Question
I need to write 6 new methods for an assignment. Java please. MyLinkedList must use generics . Here is starter code: http://www.filedropper.com/assignment5-starter Update MyLinkedList.java as
I need to write 6 new methods for an assignment. Java please. MyLinkedList must use generics.
Here is starter code:
http://www.filedropper.com/assignment5-starter
Update MyLinkedList.java as needed to achieve the specified functionality.
The 6 methods to implement are:
void removeAll(Object value)
MyLinkedList
boolean equals(Object o)
MyLinkedList
void doubler()
void sublist(int i, int j)
Method Specifications
You need to complete all of the following methods in the MyLinkedList class. You can write the methods in any order you wish, but I suggest you do them in the order they are listed. In particular, make sure all your methods work for empty lists, lists of different sizes, etc. as you can see from the sample test cases provided in ListTest.java. Also, make sure that your solutions do not modify the original lists (unless you are specifically instructed to do so).
void main(String[] args)
You can use this method in the ListTest class to create lists as needed and test each of the 12 methods listed above. Until you get add working, you will need to use addFirst to create lists to test your other methods. Ultimately, you should augment the test suite in ListTest.java to more exhaustively test your code. Note that ListTest.java wont compile until MyLinkedList uses generics.
MyLinkedList
Returns a new list that is a (shallow) copy of this list.
[Update Nov. 15] Your implementation of clone will make a deep copy of the list but a shallow copy of the data stored in each node. Create a new list and new nodes, but the new list will be a series of nodes created with something like:
Node nodeCopy = new Node(currNode.data, null);
// more code to insert nodeCopy into the new list
void removeAll(Object value)
This method removes every node that contains value in the list. Realize that the value can occur multiple times and anywhere in the list! This method does nothing if value is not in the list.
boolean equals(Object o)
This method overrides the equals method (found in the Object class). Since you are overriding the equals method, it must have the same signature as the one found in the Object class! As a result, you will need to cast the Object parameter to a variable of appropriate type (MyLinkedList
MyLinkedList
Once you've made the cast, the method should then compare list with this list for equality. The method returns true if and only if both lists have the same size and all corresponding pairs of elements in the two lists are equal. You must not create any other list in this method, and your solution must not modify the original lists.
MyLinkedList
This method splits the original list in half. The original list will continue to reference the front half of the original list and the method returns a reference to a new list that stores the back half of the original list. If the number of elements is odd, the extra element should remain with the front half of the list.
doubler()
Modifies the list so that each element is replaced by two adjacent copies of itself. For instance, if the list is [1,2,3,3,4], then list.doubler() should modify the list into [1,1,2,2,3,3,3,3,4,4].
sublist(int i, int j)
Returns a new list consisting of the elements of the original list starting at index i and ending at index j-1.
Other methods
It is OK to add other methods (helper methods) to complete the 12 methods described above.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
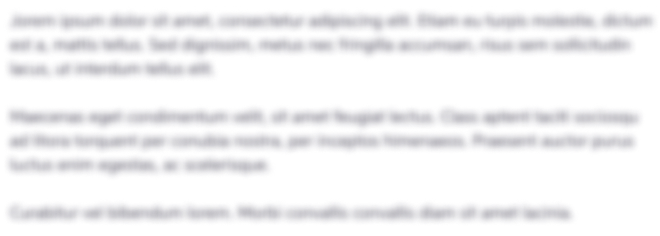
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started