Question
I was given this answer: Vacation.java //create the abstract class vacation abstract class vacation { // declare the variable destination as string private String destination;
I was given this answer:
Vacation.java
//create the abstract class "vacation"
abstract class vacation {
// declare the variable "destination" as string
private String destination;
// create the constructor
public vacation(String destination) {
this.destination = destination;
}
// create the "toString()"
public String toString() {
// return the "destination"
return " The Destination is:" + destination;
}
// call the abstract method of "calculatecost"
public abstract double calculatecost();
}
// create the "allinclusive" class extend from the "vacation" class
class allinclusive extends vacation {
// declare the necessary data types
private String brand;
private int rating;
private double price;
// create the constructor of "allinclusive"
public allinclusive(String brand, int rating, double price, String destination) {
super(destination);
this.brand = brand;
this.rating = rating;
this.price = price;
}
// create the "toString()"
public String toString() {
// return the "brand","rating","price"
return super.toString() + " Brand : " + brand + " " + " Rating : " + rating + " " + " Price : " + price;
}
// return the "price" value
public double calculatecost() {
return price;
}
}
// create the "piecemeal" class extend from the "vacation" class
class piecemeal extends vacation {
// declare the necessary data types
private double hotel;
private double meal;
private double airfare;
// create the constructor of "piecemeal"
public piecemeal(double hotel, double meal, double airfare, String destination) {
super(destination);
this.hotel = hotel;
this.meal = meal;
this.airfare = airfare;
}
// create the "toString()"
public String toString() {
// return the "hotelcost","mealcost","airfarecost"
return super.toString() + " Hotel Cost: " + hotel + " " + " Meal Cost : " + meal + " " + " Airfare Cost : "
+ airfare;
}
// return the "calculatecost()"
public double calculatecost() {
return (hotel + meal + airfare);
}
}
VacationClient.java
//create the main method
public class VacationClient {
public static void main(String[] args) {
// create the array list of "vacation"
List
// assign the value to "allinclusive"
allinclusive x = new allinclusive("Good", 5, 679, "Jamaica");
// assign the value to "piecemeal"
piecemeal y = new piecemeal(852.43, 64.8, 56.9, "Secrets St. James Montego Bay");
// add the vlaue to the list
l.add(x);
l.add(y);
// display the output
for (int i = 0; i
System.out.println(l.get(i).toString());
System.out.println(" Cost : $" + l.get(i).calculatecost());
}
}
}
Output
The Destination is:Jamaica Brand : Good Rating : 5 Price : 679.0 Cost : $679.0
The Destination is:Secrets St. James Montego Bay Hotel Cost: 852.43 Meal Cost : 64.8 Airfare Cost : 56.9 Cost : $974.1299999999999 but i get the following error:
how can i fix it?
Write an abstract super class encapsulating a vacation: A vacation has one attribute: a destination. It has an overloaded constructon a toString) and an abstract calculate cost method that returns the total cost of each vacation. This class has two non-abstract subclasses: one encapsulating an all-inclusive vacation, and the other encapsulating a vacation bought piece-meal An all-inclusive vacation has three additional attributes: a brand (for instance ClubMed); a rating, expressed as a number of stars; and a price. In addition an all-inclusive vacation has an overloaded constructor, a toString) and a concrete calculate cost method that returns the total cost of the all-inclusive vacation vacation (price) A piecemeal vacation has three additional attributes: hotel, meal airfare as corresponding costs. In addition an all-inclusive vacation has an overloaded constructor, a toString0 and a concrete calculate cost method that returns the total cost of the all-inclusive ion ( Sum of hotel, meal and airfare) vacat You also need to include a client class to test these two classes using either an AirayList or an airay of objects to populate the array. Print the state of each element and the total costs for each vacation elementStep by Step Solution
There are 3 Steps involved in it
Step: 1
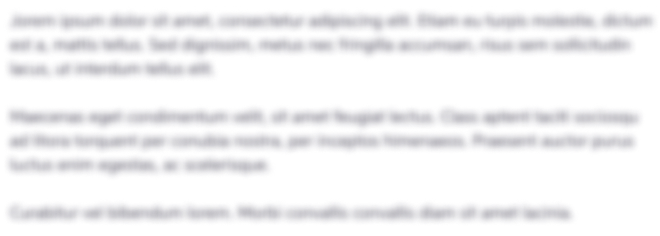
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started