Question
I was wondering if I could get some help with the assignall method. All the other classes pass their tests and I believe most of
I was wondering if I could get some help with the assignall method. All the other classes pass their tests and I believe most of the code in the scheduler class is correct. I'm just not too sure how to implement that one method.
Student.java
package scheduler;
import java.util.ArrayList; import java.util.List;
/** * A class representing a student. * * @author liberato * */ public class Student { /** * * Instantiates a new Student object. The student's maximum course load must be greater * than zero, and the preferences list must contain at least one course. * * The preference list is copied into this Student object. * * @param name the student's name * @param maxCourses the maximum number of courses that can be on this student's schedule * @param preferences the student's ordered list of preferred courses * @throws IllegalArgumentException thrown if the maxCourses or preferences are invalid */ private String name; private int maxCourses; private List
Course.java
package scheduler;
import java.util.ArrayList; import java.util.List;
/** * A class representing a Course. * * @author liberato * */ public class Course { /** * Instantiates a new Course object. The course number must be non-empty, and the * capacity must be greater than zero. * @param courseNumber a course number, like "COMPSCI190D" * @param capacity the maximum number of students that can be in the class * @throws IllegalArgumentException thrown if the courseNumber or capacity are invalid */ private String courseNumber; private int capacity; private List
/** * Returns the list of students enrolled in the course. * * This returned object does not share state with the internal state of the Course. * * @return the list of students currently in the course */ public List
Scheduler.java
package scheduler;
import java.util.ArrayList; import java.util.List;
public class Scheduler { /** * Instantiates a new, empty scheduler. */ private List
/** * Drops a student from a course. * * @param student * @param course * @throws IllegalArgumentException if either the student or the course are not known to this scheduler */ public void drop(Student student, Course course) throws IllegalArgumentException { course.getRoster().remove(student); student.getSchedule().remove(course); if(students.contains(student) == false) { throw new IllegalArgumentException("Student is not in the scheduler"); } if(classes.contains(course) == false) { throw new IllegalArgumentException("This course is not in the scheduler"); } } /** * Drops a student from all of their courses. * * @param student * @throws IllegalArgumentException if the student is not known to this scheduler */ public void unenroll(Student student) throws IllegalArgumentException{ if(students.contains(student) == false) { throw new IllegalArgumentException("Student is not in the scheduler"); } for(int i = 0; i < classes.size(); i++) { classes.remove(classes.get(i)); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
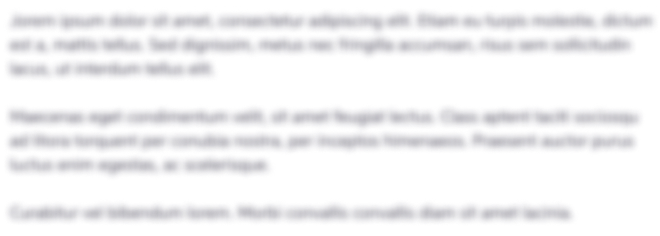
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started