Question
I tried to upload the image, but it wont let me to download. Direction: (Game: nine heads and trails) Nine coins are placed in a
I tried to upload the image, but it wont let me to download.
Direction:
(Game: nine heads and trails) Nine coins are placed in a 3 x 3 matrix with some face up and some face down. You can represent the state of the coins using a 3 x 3 matrix with values 0 (head) and 1 (trail). Here's are some examples:
000 101 110 101 100
010 001 100 110 111
000 100 001 100 110
Each state can also be represented using a binary number. For example, the preceding matrices correspond to the numbers
000010000 101001100 110100001 101110100 100111110
The total number of possibilties is 512. So you can use decimal numbers 0,1,2,3,..., and 511 to represent all states of the matrix. Write a program that prompts the user to enter a number betweem 0 and 511 and displays the corresponding matrix with characters H and T. Here is a sample run :
Enter a number between 0 and 511: 7 [Enter]
H H H
H H H
T T T
only exercise 8.11 Can you explain that to me?
#include #include #include using namespace std; //functions are to be declared before defined. void Prog8_1(); void Prog8_2(); void Prog8_3(); void Prog8_4(); void Prog8_5(); void Prog8_9(); void Prog8_11(); void Prog8_17(); void Prog8_18(); void Prog8_30();
//functions are to be declared in the global location const int SIZE = 4; double sumColumn(const double [][SIZE], int rowSIZE, int columnIndex); double sumMajorDiagonal(const double m[][SIZE]); const int NUMBER_OF_STUDENTS = 8; const int NUMBER_OF_QUESTION = 10; const int N = 3; void addMatrix(const double a[][N], const double b[][N], double c[][N]); void multiplyMatrix(const double a[][N], const double b[][N], const double c[][N]); void locateLargest(const double a[][4], int location[]); void inverse(const double A[][3], double inverseOfA[][3]); double determinantOfAMatrix(double A[][N]); bool linearEquation(const double a[][SIZE], const double b[], double result[]);
int main() { while (true) { system("cls"); cout << " Main Menu - Chapter 8 "; cout << "================================= "; cout << " 1: Programming Exercise 8.1 "; cout << " 2: Programming Exercise 8.2 "; cout << " 3: Programming Exercise 8.3 "; cout << " 4: Programming Exercise 8.4 "; cout << " 5: Programming Exercise 8.5 "; cout << " 9: Programming Exercise 8.9 "; cout << " 11: Programming Exercise 8.11 "; cout << " 17: Programming Exercise 8.17 "; cout << " 18: Programming Exercise 8.18 "; cout << " 30: Programming Exercise 8.30 "; cout << "other: Exit "; cout << "================================= "; cout << "Enter an exercise: "; char exercise[2]; cin >> exercise; cout << endl; switch (atoi(exercise)) { case 1: Prog8_1(); break; case 2: Prog8_2(); break; case 3: Prog8_3(); break; case 4: Prog8_4(); break; case 5: Prog8_5(); break; case 9: Prog8_9(); break; case 11: Prog8_11(); break; case 17: Prog8_17(); break; case 18: Prog8_18(); break; case 30: Prog8_30(); break; default: exit(0); } cout << endl; system("pause"); cin.clear(); } return 0; }
void Prog8_1() { //delcare the variable const int SIZE = 4;
//define the function sumColmn which takes the matrix, row // size and column index as its argument double sumColumn(const double [][SIZE], int l, int SIZE) { //declare and initialize sumcol double sumCol = 0; //for loop is used to alterate through the each row of // the matrix for (int i = 0; i < SIZE; i++) { for (int j = 0; j < l; j++) { //add the column value sumCol += m [j][i]; } //display the sum of the each column cout << "Sum of the column " << i << "is" << sumCol< sumCol = 0; } }
//delcare a matrix 3 by 4 double m[3][SIZE];
//delcare a matrix of size 3 by 4 and read the input from the end user through //for loop. Call the function sumColumn() which wil add the sum of elements //of each column cout << "Enter a 3 by 4 matrix row by now: " << endl;
//read the value of the matrix row wise for (int i = 0; i < 3; i++) { for (int j = 0; j < SIZE; j++) { //read the value from the end user cin >> m[i][j]; } } //call the function sumColumn cout << sumColumn(m,3,4) << endl; }
void Prog8_2() { //declare a matrix of size 4 by 4 double matrix[SIZE][SIZE]; cout << "Enter a 4 by 4 matrix row by row: " << endl;
//nested for loop is used to read the elements of the //two dimensional 4 by 4 matrix for (int i = 0; i < SIZE; i++) { for (int j = 0; j < SIZE; j++) { //read the elements from the user cin >> matrix[i][j]; } } //display the sum of the elements of the major dimensional cout << "Sum of th elements in the major diagonal is " << sumMajorDiagonal(matrix) << endl; } double sumMajorDiagonal(const double m[][SIZE]) { //declare and initalize sum equals to zero double sum = 0;
//for loop is used to ilerate through the matrix and //store the sum of the major diagonal for (int i = 0; i < SIZE; i++) { sum += m[i][i]; } //return sum of the elements of the major diagonal return sum; }
void Prog8_3() { //Students' answers to the questions char answers[NUMBER_OF_STUDENTS][NUMBER_OF_QUESTION] = { {'A', 'B', 'A', 'C', 'C', 'D', 'E', 'E', 'A', 'D'}, {'D', 'B', 'A', 'B', 'C', 'A', 'E', 'E', 'A', 'D'}, {'E', 'D', 'D', 'A', 'C', 'B', 'E', 'E', 'A', 'D'}, {'C', 'B', 'A', 'E', 'D', 'C', 'E', 'E', 'A', 'D'}, {'A', 'B', 'D', 'C', 'C', 'D', 'E', 'E', 'A', 'D'}, {'B', 'B', 'E', 'C', 'C', 'D', 'E', 'E', 'A', 'D'}, {'B', 'B', 'A', 'C', 'C', 'D', 'E', 'E', 'A', 'D'}, {'E', 'B', 'E', 'C', 'C', 'D', 'E', 'E', 'A', 'D'} };
//key to the questions char keys [] = {'D', 'B', 'D', 'C', 'C', 'D', 'A', 'E', 'A', 'D'};
//grade all answers for (int i = 0; i < NUMBER_OF_STUDENTS; i++) { //grade one student int correctCount = 0; for (int j = 0; j < NUMBER_OF_QUESTION; j++) { if (answers[i][j] == keys[j]) correctCount++; } cout << "Student's " << i << " correct count is " << correctCount<< endl; } }
void Prog8_4() { //Number of employee's const int NoOfEmp = 8;
//Employee's seven-day work hours int Weekly_Hours[NoOfEmp][7] = { {2,4,3,4,5,8,8}, {7,3,4,3,3,4,4}, {3,3,4,3,3,2,2}, {9,3,4,7,3,4,1}, {3,5,4,3,6,3,8}, {3,4,4,6,3,4,4}, {3,7,4,8,3,8,4}, {6,3,5,9,2,7,9}, }; //total hours of total employees int total_hours[NoOfEmp];
// Index number of each employee int emp[NoOfEmp];
//array variable initalization for (int i = 0; i < NoOfEmp; i++) { total_hours[i] = 0; emp[i] = i; } //execute the for loop until the i is less than number of employee for (int i = 0; i < NoOfEmp; i++) { //execute the for loop until j is less than 7 for (int j = 0; j < 7; j++) { //add the weekly hours with total hours total_hours[i] += Weekly_Hours[i][j]; } } //execute the for loop until j is less than or equal to 7 for (int j = 0; j <= 7; j++) { //sort each employee's total hours in decreasing order for (int i = 0; i < NoOfEmp; i++) { //compare the total hours of employee in sequence if (total_hours[i] < total_hours[i+1]) { //sorting total hours int temp = total_hours[i]; total_hours[i] = total_hours[i+1]; total_hours[i+1] = temp; } } }
}
void Prog8_5() { //Declare the three matrix double a[3][N], b[3][N], c[3][N];
//prompt user to enter the value in the first matrix cout << "Enter the first 3 by 3 matrix row by row: " << endl;
//for loop is used to takes an input for (int i = 0; i < N; i++) { //loop to access column of matrix for (int j = 0; j < N; j++) { cin >> a[i][j]; } } //prompt user to enter the value in the first matrix cout << "Enter the second 3 by 3 matrix row by row: " << endl; //nested for loop is used to take an input for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { cin >> b[i][j]; } } //call the method addMatrix cout << addMatrix(a,b,c) << endl; }
void addMatrix(const double a[][N], const double b[][N], double c[][N]) { //for loop is used to add and store in the third matrix for (int i = 0; i < N; i++) { //loop to access column of matrix for (int j = 0; j < N; j++) { //adding elements of a and b matrix c[i][j] = a[i][j] + b[i][j]; } } cout << "Sum of these two matrix is given by " << endl; //display the third matrix for (int i = 0; i < N; i++) { //loop to access column of matrix for (int j = 0; j < N; j++) { cout << c[i][j] << "\t"; } cout << endl; } }
void Prog8_9() { //declare three double type array double a[N][N]; double b[N][N]; double c[N][N];
cout << "Enter matrix1: ";
//use nested for loop to take input for fist matrix from user for (int i = 0; i < N; i++) { //use for loop to access column for (int j = 0; j < N; j++) { cin >> a[i][j]; } } //use nested for loop to take input for second matrix from user cout << "Enter matrix2: "; for (int i = 0; i < N; i++) { //display for loop to access column for (int j = 0; j < N; j++) { cin >> b[i][j]; } } //call function to multiply the two matrix multiplyMatrix(a,b,c); }
void multiplyMatrix(const double a[][N], const double b[][N], const double c[][N]) { for (int i = 0; i < N; i++) { //use for loop to traverse column for (int j = 0; j < N; j++) { //multiply two matrix to get element of third // matrix c[i][j] = a[i][1] * b[1][j] + a[i][2] * b[2][j] + a[i][3] * b[3][j]; cout << "The multiplication of the matrices is " << endl; } } for (int i = 0; i < N; i++) { //display third row of first matrix for (int j = 0; j < N; j++) { //use setw() function to fix 5 character space for // 1 element cout << setw(5) << a[i][j]; } if (i == 1) cout << " = "; else cout << " "; //display third row of third matrix for (int j = 0; j < N; j++) { //use setw() function to fix 7 character space for // 1 element cout << setw(7) << c[i][j]; } cout << " "; }
}
void prog8_11() {
}
void prog8_17() { double a[3][4] = {0}; cout << "Enter the array (3x4): "; for (int r = 0; r < 3; r++) for (int c = 0; c < 4; c++) cin >> m[r][c]; int location[2] = {0}; locateLargest(m, location); cout << "The location of the largest element is at (" << location[0] << " , " << location[1] << " ) "; }
void locateLargest(const double a[][4], int location[]) { double largest = a[0][0]; //let largest be the first element from the 3X4 array for (int r = 0; r < 3; r++) for (int c = 0; c < 4; c++) { if (largest < a[r][c]) { largest = a[r][c]; location[0] = r; location[1] = c; } } }
void prog8_18() { double a11, a12, a13, a21, a22, a23, a31, a32, a33; cout << "Enter a11, a12, a13, a21, a22, a23, a31, a32, a33: "; cin >> a11 >> a12 >> a13>> a21 >> a22 >> a23 >> a31 >> a32>> a33; }
double determinantOfAMatrix(double A[][N]) { double result = A[0][0] * A[1][1] * A[2][2] + A[2][0] * A[0][1] * A[1][2] + A[0][2] * A[1][0] * A[2][1]-A[0][2] * A[2][0] - A[0][0] * A[1][2] * A[2][1] - A[2][2] * A[1][0] * A[0][1];
//return the value return result; }
void inverse(const double A[][3], double inverseOfA[][3]) { //invoke the function determinantOfAMatrix() to get the determinant value. double determinant = determinantOfAMatrix(A);
//obtain the inverse of the 3x3 matrix using the formula inverseOfA[0][0] = (A[1][1] * A[2][2] - A[1][2] * A[2][1]) / determinant; inverseOfA[0][1] = (A[0][2] * A[2][1] - A[0][1] * A[2][2]) / determinant; inverseOfA[0][2] = (A[0][1] * A[1][2] - A[0][2] * A[1][1]) / determinant; inverseOfA[1][0] = (A[1][2] * A[2][0] - A[1][0] * A[2][2]) / determinant; inverseOfA[1][1] = (A[0][0] * A[2][2] - A[0][2] * A[2][0]) / determinant; inverseOfA[1][2] = (A[0][2] * A[1][0] - A[0][0] * A[1][2]) / determinant; inverseOfA[2][0] = (A[1][0] * A[2][1] - A[1][1] * A[2][0]) / determinant; inverseOfA[2][1] = (A[0][1] * A[2][0] - A[0][0] * A[2][1]) / determinant; inverseOfA[2][2] = (A[0][0] * A[1][1] - A[0][1] * A[1][0]) / determinant; }
void prog8_30() {
}
bool linearEquation(const double a[][SIZE], const double b[], double result[]) { double bottom; //calculate denominator of provided formula bottom = (a[0][0] * a[1][1] - a[0][1] * a[1][0]); //check denominator is 0 if (bottom == 0) return false; else { //calcuate x and y and store then in the result array result [0] = (b[0] * a[1][1] - b[1] *a[0][1] / bottom); result [1] = (b[1] * a[0][0] - b[0] * a[1][0] / bottom); //display x and on screen cout << "X is " << result[0] << " and Y is " << result[1] << endl; return true; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
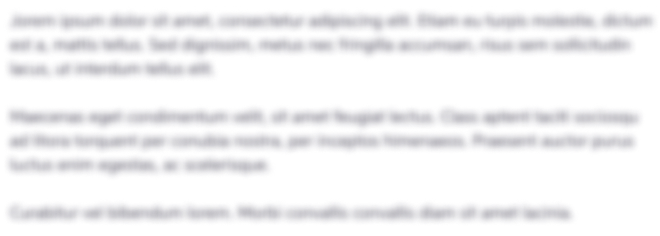
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started