Question
I want to convert my id type from int to cstring so it can take values like b001,b002 etc... however i am having trouble as
I want to convert my id type from int to cstring so it can take values like b001,b002 etc...
however i am having trouble as it only reads one character
i have to use file io
this is my code pls correct me where i am wrong
#include
int menu(); void display(int* studId, char* sec, double* grade, int numstud); void readFile(ifstream& infile, int* studid, char* sec, double* grade); int* resizeIntArr(int newelem, int* originalArray, int sizeoriginal, int& sizenew); double* resizeDoubleArr(double newelem, double* originalArray, int sizeoriginal, int& sizenew); char* resizeCharArr(char newelem, char* originalArray, int sizeoriginal, int& sizenew); void save(int* studId, char* sec, double* grade, int numstud); int search(int* studId, int starget, int numstud);
double* resizeDoubleArr(double newelem, double* originalArray, int sizeoriginal, int& sizenew) { sizenew = sizeoriginal + newelem; double* newarray = new double[sizenew]; for (int i = 0; i < sizeoriginal; i++) { newarray[i] = originalArray[i]; } newarray[sizeoriginal] = newelem;
return newarray; delete[]newarray; } int* resizeIntArr(int newelem, int* originalArray, int sizeoriginal, int& sizenew) { sizenew = sizeoriginal + newelem; int* newarray = new int[sizenew]; for (int i = 0; i < sizeoriginal; i++) { newarray[i] = originalArray[i]; } newarray[sizeoriginal] = newelem;
return newarray; delete[]newarray; } char* resizeCharArr(char newelem, char* originalArray, int sizeoriginal, int& sizenew) { sizenew = sizeoriginal + newelem; char* newarray = new char[sizenew]; for (int i = 0; i < sizeoriginal; i++) { newarray[i] = originalArray[i]; } newarray[sizeoriginal] = newelem;
return newarray; delete[]newarray; } int search(int* studId, int starget, int numstud) { for (int i = 0; i < numstud; i++) { if (studId[i] == starget) { return i; } } return -1; } void readFile(ifstream& infile, int* studid, char* sec, double* grade) {
int s = 0; while (!infile.eof())
{
infile >> studid[s]; infile >> sec[s]; infile >> grade[s];
s++;
} } void display(int* studId, char* sec, double* grade, int numstud) {
for (int i = 0; i < numstud; i++)
{
cout << "Student Id: " << studId[i] << "\t" << "Section: " << sec[i] << "\t" << "Grade: " << grade[i] << endl;
} } void save(int* studId, char* sec, double* grade, int numstud) {
ofstream outfile("data.txt");
outfile << numstud << endl;
for (int i = 0; i < numstud; i++)
{
outfile << studId[i] << " " << sec[i] << " " << grade[i] << endl;
}
outfile.close(); } int menu() {
int option;
cout << "1. Enter new student 2. View all students 3. Search for an Id 4. Save and Exit Enter option: ";
cin >> option;
return option; } int main() {
//open the file
//read from the file the number of students
//create the arrays (stud id, grades)
//read the student ids and grades
//close the file
int numstud;
ifstream infile("data.txt");
if (infile.fail())
{
cout << "Failed..." << endl;
exit(1);
}
infile >> numstud;
int* studId = new int[numstud]; char* sec = new char[numstud]; double* grade = new double[numstud];
readFile(infile, studId, sec, grade);
infile.close();
int option, newstud, index;
int newsize = numstud;
do {
option = menu();
switch (option)
{
case 1:
cout << "How many students: ";
cin >> newstud;
newsize = numstud + newstud;
studId = resizeIntArr(newstud, studId, numstud, newsize); sec = resizeCharArr(newstud, sec, numstud, newsize); grade = resizeDoubleArr(newstud, grade, numstud, newsize);
for (int i = numstud; i < newsize; i++)
{
cout << "Enter Id: ";
cin >> studId[i];
cout << "Enter Section: ";
cin >> sec[i];
cout << "Enter the grade: ";
cin >> grade[i];
}
numstud = newsize;
cout << endl; //complete the logic
break;
case 2:
display(studId, sec, grade, newsize); cout << endl; break;
case 3:
int target; cout << "Enter the Id number to search: "; cin >> target;
index = search(studId, target, newsize); if (index != -1) { cout << "Row: " << index + 1 << " " << "Id: " << studId[index] << " " << "Section: " << sec[index] << " " << "Grade: " << grade[index] << endl; } else { cout << "Id number not found" << endl; } cout << endl; break;
case 4:
save(studId, sec, grade, newsize);
break;
default:
cout << "invalid input" << endl;
}
} while (option != 4);
delete[]studId; delete[]sec; delete[]grade;
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
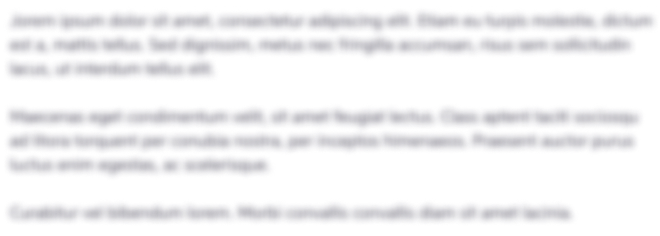
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started