Question
I posted exercises 1 and 2 in a post before. I need the answers for excersies 3,4&5 for this post please. Exercise 1 Description Create
I posted exercises 1 and 2 in a post before. I need the answers for excersies 3,4&5 for this post please.
Exercise 1 Description
Create a ClosedLab11 project folder. FOR ALL EXERCISES: Make sure you place a comment block at the top of the code with your name and your partner's name. In your ClosedLab11 project folder, import the following file:
ClosedLab11.java
There is no actual code in this file - only a set of stubs. For Exercise 1 you should just look at the isPositive method. In a separate text file named IsPositiveTests.txt, come up with a set of test cases for this method. Your test cases should test all possible cases for inputs to the isPositive method for arrays up to size 5. Make sure your test cases also indicate what the expected output should be for each test case. You will be turning in this text file as part of this lab, so take this part seriously even though there is no "coding" in this part. Once you feel that you have sufficient coverage of test cases (and you should have a good number of them - make sure you're testing arrays of all lengths up to 5 and all of the possible ways that each array could result in a true or false result), you should create a JUnit test class for the isPositive method. Highlight the ClosedLab11 class in your Package Explorer, right click and select New -> JUnit Test Case. Change the name of this Test Case to "IsPositiveTest" and click Next, then select just the isPositive method and click Finish. You should now have a JUnit class open in front of you. Fill in the JUnit class with the code for your first test case as described in class. Then run your JUnit test case. Does it pass? If it does, add your next test case to the mix and try again. Keep going until you find a test case that fails. Once you have a failed test case, look at the test case. Then open up the code for the isPositive method and make whatever changes you need to make to get that failed test case to work. Then go back and run your JUnit testing again - did it pass this time? If not, work out the bugs until you can get it to pass. If it did pass, are any of the previous test cases now broken? If so, go back and fix your isPositive method until all of the test cases you have so far work. Once you have all of your current test cases working, add another test case and repeat the cycle. Keep going until all of your test cases work in JUnit. Are you comfortable saying that your code works for all cases? If not, think about why you're not comfortable and think about some test cases you can add to either break your code or convince yourself that your fears are unfounded.
Exercise 2
Repeat the above process for the computeShipping method, including creating a text file containing your test cases and a separate JUnit method testing the computeShipping method (name this ComputeShippingTest).
Exercise 3
Now we want to examine the testing of instance methods. Import the following into your ClosedLab11 project folder:
Student.java
/** * Student.java * * * @author Jeremy Morris * @version 20120820 * * Class definition for a simple student object. * This version implements equals() and toString() * methods inherited from the Object superclass. */ import java.util.*; public class Student implements Comparable{ private String firstName; private String lastName; private String id; private ArrayList coursesTaken; private ArrayList courseGrades; /* * Constructs an empty student with a default of all * blank values for name and id attributes. */ public Student() { firstName=""; lastName=""; id=""; coursesTaken = new ArrayList(); courseGrades = new ArrayList(); } /* * Constructs a new Student object that is a copy of * another student object. * * @param sg1 - the Student to be copied */ public Student(Student sg1) { this.firstName = sg1.getFirstName(); this.lastName = sg1.getLastName(); this.id = sg1.getId(); this.coursesTaken = new ArrayList(); this.courseGrades = new ArrayList(); } /* * Constructs a new Student object that contains the * firstname, last name, and id entered as parameters. * * @param firstName - Student first name * @param lastName - Student last name * @param id - Student id */ public Student(String firstName, String lastName, String id) { this.firstName = firstName; this.lastName = lastName; if (!this.setId(id)) id = ""; this.coursesTaken = new ArrayList(); this.courseGrades = new ArrayList(); } /* * getFirstName() * Returns the first name of the student * * @return the first name of the student */ public String getFirstName() { return firstName; } /* * setFirstName * Sets the first name of the student * * @param firstName - first name of the student */ public void setFirstName(String firstName) { this.firstName = firstName; } /* * getLastName * Returns the last name of the student * * @return the last name of the student */ public String getLastName() { return lastName; } /* * setLastName * Sets the last name of the student * * @param lastName - the last name of the student */ public void setLastName(String lastName) { this.lastName = lastName; } /* * getId * Returns the id of the student * * @return the id of the student */ public String getId() { return id; } /* * setId * Sets the id of the student if the id has a length of 10, * otherwise returns false. * * @return true if the id is set, false otherwise */ public boolean setId(String id) { boolean set = false; if (id.length() == 10) { this.id = id; set = true; } return set; } /* * addCourse * Adds the course name and grade to the student's record * * @param courseName - the name of the course * @param courseGrade - grade of the course between 0.0 and 4.0 */ public void addCourse(String courseName, double courseGrade) { if (courseGrade>=0.0 && courseGrade<=4.0) { coursesTaken.add(courseName); courseGrades.add(courseGrade); } } /* * getCourseName * * @param index - the position of the course in the course list * @return the name of the course at this position */ public String getCourseName(int index) { return coursesTaken.get(index); } /* * getCourseGrade * * @param index - the position of the course in the course list * @return the grade of the course at this position */ public double getCourseGrade(int index) { return courseGrades.get(index); } /* * (non-Javadoc) * @see java.lang.Object#toString() */ @Override public String toString() { return "("+firstName+" "+lastName+" "+id+")"; } /* * (non-Javadoc) * @see java.lang.Object#equals(java.lang.Object) */ @Override public boolean equals(Object obj) { boolean equal=false; if (obj instanceof Student) { Student tmp = (Student) obj; if (this.firstName.equals(tmp.getFirstName()) && this.lastName.equals(tmp.getLastName()) && this.id.equals(tmp.getId())) { equal=true; } else { equal=false; } } return equal; } /* * compareTo * * Students should be compared in alphabetical order of their last names. If two students have the same * last name, then their first name should be used as the tie-breaker. If two students have the same * first name and the same last name, then they should be listed in order of their student IDs. */ @Override public int compareTo(Student arg0) { // TODO Auto-generated method stub return 0; } }
We want to add a compareTo method to this class. The rules for comparing two students are:
Students should be ordered according to their last names (so Abbot comes before Zelazny)
If two students have the same last names, they should be ordered according to their first names (so Justin Abbot comes before Zachary Abbot)
If two students have the same first and last names, they should be ordered by their student IDs (so Bob Zelazny ID:0000000001 comes before Bob Zelazny ID:999999999)
Two students with the same names and the same ids are equal (just like our test for the equals method - REMEMBER: compareTo should always be consistent with equals)
BEFORE WRITING ANY CODE! Come up with your test plan. How are you going to test your compareTo method? Write up your test plan in a plain text file named StudentTestPlan.txt - include inputs and expected outputs for each test case (remember - the expected outputs here are -1, 0 and 1). Now create a JUnit test class and when you reach the window where you select the methods to test, select only the compareTo method. Start writing your JUnit tests as above, but this time remember that you need to declare objects of type Student to test against each other. (Remember that Student has a constructor that takes the first name, last name and id as parameters - this can make your life easier than if you use a lot of setter method calls). Follow the test driven development idea to modify your compareTo method to return the right results as it fails test cases. Refine your compareTo method as you go, rather than trying to write it all at once! When you reach the point where you pass all of your test cases, take a step back. Are you sure that your test cases have covered all of the possible problems? Did you think of anything else that might be a problem as you were writing your code? If so, add those test cases to the text file and to your JUnit code and clearly mark in the text file which cases were added "after the fact".
Exercise 4
Go back to your ClosedLab11.java methods. Repeat the process outlined in Exercise 1 for the isReverse method. Name the JUnit class in this case IsReverseTest.
Exercise 5
Repeat the above process for the computeGraduatedTax method. This one is the most complex method of the ones that you've tested, so make sure that your test cases have good coverage. Name the JUnit method for this one ComputeGraduatedTaxTest.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
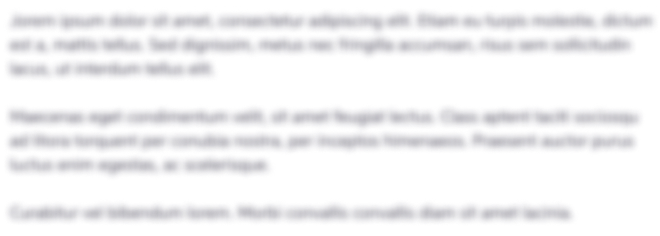
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started