Question
I need to modify the bag CLASS to use a Dynamic Array. Couple options and one of them is to modify the BAG CLASS to
I need to modify the bag CLASS to use a Dynamic Array. Couple options and one of them is to modify the BAG CLASS to contain an object of Dynamic Array Class.
Bag.h //Start
#pragma once
#include
class Bag
{
public:
typedef double value_type;
typedef std::size_t size_type;
static const size_type CAPACITY = 30;
Bag();
size_type size();
void insert(const value_type& entry);
size_type count(const value_type& target) const;
bool erase_one(const value_type& target);
size_type erase(const value_type& target);
void operator +=(const Bag& addEnd);
private:
value_type data[CAPACITY]; // array to store items
size_type used; // how much of array is used
};
Bag operator +(const Bag& b1, const Bag& b2);
Bag.ccp //Start
#include "Bag.h"
#include
#include
using namespace std;
Bag::Bag()
{
used = 0;
}
bool Bag::erase_one(const value_type& target)
{
size_type index = 0;
while (index < used && data[index] != target)
index++;
if (index >= used)
return false;
used--;
data[index] = data[used];
return true;
}
void Bag::insert(const value_type& entry)
{
assert(size() < CAPACITY);
data[used] = entry;
used++;
}
Bag::size_type Bag::count(const value_type& target) const
{
size_type answer = 0;
for (unsigned int i = 0; i < used; i++)
{
if (data[i] == target)
answer++;
}
return answer;
}
void Bag::operator +=(const Bag& addEnd)
{
assert(size() + addEnd.used <= CAPACITY);
for (unsigned int i = 0; i < addEnd.used; i++)
{
data[used] = addEnd.data[i];
used++;
}
}
Bag operator +(const Bag& b1, const Bag& b2)
{
Bag answer;
//assert(b1.size() + b2.size() <= Bag::CAPACITY);
answer += b1;
answer += b2;
return answer;
}
Bag::size_type Bag::erase(const value_type& target)
{
size_type index = 0;
size_type numErased = 0;
while (index < used)
{
if (data[index] == target)
{
used--;
data[index] = data[used];
numErased++;
}
else
index++;
}
return numErased;
}
Bag::size_type Bag::size()
{
return used;
}
Bag2.ccp //Start
#include "Bag.h"
#include
#include
using namespace std;
void getAges(Bag& ages)
{
int userInput;
cout << "Type the ages in your family." << endl;
cout << "Type a negative number when you are done." << endl;
cin >> userInput;
while (userInput >= 0)
{
if (ages.size() < ages.CAPACITY)
ages.insert(userInput);
else
cout << "I have run out of room and can't add that age." << endl;
cin >> userInput;
}
}
void checkAges(Bag& ages)
{
int userInput;
cout << "Type those ages again. Press return after each age:" << endl;
while (ages.size() > 0)
{
cin >> userInput;
if (ages.erase_one(userInput))
cout << "Yes, I've found that age and removed it." << endl;
else
cout << "No, that age does not occur." << endl;
}
}
void main()
{
// First test:
Bag ages;
getAges(ages);
checkAges(ages);
cout << "May your family live long and prosper." << endl;
// Second test:
Bag testBag;
for (int i = 0; i < 30; i++)
{
double r = 1.0 + (rand() % 12);
testBag.insert(r);
}
cout << " NEXT TEST: " << endl;
cout << "Bag size: " << testBag.size() << endl;
cout << "Occurences of the number 6: " << testBag.count(6) << endl;
Bag::size_type count = testBag.erase(6);
cout << "Bag size: " << testBag.size() << endl;
cout << "Occurences of the number 6: " << testBag.count(6) << endl;
system("pause");
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
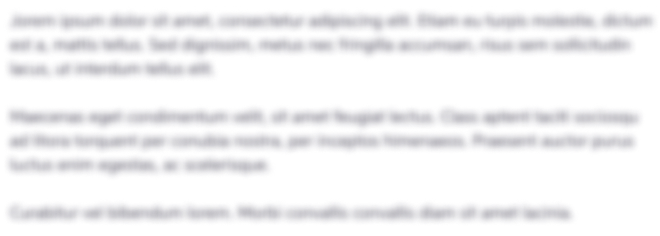
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started