Question
I want to know how to complete the given Java script (attached at the end) to meet the requirements, here is the guideline: In this
I want to know how to complete the given Java script (attached at the end) to meet the requirements, here is the guideline:
In this task, you will make a dim sum ordering system for the "G/F restaurant". The restaurant only has four different dishes of dim sum, as shown below:
- Barbecued pork bun ($25)
- Shrimp dumpling ($38)
- Siu mai ($30)
- Spring roll ($25)
First, you need to add the attributes of theDimSumclass and then finish the methods by putting appropriate content there.
Adding the Attributes
There are three attributes we want to add to the class:
- name
- price
- quantity
Thenameattribute stores the name of the dim sum dish. It will be used when the dim sum is displayed in the ordering program. Thepriceattribute stores the price of one dish of the dim sum. Finally, thequantityattribute is the quantity of the dim sum dishes that has been ordered so far. You need to create the attributes in the appropriate place in the class using appropriate data types. You can assume thepriceattribute is a float and thequantityattribute is an integer.
After adding the attributes you need to initialize their values in the constructor of the class. Looking at theDimSumclass, the constructor has two input parameters callednameandprice. That implies when you create- an instance of theDimSumclass, you need to provide the name of the dim sum and its price as input parameters. For example, if you want to create- the dim sum 'Siu Mai' you will use the following code:
DimSum siumai = new DimSum("Siu Mai", 30f);
The above example creates an instance of theDimSumclass and the dimsum object stores the name as"Siu Mai"and its price as 30. For the class, you need to store the input parametersnameandpriceto thenameattribute and thepriceattribute. You may find that you need to usethis.nameandthis.pricewhen you assign the values to the attributes, for example like this:
this.name = ...;
this.price = ...;
After that you also need to initialize thequantityattribute to become 0.
Finishing getName(), getPrice() and getQuantity()
Three methods in the class,getName(),getPrice()andgetQuantity(), have been created so that other code can retrieve the current values of the three attributes respectively. You will need to return the corresponding attribute for each of the methods, rather than a fixed value that is returned by the starting class.
Finishing order()
The program stores the quantity that each dim sum dish has been ordered so far. When one of the dim sum dishes has been ordered, the program will use theordermethod to increase the ordered quantity of that dim sum dish. Therefore, the use oforder()is to increase thequantityattribute of the class.
Testing Your Class
Now theDimSumclass has been finished. You can test it using the object bench. For example you can create- a new instance ofDimSumin BlueJ like this:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
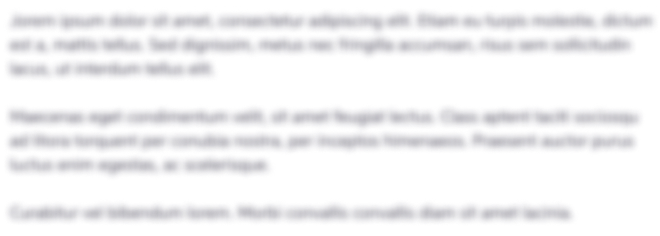
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started