
}
// adding student to list
public void addList(student obj){
if(list.size() < 5){
list.add(obj);
System.out.println("New student object stored into list.");
}
else{
System.out.println("List is full. Professor playing Halo");
}
}
// remove student object from list
public void removeList(){
try {
//assuming it takes 5 secs to complete the task
Thread.sleep(5000);
if(list.size() > 0){
list.remove(list.size()-1);
System.out.println("Student removed from list.");
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Studentscheduler class
import java.util.Timer;
import java.util.TimerTask;
public class studentscheduler extends TimerTask{
// professor object
professor profQueue = new professor();
int count = 1;
@Override
public void run() {
// creating student object
student obj = new student("Ram"+Integer.toString(count),15);
// storing into list
profQueue.addList(obj);
// removing from list
profQueue.removeList();
}
// main method
public static void main(String args[]){
studentscheduler studentScheduler = new studentscheduler();
// creating timer thread
Timer timer = new Timer(true);
// starting scheduler
timer.scheduleAtFixedRate(studentScheduler, 0, 10*2000);
System.out.println("Student scheduler started");
//explicitly cancelling adter some time
try {
Thread.sleep(100000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// cancelling timer
timer.cancel();
System.out.println("Student scheduler cancelled");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
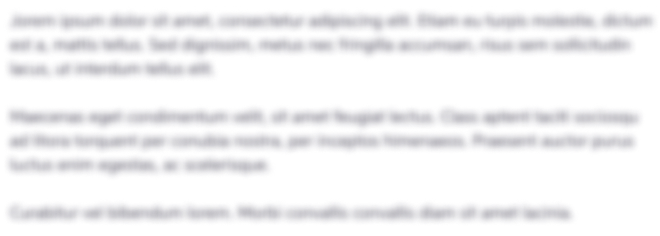
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started