Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I want to solve these questions in java. I have attached a code that I want to solve by modifying this code import javax.swing.JOptionPane; //Main
I want to solve these questions in java. I have attached a code that I want to solve by modifying this code
import javax.swing.JOptionPane; //Main Class public class MainClass { public static void main(String[] args) { // Creating object myPlatform of Platform Platform myPlatform = new Platform(); String input = ""; // variable to store input from user while (!input.equals("5")) { // check if input is not 5 (Exit) // Showing prompt to enter input input = JOptionPane.showInputDialog( "What do you want to do? 1.Add show 2.Search shows 3.Print movies 4.Print Series 5.Exit"); // switch case to perform operation based on input switch (input) { case "1": myPlatform.addShow(); // calling method to add show break; case "2": myPlatform.searchShows(); // calling method to search shows break; case "3": myPlatform.printMovies(); // calling method to print movies break; case "4": myPlatform.printSeries(); // calling method to print series break; case "5": // Program will exit break; default: // For all other options wrong input message is showed JOptionPane.showMessageDialog(null, "Wrong input"); } } } }
Movie.java
import javax.swing.JOptionPane; //Class Movie inheriting Show class public class Movie extends Show { // Attributes of class private double duration; private int productionYear; // no-args constructor of class public Movie() { // Getting input from user using JOptionPane // Getting duration as input and parsing it to double duration = Double.parseDouble(JOptionPane.showInputDialog("Enter movie duration: ")); // Getting productionYear as input and parsing it to integer productionYear = Integer.parseInt(JOptionPane.showInputDialog("Enter production year: ")); } // parameterized constructor public Movie(String name, double duration, int productionYear) { super(name); this.duration = duration; this.productionYear = productionYear; } // Getter method of Duration public double getDuration() { return duration; } // Getter method of production year public int getProductionYear() { return productionYear; } // toString method of the class public String toString() { return super.toString() + " " + duration + " - " + productionYear; } // Overriding equals method @Override public boolean equals(Object obj) { // calling parent equals method, checking production year are eqaul return super.equals(obj) && (productionYear == ((Movie) obj).getProductionYear()); } }
Platform.java
import java.util.ArrayList; import javax.swing.JOptionPane; //Class Platform public class Platform { // ArrayList to store shows private ArrayListshows; // no-args constructor public Platform() { // initializing shows to empty arraylist shows = new ArrayList(); } // Method to add shows public void addShow() { // Getting input from user String option = JOptionPane.showInputDialog("What type of show do you want to add? 1.Movie 2.Series"); switch (option) { case "1": shows.add(new Movie()); // adding new object of movie to arraylist break; case "2": shows.add(new Series()); // adding new object of series to arraylist break; default: JOptionPane.showMessageDialog(null, "Wrong Input"); // wrong input message for invalid input } } // Method to search shows public void searchShows() { // Getting showname from user String showName = JOptionPane.showInputDialog("Enter name of show you want to find: "); String output = "Could not find show with title " + showName; // initializing output message // Iteratiing over arraylist to find show with matching input for (Show show : shows) { if (show.name.equalsIgnoreCase(showName)) { // checking if name are equal output = show.toString(); // show is converted to string break; } } JOptionPane.showMessageDialog(null, output); // output is showed using message dialog } // Method to print movies public void printMovies() { String output = ""; // variable to keep output message for (Show show : shows) { // iterating over shows if (show instanceof Movie) { // checking if show is an instance of Movie output += show.toString() + " "; // storing string value of show to output variable } } JOptionPane.showMessageDialog(null, output);// output is showed using message dialog } public void printSeries() { String output = ""; // variable to keep output message for (Show show : shows) {// iterating over shows if (show instanceof Series) { // checking if show is an instance of Series output += show.toString() + " ";// storing string value of show to output variable } } JOptionPane.showMessageDialog(null, output); // output is showed using message dialog } }
Series.java
import javax.swing.JOptionPane; public class Series extends Show { private int numOfEpisodes; private double episodeDuration; // no-arg constructor public Series() { // Getting input from user using JOptionPane numOfEpisodes = Integer.parseInt(JOptionPane.showInputDialog("Enter number of episodes: ")); episodeDuration = Double.parseDouble(JOptionPane.showInputDialog("Enter average episode duration: ")); } // parameterized constructor public Series(String name, int numOfEpisodes, double episodeDuration) { super(name); this.numOfEpisodes = numOfEpisodes; this.episodeDuration = episodeDuration; } // Getter of numOfEpisodes public int getNumOfEpisodes() { return numOfEpisodes; } // Getter of episodeDuration public double getEpisodeDuration() { return episodeDuration; } // toString method to print series as string @Override public String toString() { return super.toString() + " " + numOfEpisodes + " - " + episodeDuration; } }
Show.java
import java.util.Date; import javax.swing.JOptionPane; //Class Show public class Show { // Attributes of class public String name; private int timesWatched; private double rate; private static int maxRate = 5; private Date dateAdded; // Date variable // no-args constructor public Show() { // Getting input from user using JOptionPane name = JOptionPane.showInputDialog("Enter show name: "); timesWatched = Integer.parseInt(JOptionPane.showInputDialog("Enter number of times watched: ")); rate = Double.parseDouble(JOptionPane.showInputDialog("Enter rate: ")); dateAdded = new Date(); // dateAdded is initialized using default Date constructor } // parameterized constructor public Show(String name) { this.name = name; } // Getter of rate public double getRate() { return rate; } // Getter of timesWatched public double getTimesWatched() { return timesWatched; } // Getter of maxRate public static int getMaxRate() { return maxRate; } // Getter of dateAdded public Date getDateAdded() { return dateAdded; } // Method to updateRate public void updateRate() { // Getting input from user using JOptionPane double userRate = Double .parseDouble(JOptionPane.showInputDialog("Enter you rate from 0 to " + maxRate + " for " + name)); double sum = rate * timesWatched + userRate; timesWatched++; rate = sum / timesWatched; } // Overriding toString method @Override public String toString() { // dateAdded is also printed using toString method return name + " - " + rate + " - " + timesWatched + " - " + dateAdded.toString(); } // Overriding equals method @Override public boolean equals(Object obj) { return rate == ((Show) obj).getRate(); // checking if rate is equal } }Lab Objectives: Apply the concept of abstract classes and methods. Define and implement interfaces. Use methods of the String class to perform operations on Strings. * Use the classes Show, Movie, Series, and Platform that you implemented in Labs, and perform the following modifications which are shown in this UML diagram: > Ratable + updateRate():double > java.lang. Comparable
Step by Step Solution
There are 3 Steps involved in it
Step: 1
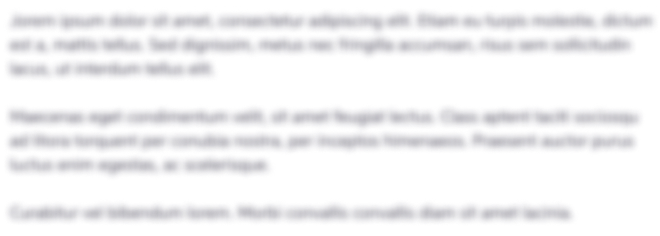
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started