Question
i need to have the graph class made according to the interface comments added to the methods so i can compare with the class i
i need to have the graph class made according to the interface comments added to the methods so i can compare with the class i made as it's not working and it would be a pain to just have you correct my thought process so i prefer to see how you do and how i went wrong. so you can copy and paste all classes without any issue as you will not need the interface as i kind included it in the class below so you can remove the implements associated with the class and edit the methods are is requested by the javadoc comments above the methods
import java.util.ArrayList; import java.util.HashMap; import java.util.Set; import java.util.TreeSet;
public class Graph implements GraphInterface { /** * Returns an edge connecting source vertex to target vertex if such * vertices and such edge exist in this graph. Otherwise returns * null. If any of the specified vertices is null * returns null * * In undirected graphs, the returned edge may have its source and target * vertices in the opposite order. * * @param sourceVertex source vertex of the edge. * @param destinationVertex target vertex of the edge. * * @return an edge connecting source vertex to target vertex. */ @Override public Road getEdge(Town sourceVertex, Town destinationVertex) { // TODO Auto-generated method stub return null; } /** * Creates a new edge in this graph, going from the source vertex to the * target vertex, and returns the created edge. * * The source and target vertices must already be contained in this * graph. If they are not found in graph IllegalArgumentException is * thrown. * * * @param sourceVertex source vertex of the edge. * @param destinationVertex target vertex of the edge. * @param weight weight of the edge * @param description description for edge * * @return The newly created edge if added to the graph, otherwise null. * * @throws IllegalArgumentException if source or target vertices are not * found in the graph. * @throws NullPointerException if any of the specified vertices is null. */ @Override public Road addEdge(Town sourceVertex, Town destinationVertex, int weight, String description) { // TODO Auto-generated method stub return null; } /** * Adds the specified vertex to this graph if not already present. More * formally, adds the specified vertex, v, to this graph if * this graph contains no vertex u such that * u.equals(v). If this graph already contains such vertex, the call * leaves this graph unchanged and returns false. In combination * with the restriction on constructors, this ensures that graphs never * contain duplicate vertices. * * @param v vertex to be added to this graph. * * @return true if this graph did not already contain the specified * vertex. * * @throws NullPointerException if the specified vertex is null. */ @Override public boolean addVertex(Town v) { // TODO Auto-generated method stub return false; } /** * Returns true if and only if this graph contains an edge going * from the source vertex to the target vertex. In undirected graphs the * same result is obtained when source and target are inverted. If any of * the specified vertices does not exist in the graph, or if is * null, returns false. * * @param sourceVertex source vertex of the edge. * @param destinationVertex target vertex of the edge. * * @return true if this graph contains the specified edge. */ @Override public boolean containsEdge(Town sourceVertex, Town destinationVertex) { // TODO Auto-generated method stub return false; } /** * Returns true if this graph contains the specified vertex. More * formally, returns true if and only if this graph contains a * vertex u such that u.equals(v). If the * specified vertex is null returns false. * * @param v vertex whose presence in this graph is to be tested. * * @return true if this graph contains the specified vertex. */ @Override public boolean containsVertex(Town v) { // TODO Auto-generated method stub return false; } /** * Returns a set of the edges contained in this graph. The set is backed by * the graph, so changes to the graph are reflected in the set. If the graph * is modified while an iteration over the set is in progress, the results * of the iteration are undefined. * * * @return a set of the edges contained in this graph. */ @Override public Set edgeSet() { // TODO Auto-generated method stub return null; } /** * Returns a set of all edges touching the specified vertex (also * referred to as adjacent vertices). If no edges are * touching the specified vertex returns an empty set. * * @param vertex the vertex for which a set of touching edges is to be * returned. * * @return a set of all edges touching the specified vertex. * * @throws IllegalArgumentException if vertex is not found in the graph. * @throws NullPointerException if vertex is null. */ @Override public Set edgesOf(Town vertex) { // TODO Auto-generated method stub return null; } /** * Removes an edge going from source vertex to target vertex, if such * vertices and such edge exist in this graph. * * If weight >- 1 it must be checked * If description != null, it must be checked * * Returns the edge if removed * or null otherwise. * * @param sourceVertex source vertex of the edge. * @param destinationVertex target vertex of the edge. * @param weight weight of the edge * @param description description of the edge * * @return The removed edge, or null if no edge removed. */ @Override public Road removeEdge(Town sourceVertex, Town destinationVertex, int weight, String description) { // TODO Auto-generated method stub return null; } /** * Removes the specified vertex from this graph including all its touching * edges if present. More formally, if the graph contains a vertex * u such that u.equals(v), the call removes all edges * that touch u and then removes u itself. If no * such u is found, the call leaves the graph unchanged. * Returns true if the graph contained the specified vertex. (The * graph will not contain the specified vertex once the call returns). * * If the specified vertex is null returns false. * * @param v vertex to be removed from this graph, if present. * * @return true if the graph contained the specified vertex; * false otherwise. */ @Override public boolean removeVertex(Town v) { // TODO Auto-generated method stub return false; } /** * Returns a set of the vertices contained in this graph. The set is backed * by the graph, so changes to the graph are reflected in the set. If the * graph is modified while an iteration over the set is in progress, the * results of the iteration are undefined. * * * @return a set view of the vertices contained in this graph. */ @Override public Set vertexSet() { // TODO Auto-generated method stub return null; } /** * Find the shortest path from the sourceVertex to the destinationVertex * call the dijkstraShortestPath with the sourceVertex * @param sourceVertex starting vertex * @param destinationVertex ending vertex * @return An arraylist of Strings that describe the path from sourceVertex * to destinationVertex */ @Override public ArrayList shortestPath(Town sourceVertex, Town destinationVertex) { // TODO Auto-generated method stub return null; } /** * Dijkstra's Shortest Path Method. Internal structures are built which * hold the ability to retrieve the path, shortest distance from the * sourceVertex to all the other vertices in the graph, etc. * @param sourceVertex the vertex to find shortest path from * */ @Override public void dijkstraShortestPath(Town sourceVertex) { // TODO Auto-generated method stub }
}
the classes you will need
road class
import java.util.ArrayList;
public class Road implements Comparable{ private Town endPoint1, endPoint2; private int distance;//distance between vertices private String name;
public Road(Town town, Town town2, int i, String string) { // TODO Auto-generated constructor stub this.endPoint1=town; this.endPoint2=town2; this.distance= i; this.name=string; }
public String getName() { // TODO Auto-generated method stub return name; }
@Override public int compareTo(Road o) { // TODO Auto-generated method stub if(this.name.equals(o.name)) { return 1; } if(this.distance==(o.distance)) { return 1; } else return 0; }
public Town getEndPoint1() { return endPoint1; }
public void setEndPoint1(Town endPoint1) { this.endPoint1 = endPoint1; }
public Town getEndPoint2() { return endPoint2; }
public void setEndPoint2(Town endPoint2) { this.endPoint2 = endPoint2; }
public int getDistance() { return distance; }
public void setDistance(int distance) { this.distance = distance; }
public void setName(String name) { this.name = name; } public String toString() { return name; } }
town class
import java.util.ArrayList;
/** * @author Will Tchouente * class holds the name of the town and a list of adjacent towns * */ public class Town implements Comparable { private ArrayList towns; private String name;
//constructor public Town(String name, ArrayList towns) { // TODO Auto-generated constructor stub this.name=name; this.setTowns(towns); }
/** * @param string */ public Town(String string) { this.name=string; }
/** * @param i */ public void setWt(int i) { // TODO Auto-generated method stub
} /** * @param name */ public void setName(String name) { this.name = name; }
/** * @return */ public String getName() { // TODO Auto-generated method stub return name; }
/* (non-Javadoc) * @see java.lang.Comparable#compareTo(java.lang.Object) */ @Override public int compareTo(Town arg0) { // TODO Auto-generated method stub if(this.name.equals(arg0.name)) { return 1; } else return 0; } public String toString() { return name; }
public ArrayList getTowns() { return towns; }
public void setTowns(ArrayList towns) { this.towns = towns; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
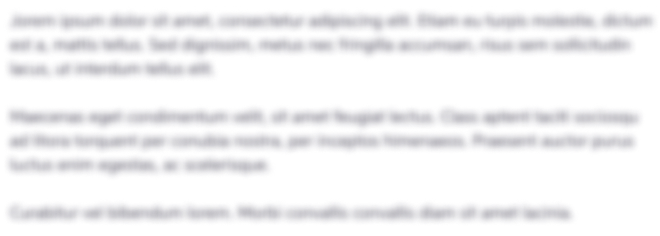
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started