Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I would like to build a code that displays the pressed button when a button is pressed on a 4 x 4 keypad. When you
I would like to build a code that displays the pressed button when a button is pressed on a x keypad. When you run the code below, the vertical columns will output the same value. Press keypad to display ; press keypad to display Please teach me what is wrong and how to correct it
Template to help create the scan function. Refer to the scanning algorithm in lecturebook section
#include
#include
#include stmlxxh
#include "UART.h
#include "SysClock.h
#include "keypad.h Include keypad header file
Let us make configs in one function and declare in the header file. Much better, right?
void KeypadInit
UARTInit;
SystemClockInit;
Port C config. Go to the header file as shown in class. Check your header file for macros.
Enable GPIO clock
RCCAHBENR RCCAHBENRGPIOCEN;
Setting PC pins as GPIO output
GPIO Mode: Input Output AlterFunc Analog reset
GPIOCMODER & ~GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE;
GPIOCMODER GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE; Output
Setting PC pins as GPIO input
GPIO Mode: Input Output AlterFunc Analog reset
GPIOCMODER & ~GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE; Input
GPIO Output Type: Output pushpull reset Output open drain
GPIOCOTYPER & ~GPIOOTYPEROT GPIOOTYPEROT GPIOOTYPEROT GPIOOTYPEROT; Pushpull
unsigned char keypadscan
unsigned char row, col;
unsigned char key;
unsigned char keymap
A
B
C
#D
;
Defineinitialize two integers, for "input" and "output" masks assignments.
uintt inputmask GPIOIDRID GPIOIDRID GPIOIDRID GPIOIDRID; input mask
uintt outputmask GPIOODROD GPIOODROD GPIOODROD GPIOODROD; output mask
Masking outputs array
uintt outputArray GPIOODROD GPIOODROD GPIOODROD GPIOODROD;
Masking inputs array
uintt inputArray GPIOIDRID GPIOIDRID GPIOIDRID GPIOIDRID;
waitms; short delay debounce
Wait until key pressed
while GPIOCIDR & inputmask inputmask;
for row ; row ; row Row scan
Set all rows to Output
GPIOCMODER & ~GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE;
GPIOCMODER GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE GPIOMODERMODE; Output
Set current row to low
GPIOCODR & ~outputArrayrow;
waitms; short delay
for col ; col ; col Column scan
if GPIOCIDR & inputArraycol
Grab the chosen key
key keymaprowcol;
Wait until key released
while GPIOCIDR & inputArraycol;
return key; return the key pressed
return xFF; Nothing is going on No press of any key
Delay function
void waitmsunsigned int ms
int i j;
for i ; i ms; i
for j ; j ; j;
int main
KeypadInit; Initialize keypad
while
unsigned char key keypadscan; Scan keypad for key press
if key xFF
printfPressed key: cr
key; Print the pressed key
Step by Step Solution
There are 3 Steps involved in it
Step: 1
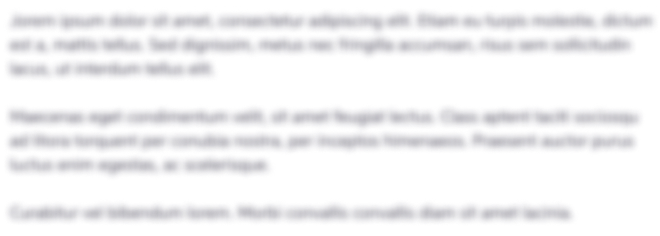
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started