Question
I tried submitting my code in UVa Online Judge, which is a website filled with computer programming challenge questions. I tried submitting my code for
I tried submitting my code in UVa Online Judge, which is a website filled with computer programming challenge questions. I tried submitting my code for one of their problems called freckles. It kept giving me this error, "Presentation error C++". Below is the definition Submission specification for the code and the meaning of "Presentation error C++". I think It has to do with taking inputs and printing outputs? There is only one printf statement, which is at the very end of my main function. My program works and achieves the correct output. I've also provided my code below. How do I fix my print statements so it gets accepted?
Presentation Error (PE):
Your program outputs are correct but are not presented in the correct way. Check for spaces, justify, line feeds...
Submission specification:
Currently, only the submissions via the "Quick Submit" link or the "Submit" icon for each problem is possible. In order to submit your code, just fill in the form and press "submit". Using this form, you don't have to include any special header to the file as everything is handled by the system.General Specifications: The program reads the test input from the standard input (stdin) and places the results in the standard output (stdout). This is always the case, regardless on what the problem description says. Every line in the input is guaranteed to be finished by the end-of-line character (' '). In a similar way, your program must print an end-of-line character at the end of every line. A correct output with a missing end-of-line character will not be judged as Accepted.
As a reference, the website provide a sample C code:
/* @JUDGE_ID: 1000AA 100 C "Easy algorithm" */ /************** solution to problem 1, Duke ACM Internet programming contest by: Owen Astrachan date: 10/19/90 **************/ #includeint cycle(m) int m; /* return number of elements in the 3n+1 cycle for m */ { int i = 1; while (m != 1){ if (m % 2 == 0){ m = m/2; } else{ m = 3*m+1; } i++; } return i; } int main() { int m,n,max,temp; int mOriginal,nOriginal; int i; while (scanf("%d %d ",&m,&n)==2){ /* remember original order of entries for output */ mOriginal = m; nOriginal = n; /* swap if out of order */ if (m > n){ temp = m; m = n; n = temp; } max = cycle(m); for(i=m+1;i<=n;i++) { temp = cycle(i); if (temp > max) max = temp; } printf("%d %d %d ",mOriginal,nOriginal,max); } return(0); }
My Code in C++:
#include #include #include #include using namespace std;
const int arraySize = 150; // arraySize is used as a size limit for the the arrays double points[arraySize][2]; // Stores the points, x and y location (vertex) double edgeWeight[arraySize][arraySize]; // Stores the distance (edge weight) double shortestPath[arraySize]; // Stores the shortest edge weight double output[arraySize]; // Stores the output from Prim function int row[arraySize]; // Helps us access the row section within the 2-D array points when it comes time to add // an edge weight to the variable total int col[arraySize]; // Prevents the program from accessing edge weight that have already been added to the // variable total. This also helps us access the column section of 2-D array points when it // comes time to add an edge weight to the variable total int frecklesNum; // The number of freckles for each test cases /* * The getCoordinates method takes the coordinates x and y from the user and stores them inside the 2-D array points */ void getCoordinates() { // This for loop will iterate by how many freckles there are. The scanf in this for loop is used to get // the actual coordinates from the user, since the coordinate consists of just two numbers, x and y, the // second bracket of the 2-dimensional array point will be 0 and 1, incidcating that there will only be two // columns to fill. for(int i = 1; i <= frecklesNum; i++) { scanf("%lf %lf", &points[i][0], &points[i][1]); } // end of for loop } // end of getCoordinates function
/* * The getEdgeWeight method calculates the distance between the two points (x1, y1) and (x2, y2) * and uses that distance as an edge weight. We store edge weights inside the 2-D array edgeWeight. * @param x1 the first x coordinate * @param y1 the first y coordinate * @param x2 the second x coordinate * @param y2 the second y coordinate */ void getEdgeWeight() { for(int i = 1; i <= frecklesNum; i++) { for(int j = 1; j <= frecklesNum; j++) { // This if statement does not execute if both index i and j are equal. // This is because when both index i and j are equal, both set of points will be // the same, and taking the distance of a point from itself is not very useful. if(i != j) { // Calculate the distance (edge weight) of two points using the formula sqrt((x1-x2)*(x1-x2) + (y1-y2)*(y1-y2)) edgeWeight[i][j] = sqrt( ((points[i][0]-points[j][0]) * (points[i][0]-points[j][0])) + ((points[i][1]-points[j][1]) * (points[i][1]-points[j][1])) ); } // end of for loop }// end of for loop } // end of for loop } // end of getEdgeWeight function /* * The Prim method implements the prim's algorithm to find the edges with the least possible cost * @return The minimum total length of ink lines that can connect all the freckles */ double Prim() { // We have no idea what the value of all the bytes are in col, shortestPath, and row array, so we set all of // them to zero using the memset function before using them. memset(col, 0, sizeof(col)); memset(shortestPath, 0, sizeof(shortestPath)); memset(row, 0, sizeof(row));
// Set the second subscript in the array equal to 1 // This will prevent the program from accessing edge weight in the 2-D array points that have already been added to // the variable total later on in the program. We will use the index location of the edge weight in shortestPath that // was already added to the variable total, and use that index to set the same index location in the col // array to 1. We will use the value 1 in the col array as an indication to skip over that edge weight (because it // has already been added) on the next couple of iterations. col[1] = 1;
// Loop through the 2d array points where all the distance (edge weight) are currently stored and store them inside // the shortestPath array. Here we also mark the row array with 1. This corresponds to the row section of the 2-D // edgeWeight array. for(int i = 1; i <= frecklesNum; ++i) { shortestPath[i] = edgeWeight[1][i]; row[i] = 1; } // end of for loop
// Total will contain the total length of ink lines that can connect all the freckles double total = 0;
// This for loop will iterate by how many edges there are, which is equal to the number of points minus 1. // If there are three points, then the total number of edges are 3 - 1 = 2. for(int i = 1; i < frecklesNum; ++i) { // ***BIG FOR LOOP*** int z = 0;
for(int j = 1; j <= frecklesNum; ++j) { // This if statement will not execute if the value inside col[j] is equal to 1. // In the beginning of the Prim method we have set col[1] = 1, therefore this if // statement will not execute on the first iteration of the for loop that it's in. // on the next couple of iterations, this if statement is used to skip over edge // weights that have already been added to the variable total. if(col[j] != 1) { // Here we are finding the index with the smallest value stored inside the // array shortestPath, and we store that index inside variable z. if( (z == 0) || (shortestPath[j] < shortestPath[z]) ) { z = j; } // end of if statement } // end of if statement } // end of for loop
// Take that edge weight that we stored in index z and add it in the total variable // We get help from the row[z] array to access the right row index in the 2-D array edgeWeight total += edgeWeight[row[z]][z];
// The idea here is to mark the location of the index z inside the col arrary to 1. // On the next iteration of the for loop, the code if(col[j] != 1) on the value // of index z (this holds the value that we previously added to variable total) will // result in a false boolean value. This basically tells us that we have already added // this particular edge weight and so we skip over it. col[z] = 1;
for(int j = 1; j <= frecklesNum; ++j) { // This if statement will not execute if the value inside col[j] is equal to 1. // The j value of 1 is the index value inside shortestPath that holds the value that has // already been added to variable total. We skip over them. if(col[j] != 1) { // This if statement finds the smallest distance (edge weight) within the edgeWeight 2-D array // and stores it in shortestPath array. If the distance stored in edgeWeight[z][j] is smaller than // what we already have in location shortestPath[j], then store edgeWeight[z][j] in shortestPath[j]. if(shortestPath[j] > edgeWeight[z][j]) { shortestPath[j] = edgeWeight[z][j]; // Here, we set the value inside row[j] to variable z, and z is the index value of the row section // in 2-D array edgeWeight where we found the new smallest edge weight. This information will be // used to access the same edge weight so we can add it to the variable total later on. row[j] = z; } // end of if statement } // end of if statement } // end of for loop
} // end of ***BIG FOR LOOP***
return total; } // end of Prim function
int main(int argc, char ** argv) { // Get input from user // testCaseNum represents the number of test cases int testCaseNum = 0; scanf("%d", &testCaseNum);
// outputLimit will be used to access the array where all the output of the Prim function is stored int outputLimit = testCaseNum;
// We have no idea what the value of all the bytes are in this output array, // so we set all of them to zero using the memset function. memset(output, 0, sizeof(output));
// We loop testCaseNum number of times to get the freckle coordinates from the user for(int i_o = 1; i_o <= testCaseNum; i_o++) { // BEGINNING OF BIG FOR LOOP // This scanf takes the number of freckles for each test cases, which should be 0 < freckles <= 100 scanf("%d", &frecklesNum);
// We have no idea what the value of all the bytes are in this 2-D array point, // so we set all of them to zero using the memset function. memset(points, 0, sizeof(points));
// GET COORDINATES getCoordinates();
// We have no idea what the value of all the bytes are in this 2-D array edgeWeight, // so we set all of them to zero using the memset function. memset(edgeWeight, 0, sizeof(edgeWeight));
// GET EDGE WEIGHT getEdgeWeight();
// Store the output of Prim method in an array. output[i_o] = Prim(); // print the output(s) if (i_o == testCaseNum) { for (int i = 1; i < outputLimit+1; i++) { printf(" "); printf("%.2lf ", output[i]); } } // end of if statement } // END OF BIG FOR LOOP
// line printf(" "); return 0; } // end of main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
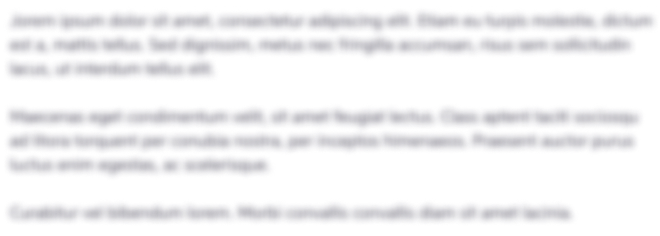
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started