Question
I need to document the work!! Look at my Customer class. I have added JavaDocs for the class, each field and each method. I need
I need to document the work!! Look at my Customer class. I have added JavaDocs for the class, each field and each method. I need do the same for the Sales class. In production, this is required as the standard documentation for every class.
Then generate the JavaDocs.
import java.io.*;
/**
* Customer class
* used to describe a Customer object
*
**/
public class Customer implements Comparable, Serializable {
// three private fields
/**
* used to hold the Customer's last name
*/
private String last;
/**
* used to hold the Customer's first name
*/
private String first;
/**
* a unique Customer id - generated by the program
*/
private int id;
/**
* a static variable used to make Customer numbers unique
*/
public static int num=200;
/**
* Full Constructor - used to creat a new customer
* Creates a new unique id for them
* @param custLast - Customer last name
* @param custFirst - Customer first name
*/
public Customer(String custLast, String custFirst)
{
last=custLast;
first=custFirst;
id = num;
num++;
}
/**
* empty constructor but still creates a unique id for them
*/
public Customer()
{
id = num;
num++;
}
/**
* used for reading back from a file where id is already set
* read the id - do not create a new one
* @param custLast - Customer last name
* @param custFirst - Customer first name
* @param idNum - Customer ID
*/
public Customer(String custLast, String custFirst, int idNum)
{
last=custLast;
first=custFirst;
id = idNum;
}
/**
* used to convert the object to a String output
* @see java.lang.Object#toString()
*/
public String toString()
{
return first + " " + last +",Id=" + id;
}
/**
* used for writing to a file
* @return String
*/
public String toStringF()
{
return first + "|" + last +"|" + id;
}
/**
* used to define how to compare two Customer objects
* @see java.lang.Comparable#compareTo(java.lang.Object)
*
**/
public int compareTo(Object o)
{
Customer c = (Customer) o;
if ((c.getLast() + c.getFirst()).compareTo( getLast() + getFirst())>0)
return 1;
else
return -1;
}
/**
* used to get the id
* @return int that is the customer number
*/
public static int getNum() {
return num;
}
/**
* used to get the first name
* @return a String that is the first name
*/
public String getFirst() {
return first;
}
/**
* used to get the id
* @return an int that is the id
*/
public int getId() {
return id;
}
/**
* used to get the last name
* @return a String that is the last name
*/
public String getLast() {
return last;
}
/**
* used to set the static next number value
* You could write the setter or make the variable public above
* @param int - the number to set
*/
public static void setNum(int i) {
num = i;
}
/**
* used to set the first name
* @param String - the first name
*/
public void setFirst(String string) {
first = string;
}
/**
* used to set the id
* @param int - that is the id
*/
public void setId(int i) {
id = i;
}
/**
* used to set the last name
* @param string that is the last name
*/
public void setLast(String string) {
last = string;
}
}
public class Sales {
private Customer cust;
private Inventory inv;
private int numBought;
public Sales()
{
}
public Sales (Customer c, Inventory i, int n)
{
cust = c;
inv = i;
numBought = n;
}
public String toString()
{
return cust.toString() + " bought " + numBought + " "+ inv.toStringC();
}
public Customer getCust() {
return cust;
}
public Inventory getInv() {
return inv;
}
public int getNumBought() {
return numBought;
}
public void setCust(Customer customer) {
cust = customer;
}
public void setInv(Inventory inventory) {
inv = inventory;
}
public void setNumBought(int i) {
numBought = i;
}
}
public class Inventory {
private String itemName;
private int numInStock;
private double cost;
private double salesPrice;
private double profitOnStock;
public Inventory()
{
}
public Inventory(String i, int n, double c, double s)
{
itemName=i;
numInStock = n;
cost = c;
salesPrice = s;
profit();
}
public String toString()
{
String s= "There are " + numInStock + " " + itemName + " in stock";
s = s + " Cost: " + cost + " SalesPrice: " + salesPrice + " Profit available: " + profitOnStock;
return s;
}
public String toStringC()
{
return itemName + " Cost:" + salesPrice;
}
public double costValue()
{
return numInStock * cost;
}
public double salesValue()
{
return numInStock * salesPrice;
}
public void profit()
{
double cost = costValue();
double sales = salesValue();
profitOnStock = sales - cost;
}
/**
* @return
*/
public double getCost() {
return cost;
}
/**
* @return
*/
public String getItemName() {
return itemName;
}
/**
* @return
*/
public int getNumInStock() {
return numInStock;
}
/**
* @return
*/
public double getSalesPrice() {
return salesPrice;
}
/**
* @param d
*/
public void setCost(double d) {
cost = d;
}
/**
* @param string
*/
public void setItemName(String string) {
itemName = string;
}
/**
* @param i
*/
public void setNumInStock(int i) {
numInStock = i;
}
/**
* @param d
*/
public void setSalesPrice(double d) {
salesPrice = d;
}
}
import java.util.*;
public class SalesDriver {
public static void main(String[] args) {
ArrayList cust = new ArrayList();
ArrayList inv = new ArrayList();
ArrayList sales = new ArrayList();
// load the data
loadData(cust, inv);
Scanner scan = new Scanner(System.in);
boolean more = true;
while (more) {
System.out.println("Let's make a sale ");
System.out.println("Customers:");
for (int i=0;i
System.out.println(i+1 + " " + cust.get(i).toString());
System.out.println(" Which customer number?");
int numC = scan.nextInt();
numC = numC-1;
System.out.println(" Inventory items:");
for (int i=0;i
System.out.println(i+1 + " " + inv.get(i).toString());
System.out.println(" Which inventory number?");
int numI = scan.nextInt();
numI = numI-1;
System.out.println (" How many would you like?");
int numBought = scan.nextInt();
if (numBought > inv.get(numI).getNumInStock())
{
System.out.println("There are not that many in stock. Sale cancelled!!");
}
else
{
System.out.println("Sold! The sale has been recorded");
Sales s = new Sales(cust.get(numC),inv.get(numI), numBought);
sales.add(s);
Inventory inven = inv.get(numI);
int newNum = inven.getNumInStock()-numBought;
inven.setNumInStock(newNum);
}
System.out.println (" More sales? (true/false)");
more = scan.nextBoolean();
}
printSales(sales);
}
public static void printSales(ArrayList s) {
for (int i=0;i
System.out.println(s.get(i).toString());
}
public static void loadData(ArrayList c, ArrayList inv) {
c.add(new Customer("Mouse", "Mickey"));
c.add(new Customer("Duck", "Donald"));
inv.add(new Inventory("Widgets", 100, 45.00, 55.00));
inv.add(new Inventory("Junk", 200, 13.35, 21.00));
}
}
The file needs to be saved as Sales.html
Step by Step Solution
There are 3 Steps involved in it
Step: 1
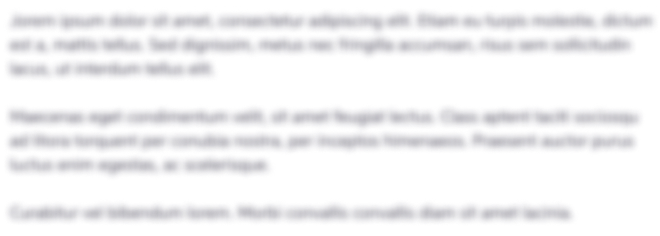
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started