Question
I only need the answer to part 4b!!!! A file has a name. A file is either a plain file (like a text file, an
I only need the answer to part 4b!!!!
A file has a name. A file is either a plain file (like a text file, an image file, a C++ source program, etc.) or a directory. Directories contain zero or more files. The following program reflects this structure:
#include#include #include using namespace std; class File { public: File(string nm) : m_name(nm) {} virtual ~File() {} string name() const { return m_name; } virtual bool add(File* f) = 0; virtual const vector * files() const = 0; private: string m_name; }; class PlainFile : public File { public: PlainFile(string nm) : File(nm) {} virtual bool add(File* f) { return false; } virtual const vector * files() const { return nullptr; } }; class Directory : public File { public: Directory(string nm) : File(nm) {} virtual ~Directory(); virtual bool add(File* f) { m_files.push_back(f); return true; } virtual const vector * files() const { return &m_files; } private: vector m_files; }; Directory::~Directory() { for (int k = 0; k < m_files.size(); k++) delete m_files[k]; } void listAll(string path, const File* f) // two-parameter overload { You will write this code. } void listAll(const File* f) // one-parameter overload { if (f != nullptr) listAll("", f); } int main() { Directory* d1 = new Directory("Fun"); d1->add(new PlainFile("party.jpg")); d1->add(new PlainFile("beach.jpg")); d1->add(new PlainFile("skitrip.jpg")); Directory* d2 = new Directory("Work"); d2->add(new PlainFile("seaslab.jpg")); Directory* d3 = new Directory("My Pictures"); d3->add(d1); d3->add(new PlainFile("me.jpg")); d3->add(new Directory("Miscellaneous")); d3->add(d2); listAll(d3); delete d3; }
When the listAll function is called from the main routine above, the following output should be produced (the first line written is /My Pictures, not an empty line):
/My Pictures /My Pictures/Fun /My Pictures/Fun/party.jpg /My Pictures/Fun/beach.jpg /My Pictures/Fun/skitrip.jpg /My Pictures/me.jpg /My Pictures/Miscellaneous /My Pictures/Work /My Pictures/Work/seaslab.jpg
This is a list, one per line, of the complete pathname for listAll's argument and, if the argument is a directory, everything in that directory. A pathname starts with / and has / separating pathname components.
You are to write the code for the two-parameter overload of listAll to make this happen. You must not use any additional container (such as a stack), and the two-parameter overload of listAll must be recursive. You must not use any global variables or variables declared with the keyword static, and you must not modify any of the code we have already written or add new functions. You may use a loop to traverse the vector; you must not use loops to avoid recursion.
Here's a useful function to know: The standard library string class has a + operator that concatenates strings and/or characters. For example,
string s("Hello"); string t("there"); string u = s + ", " + t + '!'; // Now u has the value "Hello, there!"
It's also useful to know that if you choose to traverse an STL container using some kind of iterator, then if the container is const, you must use a const_iterator:
void f(const list& c) // c is const { for (list ::const_iterator it = c.begin(); it != c.end(); it++) cout << *it << endl; }
(Of course, a vector can be traversed either by using some kind of iterator, or by using operator[] with an integer argument).
For this problem, you will turn a file named list.cpp with the body of the two-parameter overload of the listAll function, from its "void" to its "}", no more and no less. Your function must compile and work correctly when substituted into the program above.
We introduced the two-parameter overload of listAll. Why could you not solve this problem given the constraints in part a if we had only a one-parameter listAll, and you had to implement it as the recursive function?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
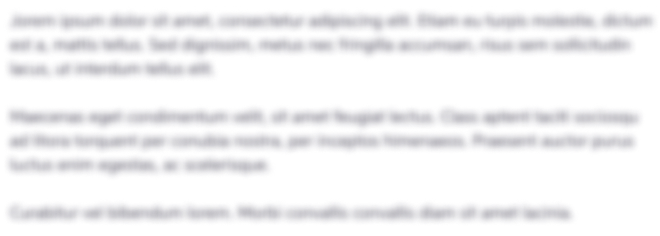
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started