Question
I need to write the implementations for Card.cpp, Hand.cpp and Blackjack.cpp in that order so I can implement a small blackjack game in c++ but
I need to write the implementations for Card.cpp, Hand.cpp and Blackjack.cpp in that order so I can implement a small blackjack game in c++ but I have been having trouble implementing Hand.cpp and Blackjack.cpp files. I think I manage to finish Card.cpp but I am not sure if the implementation is correct. Can you please show me a good approach for Hand.cpp and Blackjack.cpp. I provide here their h files as well. I do not need to care for the colors but I need strings for the type and I need to get the values for the cards and implement the class functions provided. Thank you!
//blackjack.h file
#ifndef BLACKJACK_H
#define BLACKJACK_H
#include "Hand.h"
#include
#include
enum Hit_or_Stand { HIT, STAND };
class Blackjack
{
private:
double playerMoney, playerBet;
// Objects of class Hand representing the player's hand and the dealer's hand.
Hand playerHand, dealerHand;
// An object of enumerated type Hit_or_Stand that holds the player's choice.
Hit_or_Stand playerChoice;
// Member function to obtain the player's choice.
Hit_or_Stand queryPlayer(); // Write an implementation of this function. It must
// keep querying the player until a valid choice is
// entered, then return that choice as a Hit_or_Stand.
public:
// Constructor. I have written this implementation for you.
Blackjack(double money)
:playerMoney(money), playerHand("Player"), dealerHand("Dealer")
{ }
// Member function 'play' should play a round of Blackjack according to the rules
// of the game, and return the updated playerMoney.
double play(double bet); // Write an implementation of this function.
};
#endif
//Card.h file
#ifndef CARD_H // include guard
#define CARD_H
#include
class Card
{
private:
// Data members of class Card
std::string typeString; // this will store a string corresponding to the
// card type, e.g. "Ace", or "Two", or "Jack"
int initial_value; // this will hold an integer corresponding to the initial value
// of the card type. For an Ace initial_value = 11,
// a Two initial_value = 2, a Three initial_value = 3, etc.
// Jack, Queen, and King should each have initial_value = 10
// It is called 'initial value' because the value of an Ace
// will be effectively reduced to 1 if the Hand's value gets too big.
// But this will be handled in class Hand.
public:
Card(); // write an implementation for this constructor. It should call a random
// number generator to produce a random number between 1 - 13, and then
// assign the typeString accordingly, i.e. 1 becomes an "Ace", 2 becomes a "Two",
// 3 becomes a "Three", etc. 11 becomes a "Jack", 12 becomes a "Queen",
// 13 becomes a "King". Also set the initial_value of the card.
std::string getType() const; // write an implementation for this function. It should
// return the string typeString
int getValue() const; // write an implementation for this function. It should
// return the int initial_value
};
#endif
//Hand.h file
#ifndef HAND_H
#define HAND_H
// Definition of class Hand, but only function prototypes included
#include
#include
#include "Card.h"
class Hand
{
private:
int value; // This should hold the total value of the hand. Update every time you
// add a card
int number_of_aces; // this should hold the number of Aces in the hand, which is
// useful for calculating hand value. Update every time you
// add a card
int number_of_cards; // this should hold the total number of cards in the hand.
// Update every time you add a card.
Card * cards[11]; // an array of 11 Card pointers to point to the hand's cards.
std::string hand_holder; // Holds either "Player" or "Dealer"
void updateValue(); // Private member function that updates the hand's value.
// You must write an implementation for this function.
// It should be called every time you add a card.
public:
explicit Hand(std::string); // Constructor, sets hand_holder. Write an implementation.
~Hand(); // Destructor. Deallocates all cards. Write an implementation.
Hand & operator++(); // Adds a randomly chosen card to the hand. It must do so using
// a random number generator, and must use the command 'new'
// to dynamically allocate a new Card. Write an implementation.
// Below are a set of overloaded operators for comparing the value of a Hand to the
// value of another Hand, or to an int. You must write implementations for all of these.
bool operator>(const Hand & rhs) const;
bool operator>(int rhs) const;
bool operator<(const Hand & rhs) const;
bool operator<(int rhs) const;
bool operator==(const Hand & rhs) const;
bool operator==(int rhs) const;
void printHand(); // This function should print the cards in the hand, and the total
// value of the hand. Write an implementation.
void printFirstCard(); // This function should print only the first card in the hand
// (used for dealer). Write an implementation.
// Here I'm implementing a copy constructor and an assignment operator for you.
// It is necessary to write our own copy constructor because of the dynamically
// allocated memory in this class, see class notes.
// You shouldn't use these functions in your final code, but they may be useful for debugging
// purposes, or for the extra credit.
Hand (const Hand & hand_to_copy)
: hand_holder(hand_to_copy.hand_holder), number_of_aces(hand_to_copy.number_of_aces),
number_of_cards(hand_to_copy.number_of_cards), value(hand_to_copy.value)
{
for (int i = 0; i < number_of_cards; i++)
cards[i] = new Card(*(hand_to_copy.cards[i]));
std::cout << "Your code called the copy constructor for Hand." <<
" It shouldn't do that unless maybe you're doing the extra credit. FYI" << std::endl;
}
void operator= (const Hand & hand_to_copy)
{
for (int i = 0; i < number_of_cards; i++)
delete cards[i];
hand_holder = hand_to_copy.hand_holder;
number_of_aces = hand_to_copy.number_of_aces;
number_of_cards = hand_to_copy.number_of_cards;
value = hand_to_copy.value;
for (int i = 0; i < number_of_cards; i++)
cards[i] = new Card(*(hand_to_copy.cards[i]));
std::cout << "Your code called the assignment operator for Hand." <<
" It shouldn't do that unless maybe you're doing the extra credit. FYI" << std::endl;
}
};
#endif
//Card.cpp file
#include "Card.h"
#include
#include
#include
#include
using namespace std;
char const* mtypeString;
int getinit;
Card::Card(){
srand( static_cast
getinit=rand()%13+1;
if (getinit==1){mtypeString="Ace";}
else if(getinit==2){mtypeString="Two";;}
else if(getinit==3){mtypeString="Three";}
else if(getinit==4){mtypeString="Four";}
else if(getinit==5){mtypeString="Five";}
else if(getinit==6){mtypeString="Six";}
else if(getinit==7){mtypeString="Seven";}
else if(getinit==8){mtypeString="Eight";}
else if(getinit==9){mtypeString="Nine";}
else if(getinit==10){mtypeString="Ten";}
else if(getinit==11){mtypeString="Jack";}
else if(getinit==12){mtypeString="Queen";}
else if(getinit==13){mtypeString="King";}
initial_value=getinit;
typeString=mtypeString;
//cout<<"This is the value: "< } /*gettype::gettype(){ typeString=mtypeString; return typeString; */ int Card::getValue() const { //initial_value=getinit; return initial_value; } std::string Card::getType() const{ return typeString; } //Hand.cpp file with implementations already but incomplete. I am unable to finish it because I do not // get how to apply some of the provided functions #include "Card.h" //#include "Card.cpp" #include "Hand.h" #include #include #include #include using namespace std; int myval; //char const* mystring; Hand::Hand(std::string){ value=0; number_of_aces=0; number_of_cards=0; hand_holder=""; } void Hand::updateValue(){ Card mycard; myval=mycard.getValue(); value=value+myval; } ~Hand() Hand & operator++() bool operator>(const Hand & rhs) const; bool operator>(int rhs) const; bool operator<(const Hand & rhs) const; bool operator<(int rhs) const; bool operator==(const Hand & rhs) const; bool operator==(int rhs) const; void printHand(); // This function should print the cards in the hand, and the total // value of the hand. Write an implementation. void printFirstCard(); //I also need to complete the blackjack.cpp file as well //here is the main.cpp file // Blackjack game code. #include "Blackjack.h" #include using namespace std; // Function to take player's bet. double takeBet(const double & playerMoney) { double bet; do { cout << "Enter bet in dollars, 0 to quit: "; cin >> bet; if (bet > playerMoney) cout << "You don't have that much money! Enter a lower bet." << endl; } while (bet > playerMoney); return bet; } // main function int main() { // Seed the random number generator with current time std::srand( static_cast // Player starts with 300 dollars double playerMoney = 300.0, playerBet; // integer to hold the round number int roundNum = 1; cout << "Player starts with " << playerMoney << " dollars" << endl; cout << "Round " << roundNum << endl; // Take bet playerBet = takeBet(playerMoney); // Keep playing Blackjack until the player bets 0 dollars, or they are broke. while ((playerBet > 0.0) && (playerMoney > 0.0)) { // Instantiate Blackjack object, which initializes round. Blackjack myBlackjack(playerMoney); // Play the round of Blackjack. Member function 'play' should return the updated // playerMoney. playerMoney = myBlackjack.play(playerBet); // Take a new bet unless the player is broke. if (playerMoney > 0.0) { cout << endl << endl << "Round " << ++roundNum << endl; playerBet = takeBet(playerMoney); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
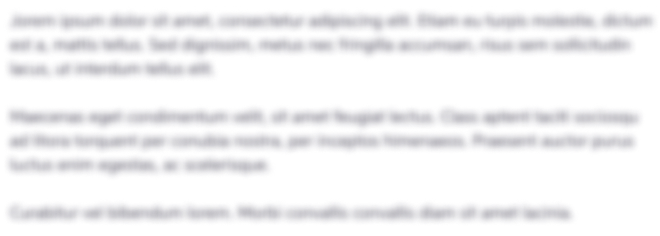
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started