Question
I would like to see this done properly thanks in advanced . Practice using loops. Problem 1: This will be a program that will use
I would like to see this done properly thanks in advanced .
Practice using loops.
Problem 1: This will be a program that will use loops to do computations.
Create a class called Computations. There should not be a constructor or any instance variables.
Create a method called computeEvenSums with an integer argument called stop. Inside the method create a local integer variable named sum and set it to 0. This should be outside the loop. Create a for loop that begins at 2 and ends at stop. It should be incremented by 2. Add the increment to sum. The first time through the increment should be 2 and the sum will be 2. The second time through the loop the increment will be 4 and the sum will be 6. End the loop. Return sum. Be careful and not test this with a large stop value.
Create a method called sumAllSquares with an integer argument called stop. Inside the method create a local integer variable named sum and set it to 0. Create an another integer variable named square and set it to 0. These variables should be outside the loop. Create a for loop that begins at 1 and ends at stop. It should be incremented by 1. Inside the loop, square the increment variable each time the loop is executed and assign it to square. For example, assuming you are using x for your increment the code should be square = x*x; Add square to sum. End the loop. Return sum. Be careful and not test this with a large stop value.
Create a method called computeOddSums with two integer arguments one called start and one called stop. Inside the method create a local integer variable named sum and set it to 0. This should be outside the loop. Create a for loop that begins at start and ends at stop. It should be incremented by 2. Add the increment to sum. The first time through the increment should be the start value and the sum will be the start value. The second time through the loop the increment will be the start value and 2. End the loop. Return sum. Be careful and not test this with a large stop value.
Create a method called calculateAllPowers with an integer argument called stop. Create a double variable named power and assign it a 0. Create a for loop that runs from 0 and ends at stop. Increment the loop by 1. Inside the loop, raise 2 to a power. Use the increment as the power. And assign it to power. For example, power = Math.pow(2,i); Print Two raised to the + i + power is: + power; End the loop. Do not return anything.
Create a tester class name ComputationTester.
Import Scanner
Create a main
Create a Scanner object.
Create a Computations object.
Ask the user to input the value to stop computing even numbers. Remind them to make the number stop at an even value. Call the receiving variable end.
Create an integer variable named sum and set it to 0.
Call the method sumAllEven and assign it to sum.
Print the result with word something like The sums of all even numbers from 0 to " + end + "is: " + sums + " "
Ask the user to input the value to stop computing the sum of the squares. Use the end variable.
Call the method sumOfSquares and assign it to sum.
Repeat n with the appropriate wording.
Ask the user to input the value to start computing all odd values. Create a begin variable to receive the value.
Ask the user to input the value to stop computing all odd values. Use the end variable.
Call the method computeOddSums and assign it to sum.
Repeat n with the appropriate wording.
Ask the user to input the value to stop computing the squares. Use the end variable.
Call the method calculateAllPowers.
Problem 2: In this problem, you will solve the same problem using all 3 loops.
Create a class called Loops with no constructor or instance variables.
The class will have 3 different method. The first method is a While loop method. Call it usingWhileLoops and it will have an integer argument called stop. Use a while loop to calculate the average of all numbers from 1 to 20. You will need three local variables which should be declared outside the loop. You will need an integer called begin which is set to 1. Next you will need a double called sum which is set to 0. Lastly you will need an integer, count, which you set to 0. I have specified the sum to be a double so that the result will be a double. You loop condition should be count
The second method will be a For loop method. Call it usingForLoops and it will have the same integer argument called stop. Remember that stop from the usingWhileLoops goes away at the end of the method so you will need a new stop value. This method should do the same thing as b. The code will be a little different. Remember the difference between a while and for loop.
The last method will be a do loop. Call it usingDoLoops and it will also have the same integer argument called stop. Again, the code will perform the same operation but using a Do loop instead of a for loop.
Create a LoopsTester class.
Import Scanner.
Create a Scanner object.
Create a Loops object.
Prompt the user to enter a stopping value. This will be used as input to each of the 3 methods.
Call the three methods and print the results.
Problem 3: Calculating Fibonacci numbers. The Fibonacci series is famous mathematical and computer science algorithm. For one thing it can describe the growth of a rabbit population.
Create a class named Fib
Create an instance variable named number
Create a constructor that has an argument which is an integer and hold the number your wish to calculate the Fibonacci series for.
Assign the argument to number.
Create a method named calcFib. It will not have an argument.
Create three integer local variables named fold1, fold2, and foldNew. Set foldNew to 0. Set fold1 and fold2 to 1.
Create a for loop that runs from 0 to less than number
Set for foldNew to fold1 plus fold2.
Set fold2 to fold1;
Set fold1 to foldNew;
At the end of the loop return foldNew
Create a class called FibTester (this should only have a main method)
Import Scanner
Create a Scanner object
Prompt the user for the number for which they want to calculate the Fibonnacci value.
Get and integer and assign it to num.
Create a Fib object using num as the argument.
Call calcFib and print the result. (This can be done in one or two steps)
Problem 4: This one you will do on your own. You will use the Bug class from Lab 4.
Copy the Bug class from Lab4. You do not need to make any alterations to it.
Create a tester class named BugTester
Create a main method
Create a Bug object with 10 as the argument
In a loop, breed the bugs 4 times.
In a loop, spray the bugs 4 times.
Print the result
Step by Step Solution
There are 3 Steps involved in it
Step: 1
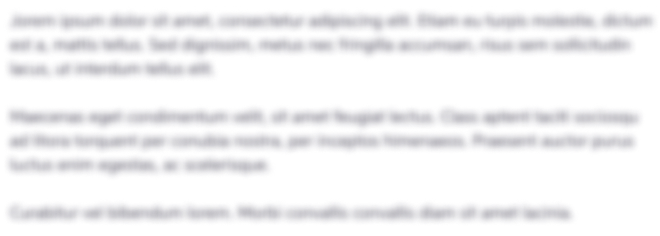
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started