Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I want this all answer Some for working with color. You do not need to understand how this functions work (yet). Author: Walker M.
I want this all answer
""" Some for working with color. You do not need to understand how this functions work (yet). Author: Walker M. White Data: August 9, 2019 """ import introcs def blend(color1,color2): """ Returns a new color that is the alpha blend of color1 over color2. This function assumes that the alpha values in the colors are not pre-multiplied. Parameter color1: The color on top Precondition: color1 is an RGB object Parameter color2: The color underneath Precondition: color2 is an RGB object """ def blendUnder(color1,color2): """ Modifies color1 by alpha-blending it underneath color2. This function assumes that the alpha values in the colors are not pre-multiplied. Parameter color1: The color to modify Precondition: color1 is an RGB object Parameter color2: The color to blend on top of color1 Precondition: color2 is an RGB object """ gl1 = color2.glColor() gl2 = color1.glColor() gl3 = [0,0,0,0] alpha = gl1[3]+gl2[3]*(1-gl1[3]) for pos in range(3): gl3[pos] = (gl1[pos]*gl1[3]+gl2[pos]*gl2[3]*(1-gl1[3]))/alpha gl3[pos] = round(gl3[pos]*255) gl3[3] = round(alpha*255) color1.red = gl3[0] color1.green = gl3[1] color1.blue = gl3[2] color1.alpha = gl3[3]
""" A script to show off the creation and use of objects. """ import introcs import funcs
""" Module that provides a simple Time class This class illustrates the concept of invariants, Invariants limit what values can be assigned to the attributes of an object. Author: Walker M. White (wmw2) Date: September 21, 2018 """ class Time(object): """ An instance represents a unit of time. Attributes: hours: Time in hours [int, must be nonnegative] minutes: Time in minutes [int in the rage 0..59] """ # Properties @property def hours(self): """ The number of hours in this time. **Invariant**: Value must be a positive int. """ return self._hours @hours.setter def hours(self, value): assert (type(value) == int), "value %s is not an int" % repr(value) assert (value >= 0), "value %s is not nonnegative" % repr(value) self._hours = value @property def minutes(self): """ The number of minutes in this time. **Invariant**: Value must be an int between 0 and 59, inclusive. """ return self._minutes @minutes.setter def minutes(self, value): assert (type(value) == int), "value %s is not an int" % repr(value) assert (value >= 0 and value <= 59), "value %s is outside of range [0,59]" % repr(value) self._minutes = value # Initializer def __init__(self,hours,minutes): """ **Constructor**: creates a new Time object with the given hours, minutes. Parameter hours: The number of hours Precondition: hours is a nonnegative int. Parameter minutes: The number of minutees Precondition: minutes is an int between 0 and 59, inclusive. """ self.hours = hours self.minutes = minutes def __eq__(self, other): """ Returns True if self and other are equivalent Time objects. Parameter other: The value to compare """ return (type(other) == Time and self.hours == other.hours and self.minutes == other.minutes) def __ne__(self, other): """ Returns True if self and other are not equivalent Time objects. Parameter other: The value to compare """ return not (self == other) def __str__(self): """ Returns a readable string representation of this time. """ min = str(self.minutes) if self.minutes < 10: min = '0'+min return str(self.hours)+":"+min def __repr__(self): """ Returns an unambiguous String representation of this time. """ return "%s(%s)" % (self.__class__,self.__str__())
""" Module demonstrating how to write functions with objects. This module contains two versions of the same function. One version returns a new value, while other modifies one of the arguments to contain the new value. """ import clock def add_time1(time1, time2): """ Returns the sum of time1 and time2 as a new Time object DO NOT ALTER time1 or time2, even though they are mutable Examples: The sum of 12hr 13min and 13hr 12min is 25hr 25min The sum of 1hr 59min and 3hr 2min is 5hr 1min Parameter time1: the starting time Precondition: time1 is a Time object Parameter time2: the time to add Precondition: time2 is a Time object """ def add_time2(time1, time2): """ Modifies time1 to be the sum of time1 and time2 DO NOT RETURN a new time object. Modify the object time1 instead. Examples: The sum of 12hr 13min and 13hr 12min is 25hr 25min The sum of 1hr 59min and 3hr 2min is 5hr 1min Parameter time1: the starting time Precondition: time1 is a Time object Parameter time2: the time to add Precondition: time2 is a Time object """
""" A completed test script for the time functions. Notice how complicated testing is now. To test that the return value of a function is correct, we need to test (1) its type and (2) each attribute separately. Because functions can now modify the arguments, we also need to verify that arguments are not modified unless the specification specifically says they are. Author: Walker M. White Date: August 9, 2019 """ import funcs import clock import introcs def test_add_time1(): """ Test procedure for the function add_time1() """ print('Testing add_time1()') # TEST 1: Sum 12hr 13min and 13hr 12min time1 = clock.Time(12,13) time2 = clock.Time(13,12) result = funcs.add_time1(time1,time2) introcs.assert_equals(clock.Time,type(result)) introcs.assert_equals(25,result.hours) introcs.assert_equals(25,result.minutes) # Verify no objects were modified introcs.assert_equals(12,time1.hours) introcs.assert_equals(13,time1.minutes) introcs.assert_equals(13,time2.hours) introcs.assert_equals(12,time2.minutes) # TEST 2: Sum 1hr 59min and 1hr 2min time1 = clock.Time(1,59) time2 = clock.Time(3,2) result = funcs.add_time1(time1,time2) introcs.assert_equals(clock.Time,type(result)) introcs.assert_equals(5,result.hours) introcs.assert_equals(1,result.minutes) # Verify no objects were modified introcs.assert_equals(1,time1.hours) introcs.assert_equals(59,time1.minutes) introcs.assert_equals(3,time2.hours) introcs.assert_equals(2,time2.minutes) # TEST 3: Sum 1hr 15min and 0hr 0min time1 = clock.Time(1,15) time2 = clock.Time(0,0) result = funcs.add_time1(time1,time2) introcs.assert_equals(clock.Time,type(result)) introcs.assert_equals(1,result.hours) introcs.assert_equals(15,result.minutes) # Verify no objects were modified introcs.assert_equals(1,time1.hours) introcs.assert_equals(15,time1.minutes) introcs.assert_equals(0,time2.hours) introcs.assert_equals(0,time2.minutes) # Feel free to add more def test_add_time2(): """ Test procedure for the function add_time2() """ print('Testing add_time2()') # TEST 1: Sum 12hr 13min and 13hr 12min time1 = clock.Time(12,13) time2 = clock.Time(13,12) # Verify that nothing is returned result = funcs.add_time2(time1,time2) introcs.assert_equals(None,result) # Verify time1 is changed, but time2 is not introcs.assert_equals(25,time1.hours) introcs.assert_equals(25,time1.minutes) introcs.assert_equals(13,time2.hours) introcs.assert_equals(12,time2.minutes) # TEST 2: Sum 1hr 59min and 1hr 2min time1 = clock.Time(1,59) time2 = clock.Time(3,2) # Verify that nothing is returned result = funcs.add_time2(time1,time2) introcs.assert_equals(None,result) # Verify time1 is changed, but time2 is not introcs.assert_equals(5,time1.hours) introcs.assert_equals(1,time1.minutes) introcs.assert_equals(3,time2.hours) introcs.assert_equals(2,time2.minutes) # TEST 3: Sum 1hr 15min and 0hr 0min time1 = clock.Time(1,15) time2 = clock.Time(0,0) # Verify that nothing is returned result = funcs.add_time2(time1,time2) introcs.assert_equals(None,result) # Verify that objects are correct introcs.assert_equals(1,time1.hours) introcs.assert_equals(15,time1.minutes) introcs.assert_equals(0,time2.hours) introcs.assert_equals(0,time2.minutes) # Feel free to add more if __name__ == '__main__': test_add_time1() test_add_time2() print('Module funcs passed all tests.')
""" Functions demonstrating string methods. Neither this module nor any of these functions should import the introcs module. In addition, you are not allowed to use loops or recursion in either function. """ def first_in_parens(s): """ Returns: The substring of s that is inside the first pair of parentheses. The first pair of parenthesis consist of the first instance of character '(' and the first instance of ')' that follows it. Examples: first_in_parens('A (B) C') returns 'B' first_in_parens('A (B) (C)') returns 'B' first_in_parens('A ((B) (C))') returns '(B' Parameter s: a string to check Precondition: s is a string with a matching pair of parens '()'. """ def isnetid(s): """ Returns True if s is a valid Cornell netid. Cornell network ids consist of 2 or 3 lower-case initials followed by a sequence of digits. Examples: isnetid('wmw2') returns True isnetid('2wmw') returns False isnetid('ww2345') returns True isnetid('w2345') returns False isnetid('WW345') returns False Parameter s: the string to check Precondition: s is a string """
""" Functions demonstrating string methods. Neither this module nor any of these functions should import the introcs module. In addition, you are not allowed to use loops or recursion in either function. """ def first_in_parens(s): """ Returns: The substring of s that is inside the first pair of parentheses. The first pair of parenthesis consist of the first instance of character '(' and the first instance of ')' that follows it. Examples: first_in_parens('A (B) C') returns 'B' first_in_parens('A (B) (C)') returns 'B' first_in_parens('A ((B) (C))') returns '(B' Parameter s: a string to check Precondition: s is a string with a matching pair of parens '()'. """ def isnetid(s): """ Returns True if s is a valid Cornell netid. Cornell network ids consist of 2 or 3 lower-case initials followed by a sequence of digits. Examples: isnetid('wmw2') returns True isnetid('2wmw') returns False isnetid('ww2345') returns True isnetid('w2345') returns False isnetid('WW345') returns False Parameter s: the string to check Precondition: s is a string """
""" A completed test script for the string functions. """ import introcs import funcs def test_first_in_parens(): """ Test procedure for first_in_parens """ print('Testing first_in_parens()') result = funcs.first_in_parens('A (B) C') introcs.assert_equals('B',result) result = funcs.first_in_parens('A (B) (C) D') introcs.assert_equals('B',result) result = funcs.first_in_parens('A (B (C) D) E') introcs.assert_equals('B (C',result) result = funcs.first_in_parens('A ) B (C) D') introcs.assert_equals('C',result) result = funcs.first_in_parens('A () D') introcs.assert_equals('',result) result = funcs.first_in_parens('(A D)') introcs.assert_equals('A D',result) def test_isnetid(): """ Test procedure for isnetid """ print('Testing isnetid()') result = funcs.isnetid('wmw2') introcs.assert_true(result) result = funcs.isnetid('jrs1234') introcs.assert_true(result) result = funcs.isnetid('ww9999') introcs.assert_true(result) result = funcs.isnetid('Wmw2') introcs.assert_false(result) result = funcs.isnetid('wMw2') introcs.assert_false(result) result = funcs.isnetid('wmW2') introcs.assert_false(result) result = funcs.isnetid('ww99a99') introcs.assert_false(result) result = funcs.isnetid('#w999') introcs.assert_false(result) result = funcs.isnetid('w#w999') introcs.assert_false(result) result = funcs.isnetid('ww#999') introcs.assert_false(result) # Script code if __name__ == '__main__': test_first_in_parens() test_isnetid() print('Module funcs is working correctly')
""" Module to show off tuple methods. Neither this module nor the function should import the introcs module. In addition, the function should not use a loop or recursion. """ def replace_first(tup,a,b): """ Returns a copy of tup with the first value of a replaced by b Examples: replace_first((1,2,1),1,3) returns (3,2,1) replace_first((1,2,1),4,3) returns (1,2,1) Parameter tup: The tuple to copy Precondition: tup is a tuple of integers Parameter a: The value to replace Precondition: a is an int Parameter b: The value to replace with Precondition: b is an int
""" A completed test script for the function replace_first Author: Walker M. White Date: August 9, 2019 """ import introcs import func def test_replace_first(): """ Test procedure for replace_first """ print('Testing replace_first()') result = func.replace_first((1,2,3),1,4) introcs.assert_equals((4,2,3),result) result = func.replace_first((1,2,3),2,4) introcs.assert_equals((1,4,3),result) result = func.replace_first((1,2,3),3,4) introcs.assert_equals((1,2,4),result) result = func.replace_first((1,2,3),5,4) introcs.assert_equals((1,2,3),result) result = func.replace_first((1,2,1),1,4) introcs.assert_equals((4,2,1),result) result = func.replace_first((1,2,1,2),2,4) introcs.assert_equals((1,4,1,2),result) result = func.replace_first((2,),2,4) introcs.assert_equals((4,),result) result = func.replace_first((),2,4) introcs.assert_equals((),result) # Script code if __name__ == '__main__': test_replace_first() print('Module func is working correctly')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
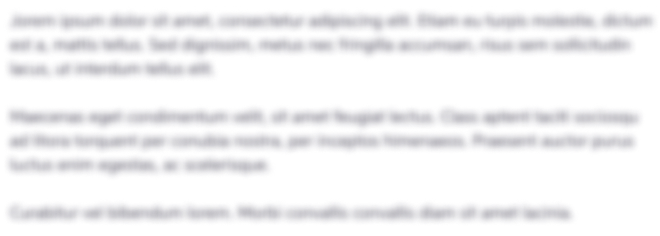
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started